Learn HTML
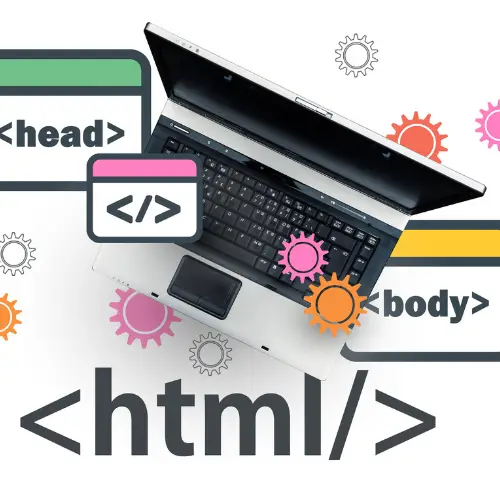
Page Index
HTML REFERENCES
What is HTML?
HTML (Hypertext Markup Language) is a markup language used to create and structure the content of a website. It defines the structure and layout of a webpage and is used in conjunction with other languages such as JavaScript and CSS to create interactive and visually appealing websites. It consists of a series of elements, such as headings, paragraphs, images, and links, that are defined using tags and attributes. A browser, such as Chrome or Firefox, reads the HTML code and renders it into a webpage that can be viewed by users.
What is the importance of HTML?
HTML is important for a number of reasons:
- Structure: It provides a way to structure the content of a webpage, including headings, paragraphs, lists, and other elements, making it easy for users to understand and navigate the content.
- Accessibility: HTML allows for the creation of accessible websites, which can be used by people with disabilities. This includes features such as alternative text for images, and proper use of headings to create a logical document structure.
- Interoperability: HTML is supported by all web browsers, which means that a webpage created using HTML will look the same across different devices and browsers, providing a consistent user experience.
- Search engine optimization (SEO): HTML provides a way to create well-structured, semantic content that search engines can easily understand and rank, which can help to improve a site’s visibility in search results.
- Interactivity: HTML can be combined with other languages such as JavaScript and CSS to create interactive and visually appealing websites, which can enhance the user experience.
- It’s a foundation of web development: HTML is the foundation of web development, which means that it is the first language that web developers learn when they start building websites. It provides the basic structure of the website, on which other languages like CSS and javascript are built.
What is the code of HTML?
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
</head>
<body>
<h1>Welcome to my website</h1>
<p>This is a simple webpage created using HTML.</p>
<img src="image.jpg" alt="My Image">
<a href="https://www.example.com">Visit my other website</a>
</body>
</html>
In this example, the <!DOCTYPE>
declaration specifies that this is an HTML5 document. The <html>
element is the root element of the document, and contains all other elements. The <head>
element contains meta information about the document, such as the title, which is displayed in the browser’s title bar or tab. The <body>
element contains the visible content of the webpage. Inside the body element, you can see <h1>
is used for the heading, <p>
is used for the paragraph, <img>
is used for the image, and <a>
is used for the hyperlink.
What is the Syntax of HTML?
The syntax of HTML consists of a series of elements, which are defined using tags. Each element has a specific purpose, such as creating headings, paragraphs, images, links, and other types of content.
Here is a brief overview of the syntax of HTML:
- Elements are defined using tags, which are enclosed in angle brackets
< >
. For example, the heading element is defined using the<h1>
tag. - An opening tag and a closing tag are used to define an element. The closing tag has a forward slash
/
before the element name. For example,<h1>
is the opening tag and</h1>
is the closing tag. - Elements can have attributes, which provide additional information about the element. Attributes are defined within the opening tag, and consist of a name and a value. For example, the
src
attribute of the<img>
element is used to specify the URL of the image. - Elements can be nested inside other elements to create a hierarchical structure. For example, a paragraph element
<p>
can be nested inside a div element<div>
- Comments can be added to the HTML code using the
<!--
and-->
syntax.
HTML code defines the structure and layout of a webpage, but it does not include any styling or formatting. This is where CSS (Cascading Style Sheets) comes in. CSS is used to control the appearance of elements on a webpage, such as their color, font, and layout.
Here is an example of HTML code that creates a simple webpage with a heading, a paragraph, and an image:
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
<style>
h1 {
color: blue;
}
p {
font-size: 16px;
}
</style>
</head>
<body>
<h1>Welcome to my website</h1>
<p>This is a simple webpage created using HTML and CSS.</p>
<img src="image.jpg" alt="My Image">
</body>
</html>
This code creates a simple webpage with a blue heading and 16px font size for the paragraph. The <style>
element is used to define CSS styles that will be applied to the elements on the webpage. The <h1>
and <p>
elements have CSS styles that define their color and font size, respectively. The <img>
element has an src
attribute that specifies the URL of the image, and an alt
attribute that provides alternative text for the image.
When this code is rendered in a web browser, it will display a webpage with a blue heading that says “Welcome to my website”, a paragraph that says “This is a simple webpage created using HTML and CSS”, and an image.