Today’s digital world values Android developers. You must grasp the Android platform, architecture, and programming languages and tools to become an Android developer. Android app developers are in demand due to the rise of smartphones and mobile devices. Technical expertise, programming experience, and a passion for creating high-quality apps are needed. In this article, you will read complete detail about What is Android Development, How to Become and Android developer and If you are an android Developer you can check the Android Developer Interview Questions.
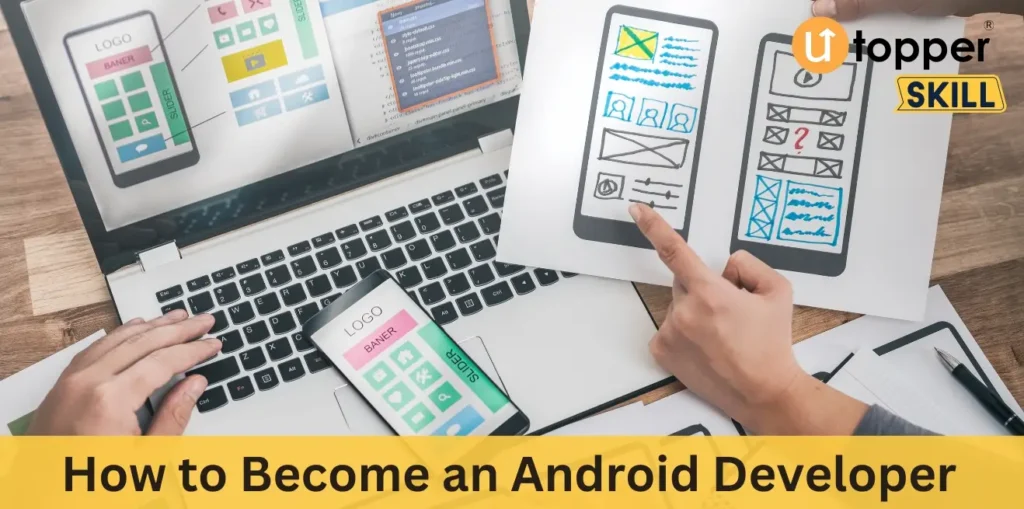
Definition of Android Development
Android Development is the process of Building apps for Android-powered smartphones, tablets, and wearables.
Android development comprises designing, creating, testing, and delivering apps. This requires Android Studio, Java, and the Android Software Development Kit (SDK). Developers can utilise the Android SDK’s frameworks, tools, and sample code to create apps that use Android’s touch-screen interface, camera, and internet connectivity. Android Development aims to produce apps that offer a smooth and intuitive user experience while maximizing Android’s capabilities.
Overview of the Android Operating System
Google’s Android mobile platform. It is a Linux-based touch-screen operating system for smartphones and tablets. Android has been one of the most popular mobile operating systems since 2008.
Importance of Android Development in today’s world
Due to the growing use of mobile devices and technology, Android development is crucial today. Android Development’s benefits include:
- Android has billions of users globally. This shows that Android apps are in high demand and that creating for Android opens up a huge market.
- Demand for Android developers is rising as mobile device use rises. Android developers get jobs and businesses may access a wide and increasing audience with mobile apps.
- Developers can edit Android’s source code because it’s open source. This simplifies Android development and encourages inventiveness.
- Customizability: Android’s great level of flexibility lets developers design creative apps that match customers’ needs.
Prerequisites for Becoming an Android Developer
1. Knowledge of Java / Kotlin
Java:
- Java is a general-purpose, object-oriented programming language.
- It was first released in 1995 by Sun Microsystems (now owned by Oracle).
- Java is used for developing a variety of applications, including desktop, web, mobile, and enterprise applications.
- Java code runs on the Java Virtual Machine (JVM), which provides a platform-independent environment for running Java code.
- Java has large, well-established libraries and frameworks, which make it easier to develop a wide range of applications.
- Java is widely used in large organizations and has a large community of developers and users.
Kotlin:
- Kotlin is a cross-platform, statically typed, general-purpose programming language.
- It was first released in 2011 by JetBrains.
- Kotlin is designed to be more concise and expressive than Java, while still being fully interoperable with Java.
- Kotlin is fully supported for Android development by Google.
- Kotlin has a growing ecosystem, with a number of libraries and tools available for various tasks.
- Kotlin has a growing community of developers and users, and is becoming increasingly popular for developing Android and server-side applications.
2. Understand the Concept of Oops
The key concepts in Object-Oriented Programming (OOP) are:
- Objects: Objects are instances of classes and represent real-world entities. Objects contain data and code that manipulate that data.
- Classes: A class is a blueprint for creating objects. It defines the data and code that make up an object.
- Inheritance: Inheritance is a mechanism where a new class can be created by inheriting properties and behavior from an existing class.
- Polymorphism: Polymorphism is the ability of an object to take on multiple forms. This allows objects of different classes to be used interchangeably, as long as they implement a common interface.
- Encapsulation: Encapsulation is the practice of hiding the implementation details of an object and exposing only the public interface. This helps to protect the data within an object from unintended modifications.
- Abstraction: Abstraction is the practice of providing a simplified interface to complex systems, hiding their underlying details. This makes it easier to work with complex systems, and reduces the likelihood of errors.
3. Familiar with XML Files
XML (Extensible Markup Language) is a markup language used to store and exchange data. XML files contain data in a structured format, using tags to define the structure and meaning of the data.
Here is an example of an XML file that describes a book:
<book>
<title>The Great Gatsby</title>
<author>F. Scott Fitzgerald</author>
<publisher>Charles Scribner's Sons</publisher>
<published_year>1925</published_year>
<price>9.99</price>
</book>
In Android, XML files are used to define various types of resources, such as layouts for activities, strings for localization, and styles for appearance.
Here is an example of an XML layout file for an activity:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, World!" />
</LinearLayout>
In this example, the root element is a LinearLayout
, which is a type of view group in Android that arranges its children in a single row or column. The android:layout_width
and android:layout_height
attributes define the size of the layout.
What is the Type of App You want to Build?
Hybrid / Native / Web App
What is a Hybrid App :
Hybrid mobile apps mix native and web-based features. It operates in a native container on mobile devices utilizing HTML, CSS, and JavaScript. This lets the app utilize native device functionality and reach a wider audience through numerous app stores.
Building a hybrid mobile app involves the following steps:
- Define the app requirements and goals
- Choose a development framework such as Ionic, React Native or Xamarin.
- Design the app’s user interface and user experience.
- Write the app’s code using HTML, CSS, and JavaScript.
- Test the app on multiple devices and platforms to ensure compatibility.
- Package the app for distribution through app stores such as the Apple App Store or Google Play Store.
- Publish the app to the app stores and make it available to users.
What is a Native App :
Native apps are mobile applications designed for a single operating system (OS) like iOS or Android utilising that OS’s programming languages and development tools. Native apps integrate with the device’s hardware and software and offer a native user experience.
Native apps employ the newest OS-level technology and have access to all of the device’s functionality. Hybrid or web-based programs don’t have this advantage. Designing and maintaining native apps for different platforms might take longer and cost more than building a hybrid or web-based app.
These steps will build a native app:
- App objective and features: Determine the app’s purpose and features.
- Platform: Build the app for iOS, Android, or both.
- Development environment: Choose Xcode for iOS or Android Studio for Android.
- Learn programming: Swift for iOS and Java / Kotlin for Android are supported programming languages.
- App interface design: Make your interface user-friendly and attractive.
- Code: Write app code in your chosen programming language and development environment.
- App test: To verify app functionality, test it on multiple platforms and devices.
- Submit the app to the app store for approval and release.
4. Read other Developer Code
Reading other developers’ code helps improve your coding skills. Here is the Code-reading tips:
- Before going into the code, try to understand its goal. Read the code’s documentation, and comments, and commit messages to understand it.
- Explain: Break the code into smaller, manageable bits. Focus on one function or module.
- Patterns: Find patterns in the code and figure out how it all fits together.
- Key ideas: Focus on data structures, algorithms, and design patterns in the code.
- Experiment: Make modest modifications to the code and run it. This can explain how the code works.
- Ask questions: Ask the author or other developers if you have coding questions.
- Repetition: Read as much code as possible and try to understand it.
- Reading code is about learning, therefore don’t be scared to ask questions or make mistakes.
5. Read Documentation
6. Start a Small Project and then Learn Slowly .
What are the topics You should read to Become an android developer?
Here is a list of topics that Android developers should familiarize themselves with:
- Android Activity Lifecycle
- Intents and Services
- User Interface (UI) Design
- Material Design
- Layouts and Views
- Multithreading and AsyncTask
- Android SQLite Database
- Fragments
- Android Networking
- Permissions and Security
- Android Animations
- Google Maps API
- Push Notifications
- Android Studio and Gradle
- Learn Version Control
- JUnit and Espresso for testing
- RESTful API and JSON parsing
- Dependency Injection with Dagger 2
- Reactive programming with RxJava
- Read Jetpack Compose
Android Architecture and Android Activity Lifecycle :
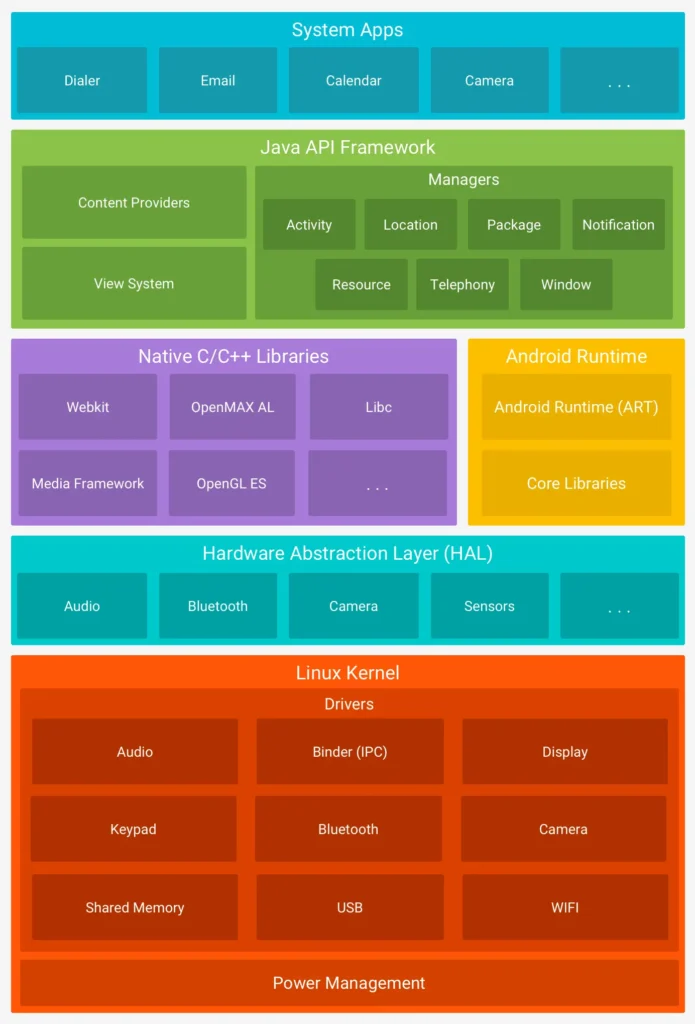
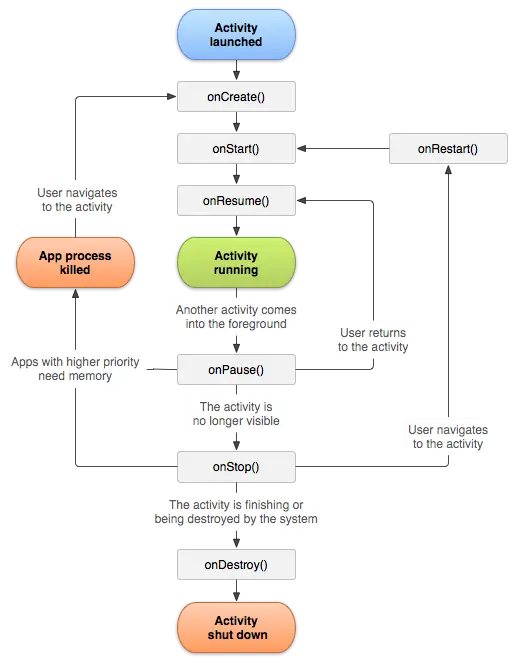
Components of Android :
Here are the main components of the Android operating system:
- Activities: represent the UI of an Android app and handle user interaction.
- Services: run in the background to perform long-running operations.
- Broadcast receivers: respond to system-wide broadcast announcements.
- Content providers: manage the sharing of app data with other apps.
- Intent: message objects that are used to request actions from other components.
- Views: basic UI building blocks for creating the user interface.
- Layouts: arrange views in a specific way to define the user interface.
- Widgets: are UI elements that can be placed on the home screen.
- Notification: provide the user with information about events that occur in the background.
- Fragments: represent a portion of the UI in an activity.
These components work together to create a complete, functional Android app.
What is the difference between Java and Kotlin?
Java and Kotlin are both programming languages used for developing Android applications. In the Below Table you can find the difference between java and Kotlin based on the Features.
Feature | Java | Kotlin |
---|---|---|
Syntax | Verbose | Concise |
Null safety | No built-in null safety, prone to null pointer exceptions | Has built-in null safety features |
Extension functions | No built-in support | Supports extension functions |
Lambdas | Java 8 introduced support for lambdas | Has full support for lambdas |
Interoperability with Java | Java and Kotlin code can be mixed seamlessly | – |
Community | Large, established community | Growing community |
Learning curve | Steep for beginners, as the syntax is verbose and difficult to read | Gentle for beginners, as the syntax is concise and easy to read |
Performance | Generally performs well, but can be slower for some operations compared to Kotlin | Generally performs better than Java for some operations |
Type inference | No type inference, requires explicit type declaration | Has type inference, reducing the amount of code needed to be written |
Syntactic sugar | Limited syntactic sugar | Rich set of syntactic sugar features, making code more concise and readable |
What are the Job responsibilities of the android developer?
Android developers’ duties vary by organisation and role, however below are some frequent tasks:
- Design and develop Android apps by writing code, testing, and debugging.
- Collaborate with cross-functional teams: Android developers engage with designers, product managers, and other stakeholders to understand project objectives and ensure the app satisfies user demands.
- Write clean, maintainable, and efficient code: Android developers must write code that is well-organized, comprehensible, and performs well on various Android devices.
- Debug and fix bugs: This involves finding and correcting issues during development and testing and evaluating the fixes to make sure the programme works.
- Stay current: Android programming is a quickly growing profession, thus Android developers must stay current on the latest tools, frameworks, and best practises to stay competitive and productive.
- Write unit tests: Android developers may write automated tests to test app components.
- Code reviews: Android developers can collaborate with other developers to evaluate code, enhance it, and exchange knowledge and best practises.
- Upload apps to the Google Play Store, release updates, and correct bugs and performance issues.
What are the job roles for android developers?
Android developer jobs include:
- Junior Android Developer: An entry-level position for Android developers. They may help senior developers code, test, and debug.
- Android Developers create, build, and maintain Android apps. They develop Android app features using Java and the Android SDK.
- Senior Android Developer: An experienced Android developer knows the platform and app development process. They may manage developers, oversee sophisticated app development, and assure Android app quality and performance.
- Android Engineers design Android apps. They develop user-friendly Android apps using their technological skills.
- Android Technical Lead: An Android Technical Lead manages the project’s technical components. They may mentor other developers, evaluate code, and improve Android app quality.
- Android App Architect: Android app architects develop high-level apps. They collaborate with developers, designers, and stakeholders to make the software scalable, maintainable, and user-friendly.
- Android Full-Stack Developer: An Android Full-Stack Developer can build an app’s front-end and back-end. They understand Android and have the abilities and knowledge to design a complete and working Android app.