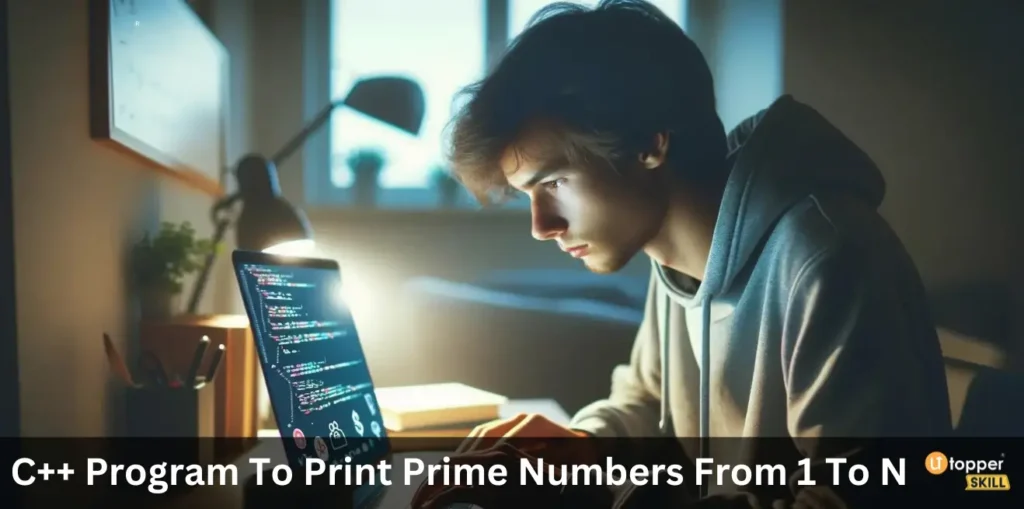
Explore our efficient C++ program to print prime numbers from 1 to N. Designed for beginners and advanced programmers alike, this guide provides a clear and concise program that systematically finds and prints prime numbers within a user-specified range. Whether you’re learning about prime numbers, enhancing your problem-solving skills, or seeking a practical implementation of loops and conditionals in C++, this example offers valuable insights.
What is Prime Numbers ?
Prime numbers are a fundamental concept in mathematics, particularly in the field of number theory. A prime number is defined as a natural number greater than 1 that has no positive divisors other than 1 and itself. In simpler terms, a prime number is a number that can only be divided evenly (without a remainder) by 1 and itself.
Examples: The first few prime numbers are 2, 3, 5, 7, 11, 13, 17, 19, 23, 29,…
Pseudo code for C++ Program to Print Prime Numbers From 1 to N.
Begin
Declare N, i, j
// Prompt user to enter the value of N
Print "Enter the value of N: "
Read N
// Loop from 2 to N (1 is not a prime number)
For i = 2 to N do
Declare isPrime = true
// Check if the number i is prime
For j = 2 to sqrt(i) do
If i is divisible by j then
Set isPrime = false
Break the loop
EndIf
EndFor
// If isPrime is true, print the number
If isPrime is true then
Print i
EndIf
EndFor
End
Explanation:
- Start: This signifies the beginning of the program.
- Declare variables: N, i, j, isPrime:
N
: This will hold the upper limit up to which prime numbers are to be found.i
andj
: These are loop counters.i
is used to iterate through each number from 2 to N, andj
is used for checking the divisibility ofi
.isPrime
: A boolean variable used to determine if the current numberi
is prime or not.
- Input the value of N: Here, the program will take an input from the user, which is the value of
N
, the upper limit for finding prime numbers. - For i = 2 to N, do steps 5-9: This is a loop that starts with
i = 2
and continues untili
equalsN
. Each iteration of this loop will check if the current value ofi
is a prime number.- Set isPrime = true: Initially, for each number
i
, it is assumed to be prime (isPrime
is set totrue
). - For j = 2 to i/2, do step 7: This is a nested loop inside the
i
loop. This loop checks if the numberi
is divisible by any number from 2 toi/2
. If a number is not divisible by any other number up to its half, it is prime.- If i mod j is 0, set isPrime = false and break the loop: This step checks if
i
is divisible byj
(using the modulus operator%
). Ifi
is divisible byj
, theni
is not a prime number, soisPrime
is set tofalse
, and the loop is exited using the break statement.
- If i mod j is 0, set isPrime = false and break the loop: This step checks if
- If isPrime is true, print i: After the inner loop, if
isPrime
is stilltrue
, it means thati
is a prime number, and it is printed.
- Set isPrime = true: Initially, for each number
- End For: This marks the end of the outer loop. The program will continue to iterate until all numbers from 2 to N have been checked.
- End: This signifies the end of the program.
Approach for C++ Program to Print Prime Numbers From 1 to N
To create a C++ program that prints prime numbers from 1 to N, you can follow this approach:
- Input the Upper Limit (N):
- Begin by asking the user to input the upper limit, N. This is the number up to which you want to find prime numbers.
- Initialize Variables:
- You’ll need variables for loop control (commonly
i
andj
) and a flag variable (likeisPrime
) to indicate whether a number is prime.
- You’ll need variables for loop control (commonly
- Iterate Through Numbers from 2 to N:
- Start a loop with
i
from 2 up to N (inclusive). We start from 2 because 1 is not considered a prime number.
- Start a loop with
- Check for Prime Numbers:
- For each number
i
, check if it is a prime number.- Set
isPrime
to true initially for eachi
. - Run an inner loop with
j
from 2 toi/2
. This range is sufficient because a number greater than half ofi
cannot evenly dividei
. - In the inner loop, check if
i
is divisible byj
(i.e.,i % j == 0
). If so, setisPrime
to false and break out of the inner loop, as this meansi
is not a prime number.
- Set
- For each number
- Print the Prime Number:
- After the inner loop, if
isPrime
is still true, it meansi
is a prime number, so printi
.
- After the inner loop, if
- Repeat for Next Number:
- Continue the outer loop to check the next number in the sequence until you reach N.
- End of Program:
- Once the loop completes, the program ends. All prime numbers between 1 and N will have been printed.
Logic for Prime Number from 1 to N
- Input the upper limit (N): Prompt the user to enter the value of
N
. - Check for prime numbers: For each number
i
from 2 toN
(since 1 is not a prime number), check if it is a prime number. - Prime number checking logic: A number is prime if it is only divisible by 1 and itself. So, for each number
i
, we check if it can be divided by any number from 2 tosqrt(i)
. If it can’t be divided by any of these numbers, it is a prime number. - Print the prime numbers: If a number is found to be prime, print it.
C++ Program to Print Prime Numbers From 1 to N
#include <iostream>int main() {
int N, i, j;
bool isPrime;
// Asking for upper limit
std::cout << "Enter the upper limit (N): ";
std::cin >> N;
// Loop through each number from 2 to N
for (i = 2; i <= N; i++) {
isPrime = true; // Assume the current number is prime
// Check if the number is divisible by any number from 2 to i/2
for (j = 2; j <= i / 2; j++) {
if (i % j == 0) {
isPrime = false; // If divisible, it's not prime
break; // No need to check further
}
}
// If isPrime is still true, the number is prime
if (isPrime) {
std::cout << i << " ";
}
}
return 0;
}
Explanation of the Program:
- Include Headers:
#include <iostream>
: This includes the IOstream library for input-output operations.
- Main Function:
int main() { ... }
: The entry point of the program.
- Variable Declaration:
int N, i, j;
: Declares integersN
(upper limit),i
(number to check), andj
(divisor).bool isPrime;
: A boolean variable to flag whether a number is prime.
- Input Upper Limit (N):
- The user is prompted to enter the upper limit
N
.
- The user is prompted to enter the upper limit
- Loop to Check Prime Numbers:
- The outer loop
for (i = 2; i <= N; i++)
iterates through each number from 2 to N. isPrime
is initially set totrue
for each numberi
.
- The outer loop
- Check for Divisibility:
- The inner loop
for (j = 2; j <= i / 2; j++)
checks ifi
is divisible by any number from 2 to its half. - If
i % j == 0
,isPrime
is set tofalse
, and the loop breaks.
- The inner loop
- Print Prime Numbers:
- If
isPrime
is true after the inner loop,i
is a prime number and is printed.
- If
- End of Program:
- The program ends with
return 0;
, which is a successful termination.
- The program ends with
When you run this program, it will ask for an upper limit N
. It will then print all prime numbers from 2 up to and including N
. The logic behind only checking up to i/2
for divisors is that a number cannot have a factor greater than half of its value, other than itself. This makes the program more efficient.
Output:
Enter the upper limit (N): 10
2 3 5 7
Other C++ Programs you can Read: