Finding out whether a given integer is even or odd is a common need in computer programming. Numerous applications, including sorting numbers, confirming user input, and carrying out mathematical calculations, can benefit from this. It is simple to detect whether a number is even or odd in the C programming language by using the modulus operator%. With the help of a straightforward conditional statement, we will show you how to use C to determine whether a given integer is even or odd.
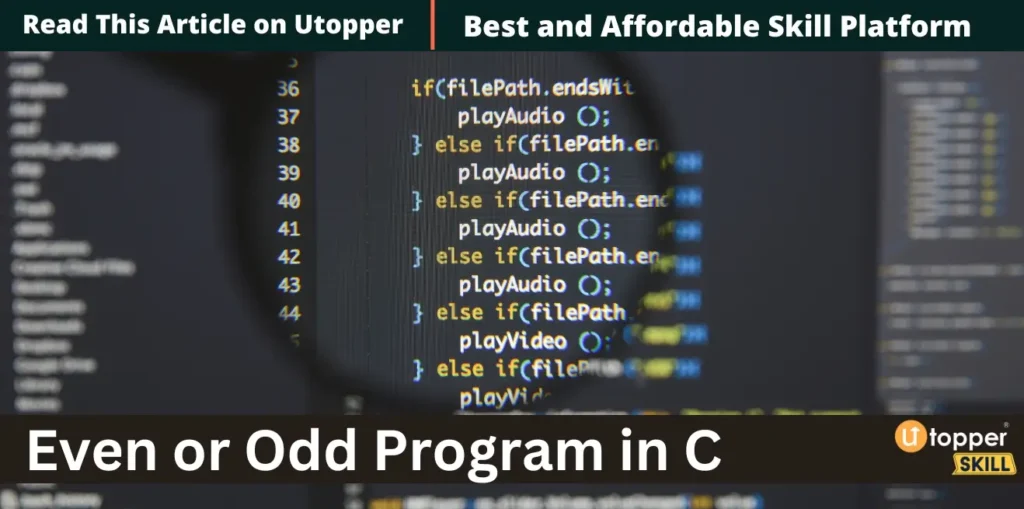
What is the odd number?
An odd number is a whole number that is not divisible by 2 without leaving a remainder. In other words, an odd number is any integer that can be expressed in form 2n + 1, where n is an integer. Examples of odd numbers include 1, 3, 5, 7, 9, -1, -3, -5, -7, and so on. The set of odd numbers can be represented as { …, -5, -3, -1, 1, 3, 5, … }.
What is the even number?
An even number is a whole number that is divisible by 2 without leaving a remainder. In other words, an even number is any integer that can be expressed in form 2n, where n is an integer. Examples of even numbers include 2, 4, 6, 8, 10, -2, -4, -6, -8, and so on. The set of even numbers can be represented as { …, -4, -2, 0, 2, 4, 6, … }.
Approach to find Even and Odd Numbers in c Language
To find whether a given number is even or odd in C language, you can follow this approach:
- Prompt the user to enter a number.
- Read the input number using
scanf()
. - Use the modulus operator
%
to check if the remainder of the division of the number by 2 is zero or not. - If the remainder is zero, then the number is even, and you can print a message indicating that.
- If the remainder is not zero, then the number is odd, and you can print a different message.
Pseudo code to check even or odd in C Language
Sure, here’s the pseudocode to check whether a given number is even or odd in a C program:
1. Prompt the user to enter a number
2. Read the input number
3. Check if the number is even or odd using the modulus operator %
4. If the result of the modulus operator is 0, the number is even
5. If the result of the modulus operator is not 0, the number is odd
6. Print the appropriate message based on whether the number is even or odd
C program to check even or odd numbers.
#include <stdio.h>
int main() {
int number;
// Step 1: Prompt the user to enter a number
printf("Enter a number: ");
// Step 2: Read the input number
scanf("%d", &number);
// Step 3: Check if the number is even or odd
if (number % 2 == 0) {
// Step 4: If the result of the modulus operator is 0, the number is even
printf("%d is even", number);
} else {
// Step 5: If the result of the modulus operator is not 0, the number is odd
printf("%d is odd", number);
}
return 0;
}
Output :
Enter a number: 10
10 is even.
Enter a number: 7
7 is odd.
Enter a number: -12
-12 is even.
C Program to Check Odd or Even Using the Ternary Operator
#include <stdio.h>
int main() {
int number;
printf("Enter a number: ");
scanf("%d", &number);
number % 2 == 0 ? printf("%d is even.\n", number) : printf("%d is odd.\n", number);
return 0;
}
The ternary operator?: checks whether the remainder of the number’s division by 2 is zero in this programme. We print “even” if the remainder is zero. Otherwise, we print an odd number message.
The ternary operator simplifies if-else statements. Ternary operators have this syntax:
condition ? value_if_true : value_if_false;
In the above program, the condition is number % 2 == 0
, which checks whether the remainder of the division of the number by 2 is zero or not. If the condition is true, the value of true is printf("%d is even.\n", number)
, which prints a message indicating that the number is even. Otherwise, the value if false is printf("%d is odd.\n", number)
, we print a message indicating the number is odd.
You can also check the Other C Programs :