Top 35+ Git Interview Questions
Git interview questions: In preparation for a technical interview, it’s crucial to be well-versed in various aspects of Git and GitHub. Some common Git interview questions and answers might revolve around essential commands, such as ‘git commit,’ ‘git pull,’ and ‘git push,’ each with their specific functions. For GitHub interview questions, understanding how to effectively collaborate on projects using branches, pull requests, and merges is crucial.
Comprehensive knowledge of Git and GitHub can be tested through questions on resolving merge conflicts, reverting commits, and handling large repositories. Additionally, interviewers might ask for explanations of distributed version control, the advantages of Git commands in DevOps workflows, and scenarios where certain Git strategies are preferable. Preparing for these git questions with clear and concise answers can significantly enhance your performance during the interview.
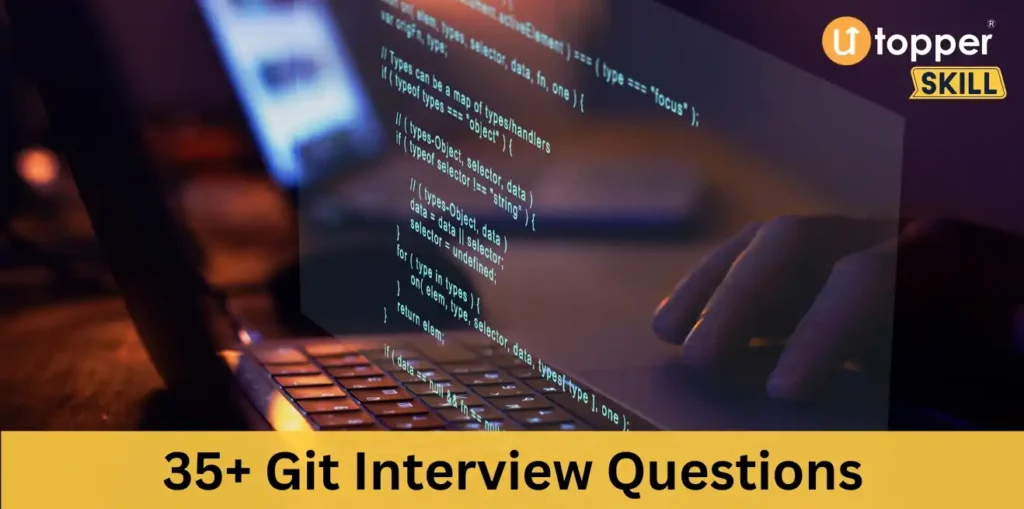
Q.1 What is Git?
Git is a distributed version control system used by software developers to track changes in their code. It was created by Linus Torvalds in 2005.
- Version Control: Git helps manage different versions of code. As you make changes to your code, Git allows you to save these changes as different versions. This makes it easy to go back to previous versions, compare changes over time, and merge changes from different branches.
- Distributed Architecture: Unlike some other version control systems, Git is distributed, meaning that every developer’s copy of the codebase is also a full-fledged repository with complete history and tracking capabilities. This allows for more flexibility in how teams can work together and makes it possible to work offline.
- Branching and Merging: Git’s branching model allows developers to create isolated environments within the same codebase to work on individual features or bug fixes. Once the work on a branch is complete, it can be merged back into the main code line. This enables concurrent development without conflicts.
- Conflict Resolution: When different team members are working on the same parts of the code, conflicts can occur. Git provides tools to easily identify and resolve these conflicts, ensuring that changes are integrated smoothly.
- Integration with Development Tools: Git is supported by most Integrated Development Environments (IDEs) and can be integrated into various development workflows and collaboration platforms like GitHub, GitLab, and Bitbucket.
- Open Source: Git is free and open-source software, so it can be used, modified, and shared by anyone.
- Performance: Git is known for its speed and efficiency, especially when compared to some other version control systems.
- Security: Git uses SHA-1 to manage its version history securely, ensuring the integrity and consistency of the codebase.
Q.2 What are the advantages of using Git?
Git is a distributed version control system that is used in software development to track changes in source code. It provides a number of advantages that make it popular among developers:
- Version Control: Git enables you to track changes, making it easy to revert to previous versions of your code. This is essential for identifying when and where bugs were introduced.
- Branching and Merging: Git’s branching model allows developers to work on different parts of a project simultaneously. Branches can be easily merged, and conflicts are usually easy to resolve. This makes team collaboration smoother and more efficient.
- Distributed Development: Every user has a complete copy of the entire project history, enabling them to work offline or without a central server. This also means that if one repository is lost, the code can be recovered from any of the developers’ local repositories.
- Concurrency and Collaboration: Multiple developers can work on the same project without much hassle. Git provides tools to handle conflicts if two developers make conflicting changes.
- Speed and Efficiency: Git is optimized for performance. Operations like branching, merging, and committing changes are very fast, making the development process more efficient.
- Integrity: Git uses cryptographic methods to keep track of changes, ensuring the integrity of your code. Once committed, it’s almost impossible to alter history without detection.
- Integration with Other Tools: Git integrates with various development and continuous integration tools. This makes it a versatile choice for different types of projects and workflows.
- Open Source: Git is free and open-source, meaning that it’s accessible to anyone and can be modified if needed.
- Support and Community: Since Git is widely used, there is an extensive community, plenty of documentation, tutorials, and support, making it easier to learn and troubleshoot issues.
- Reduces Risk: By maintaining a complete history of changes and facilitating branching, Git helps in reducing the risk of project derailment due to errors or unforeseen problems.
- Scalability: Git can handle large codebases efficiently, making it suitable for both small projects and large enterprise applications.
- Stashing: Git allows you to “stash” changes, meaning that you can save changes that haven’t been committed, switch to a different branch, and then come back to those changes later. This is very useful for maintaining a clean workflow.
- Blame Feature: Using the “blame” function, you can easily trace changes to their origin, identifying when a particular line of code was altered and by whom.
- Customizable: Git’s behavior can be customized according to the requirements of a project or a team by using different kinds of hooks and scripts.
- Platform Independent: Git is available on most platforms, providing a consistent experience across different operating systems.
Q.3 What is a Git repository?
A Git repository is a collection of files and their complete history of changes. It is a core component of the Git version control system, which allows multiple developers to collaborate on a project without interfering with each other’s work.
- Version Control: Git repositories enable tracking of changes to code over time. Every change, or “commit,” includes a snapshot of the files at that point, a reference to the parent commit, the author of the changes, a timestamp, and a message describing the changes.
- Branching and Merging: Within a repository, different branches can be created to work on various features or bug fixes independently. When those changes are ready, they can be merged back into the main branch.
- Remote Repositories: Git allows for both local and remote repositories. Local repositories reside on the developer’s machine, while remote repositories exist on a server. Remote repositories enable collaboration among multiple developers.
- Conflict Resolution: If two developers modify the same part of a file simultaneously, Git provides tools to resolve conflicts and merge the changes manually.
- History and Auditing: You can view the entire history of changes to the project by examining the commits in the Git repository. This is useful for understanding how the code has evolved, identifying when a bug was introduced, and so on.
- Distributed Architecture: Git is distributed, meaning every developer has a complete copy of the project history on their local machine. This allows for work to be done offline and enables better performance and flexibility.
Q.4 How do you create a new Git repository?
Creating a new Git repository is a common task and can be done with a few simple commands. Here’s a step-by-step guide:
1. Install Git (if not already installed)
First, make sure you have Git installed on your computer. If not, you can download and install it from the Official Git website.
2. Open a Terminal or Command Prompt
You’ll need to use a command-line interface to interact with Git.
3. Navigate to Your Project Directory
Use the command line to navigate to the directory where you want to create the repository. If the directory doesn’t exist, you’ll need to create it. Here’s how you can do that:
mkdir my_project
cd my_project
Replace my_project
with the name, you want for your project directory.
4. Initialize the Git Repository
Once you’re in your project directory, run the following command to initialize a new Git repository:
git init
This command will create a new subdirectory named .git
that contains all the necessary Git files. Your project directory is now a Git repository, and you can start tracking changes to your files.
5. Add Files (Optional)
If you already have files in the directory, you can add them to the Git repository:
git add .
The .
adds all files in the current directory to the repository. If you want to add specific files, you can replace the .
with the filenames.
6. Commit Your Initial Changes (Optional)
After adding the files, you can make your first commit to save the current state of your project:
git commit -m "Initial commit"
The text inside the quotes is a commit message, and it’s a description of the changes you’ve made.
Q.5 What is a branch in Git and why to use it ?
In Git, a branch is a separate line of development within a repository. It represents an independent series of changes, allowing you to work on different parts of a project simultaneously without affecting other work. Here’s an overview of what a branch is and why it’s useful:
What is a Branch?
- Separate Line of Development: A branch allows you to isolate your work on a particular feature, bug fix, or experiment from the rest of the project. This makes it easier to manage complex projects with many moving parts.
- Pointers to Commits: Technically, a branch in Git is a simple pointer to a specific commit. When you create a new branch, you’re essentially creating a reference to the current state of your project. As you make new commits on that branch, the pointer updates to refer to the latest commit.
- Main and Feature Branches: By convention, most Git repositories have a primary branch (often called “main” or “master”) that represents the official, stable version of a project. Other branches, such as feature or bug-fix branches, diverge from this main line of development and are merged back in when the work is complete.
Why Use Branches?
- Collaboration: Multiple developers can work on different branches simultaneously without conflicting with each other. This allows for parallel development on various parts of a project.
- Experimentation: Branches provide a safe space to try out new ideas or experiments without affecting the main codebase. If the experiment is successful, it can be merged into the main branch. If not, it can be discarded without any harm to the main project.
- Code Review and Quality Control: By working on separate branches, you can submit changes for review and testing before they are integrated into the main codebase. This can improve code quality and ensure that only well-vetted changes are added to the main project.
Common Commands
Here are a few common Git commands related to branches:
- Create a New Branch:
git branch branch-name
- Switch to a Branch:
git checkout branch-name
- Create and Switch to a New Branch:
git checkout -b branch-name
- Merge a Branch into the Current Branch:
git merge branch-name
- Delete a Branch:
git branch -d branch-name
Q.6 How do you create a new branch in Git?
Creating a new branch in Git is a straightforward process that can be accomplished with a few simple commands. Here’s how you can do it:
Step 1: Open a Terminal or Command Prompt
First, you’ll need to use a command-line interface to interact with Git.
Step 2: Navigate to Your Git Repository
Use the command line to navigate to the directory where your Git repository is located.
Step 3: Check Current Branches
Before creating a new branch, you might want to see what branches already exist. You can do that with the following command:
git branch
Step 4: Create the New Branch
To create a new branch, use the git branch
command followed by the name you want to give the new branch. Here’s an example:
git branch my-new-branch
Replace my-new-branch
with whatever name you’d like to give the branch.
Step 5: Switch to the New Branch
Creating the new branch doesn’t automatically switch to it; you remain on the branch you were on previously. To switch to the new branch, use the git checkout
command:
git checkout my-new-branch
Again, replace my-new-branch
with the name of the branch you just created.
Alternative: Create and Switch to a New Branch in One Step
Alternatively, you can create and switch to a new branch in one step using:
git checkout -b my-new-branch
This will both create the new branch and immediately switch to it.
Additional Notes
- Branch names should not contain spaces.
- It’s a good practice to name branches in a way that describes their purpose (e.g.,
feature-login
,bugfix-header
). - Make sure to commit any outstanding changes on the current branch before switching to a new branch, as uncommitted changes will carry over between branches.
Creating a branch in Git allows you to work on different parts of a project simultaneously, isolating your work from other branches. It’s a powerful tool for collaborative development and managing multiple tasks within a single repository.
Q.7 What is the difference between Git merge and Git rebase?
Both git merge
and git rebase
are commands used to integrate changes from one branch into another within Git, but they do so in different ways. Here’s a detailed explanation of the differences:
Git Merge
- Functionality:
git merge
takes the contents of a source branch and integrates it with a target branch. - History: The history of the branch is maintained. When you merge one branch into another, a new “merge commit” is created, and the history of the commits from both branches is preserved. This results in a non-linear, bifurcating history.
- Use Case: Ideal when you want to combine code from two different branches and maintain the complete history of changes, such as merging a feature branch into the main branch.
Example:
git checkout main
git merge feature-branch
This will merge the feature-branch
into main
, creating a new merge commit in the process.
Git Rebase
- Functionality:
git rebase
takes the changes made in one branch and “replays” them on top of another branch. Essentially, it moves or “rebases” a branch onto the base of another branch. - History: The history is rewritten to produce a linear sequence of commits. It essentially moves the entire feature branch to begin on the tip of the master branch, discarding the specific historical context where the changes originally occurred.
- Use Case: Often used to make local, private branches cleaner and easier to follow by putting them in a linear history. This can simplify understanding the history of the project but may not be suitable for public branches where others may have based work on them.
Example:
git checkout feature-branch
git rebase main
This will replay the changes made in feature-branch
on top of main
, making it look as though the changes were made on top of the latest commit in main
.
Key Differences:
- History Preservation:
merge
maintains the history, whereasrebase
creates a new, linear history. - Commits:
merge
creates a new merge commit, whereasrebase
rewrites the commit history. - Conflicts: In both operations, conflicts can arise. However, with
rebase
, conflicts are resolved one commit at a time as changes are reapplied, whereas, withmerge
, conflicts are resolved all at once.
Q.8 What is Git stash?
git bisect is a powerful tool in Git used for finding the commit that introduced a bug or undesirable behavior into a codebase. It uses a binary search algorithm to quickly and efficiently identify the specific commit that caused the problem. Here’s an overview of how it works and why it’s useful:
How Git Bisect Works
- Start the Process: You begin by telling Git to start the bisecting process:
git bisect start
2. Mark the Known Good and Bad Commits: You then need to mark a known ‘bad’ commit (usually the current commit where the bug is present) and a known ‘good’ commit (a commit where the code was working correctly).
git bisect bad # Marks the current commit as bad
git bisect good <hash> # Marks a specific commit as good
3. Binary Search: Git will then check out a commit that lies halfway between the known good and bad commits. You test this commit to determine whether it’s good or bad, and then tell Git the result:
git bisect good # or git bisect bad
4. Repeat: Git continues to narrow down the range, checking out commits halfway between the known good and bad points, and you continue to mark them as good or bad. This process repeats until Git identifies the first bad commit.
5. End the Bisect Process: Once the culprit commit has been found, you’ll likely want to exit the bisect mode:
git bisect reset
Why Use Git Bisect?
- Efficient Debugging: By using a binary search algorithm,
git bisect
can find the problematic commit in a logarithmic number of steps, making it an efficient way to pinpoint issues even in large codebases with extensive histories. - Automating the Process: If you have a script that can test whether a commit is good or bad, you can automate the bisecting process with
git bisect run <script>
, making it even quicker and more convenient. - Understanding Regression: When a previously working feature breaks,
git bisect
is a valuable tool to understand exactly when and why the regression occurred.
Q. 9 How do you resolve a merge conflict in Git?
Resolving a merge conflict in Git involves manually addressing conflicting changes between two branches that are being merged. Merge conflicts occur when Git cannot automatically reconcile differences between the changes made in different branches. Here’s a step-by-step guide on how to resolve a merge conflict in Git:
- Identify the Conflict:
- When you attempt to merge two branches using
git merge
orgit pull
, Git will notify you if there are conflicts. - Running
git status
will display the list of conflicted files with the term “both modified” or similar messages.
- When you attempt to merge two branches using
- Open the Conflicted File:
- Use a text editor or Git GUI tool to open the conflicted file. Inside the file, you will see markers that indicate the conflicting changes.
- Understanding Conflict Markers:
- Conflict markers usually look like this:
<<<<<<< HEAD
// Your changes in the current branch
=======
// Changes from the branch you are merging into the current branch
>>>>>>> branch-name
- The content between
<<<<<<< HEAD
and=======
represents the changes in the current branch, and the content between=======
and>>>>>>> branch-name
represents the changes in the other branch you are merging.
- Resolve the Conflict Manually:
- Edit the conflicted file, keeping only the desired changes and removing the conflict markers. You may also modify the content to combine the conflicting changes if needed.
- Save the File:
- After resolving the conflict, save the file with the changes you’ve made.
- Stage the File:
- Use the command
git add <file>
to stage the resolved file. This marks the conflict as resolved for the file.
- Use the command
- Commit the Merge:
- Once all conflicts in the project have been resolved and staged, commit the merge with
git commit
. Git will create a new merge commit with the resolved changes.
- Once all conflicts in the project have been resolved and staged, commit the merge with
- Complete the Merge:
- If there are no other conflicts, the merge is now complete, and the branches are successfully merged.
- Push the Changes:
- If you are working with a remote repository, push the merged changes using
git push
.
- If you are working with a remote repository, push the merged changes using
It’s important to carefully review and test the resolved changes to ensure that the merge was successful and that no unintended issues were introduced. In cases where conflicts are complicated or numerous, collaborating with other team members can be helpful to ensure a thorough resolution.
Q.10 What is Git clone?
Git Clone: Copying a Git Repository
Git clone is a command in the Git version control system that allows you to create a copy of an existing Git repository. It is used to fetch the entire repository, including all its history, branches, and files, from a remote source and place it on your local machine. Cloning is a fundamental operation in Git that enables collaboration, contribution, and version control for developers.
Key Points about Git Clone:
- Copy Entire Repository: When you run
git clone <repository_url>
, Git creates a duplicate of the specified repository, including all the commits, branches, tags, and files. - Remote Source: The
<repository_url>
parameter in thegit clone
command points to the remote location of the repository you want to clone. This can be a URL starting withhttps://
,git://
,ssh://
, or a local file path. - Complete History: Cloning fetches the entire history of the repository, allowing you to access and explore the project’s development timeline.
- Local Copy: The cloned repository is a standalone copy that exists on your local machine. You can work with it independently and make changes without affecting the original remote repository until you push your changes back.
- Branches and Tags: All branches and tags present in the remote repository are replicated in the local clone, making it easy to switch between different versions or work on specific branches.
- Working Directory: After cloning, you have a working directory with the latest version of the project files. You can immediately start working on the project, modifying files, and making commits.
- Full Version Control: Git clone preserves the entire version control history, allowing you to review the project’s development, trace changes, and collaborate effectively with other developers.
- Collaboration and Contributions: Cloning is the starting point for collaborative development. Developers clone the repository to contribute changes and propose modifications to the project.
How to Use Git Clone:
To clone a Git repository, use the following command:
git clone <repository_url>
Replace <repository_url>
with the URL of the remote Git repository you want to clone. The URL can start with https://
, git://
, ssh://
, or be a local file path.
Why Use Git Clone:
- Collaboration: Cloning is essential for collaborative development. It allows multiple developers to work on the same project simultaneously and independently. Each developer can clone the repository to their local machine, make changes, and contribute to the project.
- Version Control: Git clone provides a complete copy of the project’s version history. This means you have access to the entire development timeline, including all commits, branches, and tags. It enables developers to track changes and understand the evolution of the project.
- Isolation: When you clone a repository, you create a separate and isolated working environment. This means you can experiment with changes, create new branches, and test features without affecting the original repository or other developers’ work.
- Offline Work: After cloning, you have a local copy of the entire repository. This allows you to work on the project even when you don’t have an internet connection. You can commit changes, create branches, and switch between versions without needing an active connection to the remote repository.
- Backup and Redundancy: Cloning provides redundancy by creating multiple copies of the repository. If the remote server becomes unavailable or data is lost, you can rely on the cloned repositories for backup and recovery.
- Faster Operations: Working with a local clone typically results in faster operations compared to directly interacting with a remote repository. Commits, diffs, and other Git commands are usually faster on a local machine.
- Safe and Secure: Cloning allows you to experiment with changes and test new features without risking the integrity of the main repository. This safety net promotes a culture of experimentation and innovation.
Q.11 What is Git fetch?
git fetch
is a command used in Git to retrieve updates from a remote repository. It downloads the latest updates and references from the remote repository, but unlike git pull
, it doesn’t automatically merge those changes into your current branch. Here’s a detailed explanation:
What git fetch Does
- Retrieving Updates:
git fetch
contacts the remote repository and fetches any new branches, tags, and updates to existing branches that have been pushed by others but don’t yet exist in your local repository. - Updating Remote-tracking Branches: Your local repository keeps references to the state of remote branches.
git fetch
updates these references to reflect the current state of the remote repository. - No Automatic Merging: Unlike
git pull
, which is a combination ofgit fetch
followed bygit merge
, thegit fetch
command alone doesn’t alter your working directory or your current branch. It merely downloads the updates, allowing you to review or merge them manually when you’re ready.
Usage
You can run git fetch
with or without additional parameters:
- Fetching All Branches: Simply run
git fetch
, and Git will fetch updates for all branches from the configured remote repository. - Fetching a Specific Remote: If you have multiple remotes configured, you can specify which one to fetch from:
git fetch <remote-name>
- Fetching a Specific Branch: If you want to fetch a specific branch from a remote repository, you can specify it:
git fetch <remote-name> <branch-name>
Why Use git fetch?
- Review Before Merging: By fetching without merging, you can inspect the updates before deciding whether or how to integrate them into your local branches.
- Keep Up-to-date: It’s a way to keep your local repository informed about the state of the remote repository without altering your working state.
- Flexibility: You can decide whether to merge or rebase the changes as you see fit, giving you more control over how you incorporate remote updates.
Q.12 What is Git pull?
git pull
is a command used in Git to update the local branch with the latest changes from a corresponding remote branch. It’s a commonly used command in collaborative environments where multiple people might be working on the same project. Here’s an overview of what git pull
does and how it’s used:
What git pull
Does
- Fetches Changes: The
git pull
command first performs agit fetch
, retrieving the latest updates from the remote repository. This includes changes to branches, new branches, tags, and more. - Merges Changes: After fetching the changes,
git pull
then automatically merges the updates from the remote tracking branch into the current local branch. If the local branch is behind the remote branch, the changes are applied to bring the local branch up to date.
Usage
Here’s the basic syntax for git pull
:
git pull <remote> <branch>
<remote>
is the name of the remote repository (e.g.,origin
).<branch>
is the name of the branch you want to pull from.
If you’re already on the branch that you want to update, and the remote repository is configured correctly, you can simply use:
git pull
Merge Conflicts
If there are changes in the remote repository that conflict with your local changes, a merge conflict may occur. When this happens, you’ll need to manually resolve the conflict by editing the conflicting files, and then commit the resolved changes.
Pull with Rebase
If you prefer, you can use the --rebase
option to reapply your local changes on top of the incoming changes rather than merging them:
git pull --rebase <remote> <branch>
This can create a cleaner, more linear history by avoiding unnecessary merge commits.
Why Use git pull
?
- Synchronization:
git pull
allows you to stay synchronized with a remote repository, making sure your local copy is up to date with the latest changes made by other collaborators. - Convenience: It’s a quick way to fetch and merge changes in one step, streamlining your workflow.
- Collaboration: It’s essential in a collaborative environment to ensure that everyone’s work is integrated and that you’re working with the most recent version of the code.
Q.13 What is Git push?
git push
is a command used in Git to upload local repository content to a remote repository. It’s the counterpart to git pull
, and it’s essential for collaborating with others on a shared codebase. Here’s an overview of what git push
does and how it’s used:
What git push
Does
- Uploads Changes: The
git push
command takes the commits from your local branch and pushes them to the corresponding branch on the remote repository. This shares your changes with others and updates the remote repository with your local commits. - Updates Remote Branch: If the branch doesn’t exist on the remote,
git push
can create it, and if it does exist,git push
updates it with the new commits. - Requires Write Access: To push changes to a remote repository, you must have the necessary permissions to write to that repository.
Usage
Here’s the basic syntax for git push
:
git push <remote> <branch>
<remote>
is the name of the remote repository (e.g.,origin
).<branch>
is the name of the branch you want to push.
You can also push all branches to the remote repository using:
git push --all <remote>
Handling Non-Fast-Forward Errors
If the remote branch has changes that you don’t have locally, you may encounter a non-fast-forward error when trying to push. This means that you must first fetch and integrate the remote changes (using git pull
or git fetch
and git merge
/git rebase
) before pushing your local changes.
Force Pushing
In some cases, you might want to overwrite the remote branch, even if it contains changes you don’t have locally. This can be done with a force push:
git push <remote> <branch> --force
Caution: Force pushing can overwrite changes on the remote branch and is generally considered risky, especially on shared branches. It’s usually recommended only for branches where you are the sole collaborator.
Why Use git push
?
- Sharing Changes:
git push
enables you to share your local changes with other collaborators, ensuring that everyone has access to the latest version of the code. - Backup: Pushing changes to a remote repository also serves as a form of backup, safeguarding your work.
- Collaboration Workflow: It’s a key part of the typical collaborative workflow, allowing multiple developers to contribute to the same project and keep everything synchronized.
Q.14 What is Git tag?
Git tag is a feature in the Git version control system that allows you to label or mark specific commits in your repository with a descriptive name. Tags provide a way to easily reference significant points in your project’s history, such as version releases, milestones, or important changes. They help make it easier to navigate through the commit history and find specific points of interest.
Key Points about Git Tag:
- Labeling Commits: A Git tag is like a bookmark or label assigned to a specific commit. It is a meaningful name that you give to a particular point in your project’s history.
- Creating Tags: You can create a tag using the
git tag
command followed by the tag name and the commit hash you want to tag. For example,git tag v1.0 <commit_hash>
. - Lightweight Tags: By default, Git creates lightweight tags, which are simply pointers to specific commits. Lightweight tags are easy to create and delete but lack additional metadata.
- Annotated Tags: You can also create annotated tags using the
a
flag, which includes extra information such as the tagger’s name, email, date, and an optional message. Annotated tags are useful for version releases or documenting important changes. - Listing Tags: To see a list of all tags in your repository, use the command
git tag
. This will display the names of all existing tags. - Tagging Specific Commits: You can tag specific commits based on their commit hashes. This allows you to label critical milestones, releases, or significant changes.
- Lightweight vs. Annotated Tags: Lightweight tags are generally used for personal or temporary references, while annotated tags are often preferred for official releases and long-term references.
- Pushing Tags: By default, when you push changes to a remote repository, Git does not push tags. You need to explicitly use
git push --tags
to push all tags to the remote repository. - Tagging Past Commits: You can add tags to past commits even if you didn’t tag them initially. Use
git tag -a <tag_name> <commit_hash>
to retroactively tag a commit.
Usage of Git Tag:
To create a lightweight tag, use the following command:
git tag <tag_name> <commit_hash>
To create an annotated tag with additional information, use:
git tag -a <tag_name> <commit_hash> -m "Tag message"
Q.15 What is Git cherry-pick?
Git Cherry-Pick: Applying Specific Commits to Another Branch
Git cherry-pick is a command in the Git version control system that allows you to apply the changes introduced by specific commits to a different branch. It enables you to select individual commits from one branch and “cherry-pick” them onto another branch, incorporating only the desired changes without merging the entire branch history.
Key Points about Git Cherry-Pick:
- Selecting Specific Commits: With
git cherry-pick
, you can choose specific commits from one branch and apply them to another branch independently of the branch’s entire commit history. - No Branch Merging: Unlike
git merge
, which combines entire branch histories, cherry-picking allows you to pick and choose individual commits to apply to a target branch, preserving a clean and selective commit history. - Isolated Changes: Cherry-picking is particularly useful when you want to introduce specific bug fixes, features, or changes from one branch to another without including other unrelated commits.
- Applying Patch: When you cherry-pick a commit, Git creates a new commit with the same changes as the original commit. This creates a patch of the selected commit, which is then applied to the target branch.
- Conflict Resolution: In cases where the changes from the cherry-picked commit conflict with the target branch’s code, Git will notify you about the conflicts. You’ll need to resolve these conflicts manually before committing the changes.
- Cherry-Picking Multiple Commits: You can cherry-pick multiple commits at once by providing their commit hashes in the
git cherry-pick
command. - Reordering Commits: Cherry-picking also allows you to apply commits in a different order on the target branch, providing flexibility in how you incorporate changes.
- Commit Hash Identification: To cherry-pick a commit, you need to identify its unique commit hash or use other Git references to locate the desired commit.
Usage of Git Cherry-Pick:
To cherry-pick a commit onto the current branch, use the following command:
git cherry-pick <commit_hash>
To cherry-pick multiple commits, provide their commit hashes:
git cherry-pick <commit_hash1> <commit_hash2> ...
Q.16 What is Git blame?
Git Blame: Tracking Changes to Specific Lines in a File
Git blame is a command in the Git version control system that allows you to view the commit history of a file and track changes made to specific lines within that file. It shows which commit and author last modified each line of code, providing valuable insights into the history and responsibility of each change.
Key Points about Git Blame:
- Line-Level History: Git blame operates on a per-line basis. It displays the commit hash, author name, and timestamp for the last modification of each line in a file.
- Identifying Changes: With
git blame
, you can identify when and by whom each line was last changed. This information helps with code accountability and understanding the evolution of the file. - Identifying Authors: Git blame displays the author name associated with each line’s last modification, allowing you to see who made specific changes.
- Commit Hash: For each line,
git blame
shows the commit hash associated with the last modification. This enables you to locate the complete commit history for that change. - Timestamps: The command also shows the timestamp of each line’s last modification, providing details about when the changes were made.
- Blame Annotations: When running
git blame
, Git can annotate the file with blame information, visually displaying the commit and author details next to each line. - Line Ranges: You can specify line ranges with
git blame
to restrict the output to specific parts of the file, useful for investigating particular sections or changes. - Ignoring Whitespaces: By default,
git blame
considers whitespace changes as significant. However, you can use thew
option to ignore whitespace differences when tracking line changes.
Usage of Git Blame:
To view line-by-line annotations for a file, use the following command:
git blame <file_name>
To view annotations for specific lines within a file, specify the line numbers:
git blame -L <start_line_number>,<end_line_number> <file_name>
Q.17 What is Git diff?
Git Diff: Displaying Differences Between Commits, Branches, and Files
Git diff is a command in the Git version control system that shows the differences between different states of a Git repository. It allows you to compare changes between commits, branches, or even individual files. The output of the git diff
command displays the added, modified, and deleted lines, enabling developers to understand the code changes and track modifications in the project.
Key Points about Git Diff:
- Comparing Commits: With
git diff
, you can compare the content differences between two commits. This helps you understand what has changed in the codebase over time. - Comparing Branches: Git diff allows you to compare the differences between two branches. It helps you see what changes have been made in one branch compared to another.
- Comparing Files: You can also use
git diff
to compare changes between two different versions of a single file, showing modifications line by line. - Unified Diff Format: The default output of
git diff
is in the unified diff format. It displays the changes for each modified file, highlighting added and deleted lines with a +/- notation. - Context Lines: Git diff also includes context lines to provide additional context around the changes, making it easier to understand the modifications in the context of the surrounding code.
- Staged Changes: By default,
git diff
shows the differences between the working directory and the most recent commit. You can usegit diff --staged
to compare changes between the staged (index) area and the last commit. - Comparing Specific Commits: You can specify commit hashes or branch names in
git diff
to compare specific commits or branches. - Comparing with Ancestor: Using the
^
symbol, you can compare a commit with its parent to see what was changed in that particular commit.
Usage of Git Diff:
To see the differences between the working directory and the last commit, use the following command:
git diff
To compare staged changes (index) with the last commit, use:
git diff --staged
To compare two specific commits, use their commit hashes:
git diff <commit_hash1> <commit_hash2>
Q.18 What is the difference between Git and Github?
- Git is the underlying system that manages the source code, allowing developers to track changes, collaborate, and manage versions.
- GitHub is a platform that hosts Git repositories and provides additional tools and features to enhance collaboration, project management, and development workflows.
Aspect | Git | GitHub |
---|---|---|
Nature | Distributed Version Control System | Web-based Hosting Platform for Git Repositories |
Primary Function | Tracking changes, merging code, version management | Hosting Git repositories, collaboration, project management |
Usage | Command-line interface, GUIs available | Web interface, desktop clients, mobile apps |
Accessibility | Local and remote repositories, platform agnostic | Centralized online platform |
Open Source | Yes | No (but hosts open-source projects) |
Collaboration Tools | Basic collaboration via branches, merges, etc. | Enhanced collaboration via pull requests, issues, etc. |
Hosting | Can be self-hosted or hosted on various platforms | Hosted on GitHub’s servers |
Pricing | Free | Free for public repositories, paid plans for private/teams |
Q.19 What is Git reset?
Git Reset: Undoing Changes and Moving Branch Pointers
Git reset is a command in the Git version control system that allows you to undo changes and move branch pointers to a different commit. It is a powerful and flexible command used to manipulate the history of a Git repository.
Key Points about Git Reset:
- Moving Branch Pointers: Git reset primarily moves the branch pointer to a specific commit, effectively changing the branch’s reference point to that commit.
- Resetting Commit History: Depending on the reset mode used,
git reset
can discard, unstage, or move commits, effectively altering the commit history. - Three Reset Modes: Git reset offers three main modes:
-soft
,-mixed
(default), and-hard
.-soft
: Moves the branch pointer to the specified commit, leaving all changes staged. It does not modify the working directory or undo any changes.-mixed
: This is the default mode. It moves the branch pointer to the specified commit and unstages the changes, keeping them in the working directory. It effectively undoes the last commit but leaves the changes intact.-hard
: Moves the branch pointer to the specified commit and resets the staging area and the working directory to match the state of that commit. It completely discards all changes made after the specified commit.
- Changing Branch History: Git reset can rewrite the commit history, especially when combined with the
-force
option. This is a powerful operation and should be used with caution, especially if you are collaborating with others on the same repository. - Discarding Commits: By using
git reset
to move the branch pointer backward, you can effectively discard commits that are no longer needed in the project history. - Resetting to Specific Commit: You specify the commit you want to move the branch pointer to by providing its commit hash as an argument to the
git reset
command.
Usage of Git Reset:
To move the branch pointer and undo the last commit, use the following command for the default --mixed
mode:
git reset HEAD^
To completely remove the last commit and all changes, use --hard
mode:
git reset --hard HEAD^
Q.20 What is the difference between Git rebase and Git merge? When would you use one over the other?
Git rebase and Git merge are both used to integrate changes from one branch into another, but they do so in different ways, leading to different histories and workflows. Here’s a comparison of the two, along with guidance on when to use each:
Git Merge
What It Does:
- Combines the contents of two branches by creating a new merge commit.
- Preserves the chronological order of commits on both branches.
- Maintains the original branch history.
When to Use:
- When you want to combine code from two different branches and preserve the complete history and context of all changes.
- When working on shared or public branches, where you want the history to reflect the collaborative process.
Pros:
- Simple to understand and use.
- Preserves the entire history of all changes.
Cons:
- Can lead to a more complex and tangled commit history, especially in highly collaborative environments.
Git Rebase
What It Does:
- Moves or “replays” commits from one branch on top of another.
- Creates a new commit for each original commit, leading to a linear, clean history.
- Modifies the existing commit history, discarding the original branch’s separate history.
When to Use:
- When you want to make your feature branch up-to-date with the latest code from the main branch.
- When working on a private or feature branch, where a linear history makes it easier to understand the development process.
Pros:
- Provides a cleaner and more linear commit history.
- Eliminates unnecessary merge commits, making the history easier to follow.
Cons:
- Rewrites commit history, which can be risky on shared branches.
- Loses the context of the branch structure, as it makes the history linear.
Comparison Table
Aspect | Git Merge | Git Rebase |
---|---|---|
Commit History | Non-linear, preserves all commits | Linear, rewrites commits |
Result | Creates a new merge commit | Moves existing commits |
Complexity | Simpler, but can lead to tangled history | More complex, but results in cleaner history |
Usage | Shared/public branches, where full history is important | Private/feature branches, where linear history is preferred |
Risk | Safe on shared branches | Risky on shared branches (rewrites history) |
Q.21 What is Git bisect? How can you use it to find a specific commit that introduced a bug?
Git Bisect: Automated Binary Search for Finding the Commit that Introduced a Bug
Git bisect is a powerful command in Git that allows you to perform an automated binary search through the commit history to find the specific commit that introduced a bug or issue in the codebase. It is a valuable tool for efficiently identifying the commit responsible for a regression or problem, making it easier to pinpoint and fix the issue.
How Git Bisect Works:
Git bisect uses a binary search algorithm to traverse the commit history and identify the “bad” commit where the bug first appeared. It does this by marking specific commits as “good” (known to be bug-free) and “bad” (known to have the bug). The search space is divided in half with each iteration, quickly narrowing down the range of commits to examine.
Using Git Bisect to Find a Bug:
- Identify a Known Good and Bad Commit: First, you need to identify a commit where the bug was not present (known as a good commit) and another commit where the bug is present (known as a bad commit).
- Start the Bisecting Process: Use the following command to start the bisect process and specify the good and bad commits:
git bisect start git bisect bad <bad_commit> git bisect good <good_commit>
- Testing Each Commit: Git will now automatically check out a commit in between the good and bad commits. You should test the code at each of these commits to determine if the bug is present or not.
- Marking the Commit as Good or Bad: After testing a commit, you need to mark it as either good or bad using the appropriate bisect command:
- If the commit is good (no bug):
git bisect good
- If the commit is bad (bug present):
git bisect bad
- If the commit is good (no bug):
- Iterative Binary Search: Git bisect will continue the iterative binary search, selecting commits in the middle of the remaining range, and having you test and mark them until it identifies the exact commit that introduced the bug.
- Finding the Culprit Commit: Once Git bisect identifies the commit where the bug was introduced, it will output the hash of that commit. You can then review the code changes in that commit to understand what caused the bug.
- Completing the Bisect Process: To finish the bisect process, use the following command:
git bisect reset
Benefits of Git Bisect:
- Git bisect automates the process of finding the problematic commit, saving significant time and effort compared to manual investigation.
- It is efficient for large codebases with extensive commit histories, as it performs a binary search and quickly narrows down the search space.
- Git bisect provides a systematic approach to regression testing, helping to identify and fix issues early in the development process.
Q.22 What is Git submodule? How can you add and update submodules in your Git repository?
Git Submodule: Integrating External Repositories within a Git Repository
Git submodule is a feature in Git that allows you to include another Git repository as a subdirectory within your main Git repository. This enables you to incorporate external code or dependencies into your project while keeping them isolated in their own separate repositories. Submodules are useful when you want to use external libraries or components that are actively maintained in their own Git repositories.
Adding a Git Submodule:
To add a submodule to your Git repository, use the git submodule add
command followed by the URL of the external repository and the path where you want the submodule to be located in your project.
- Add a submodule:
git submodule add <repository_url> <path_to_submodule_directory>
2. Commit the changes to the main repository to save the submodule information.
Updating a Git Submodule:
When you work with submodules, you need to update them independently from the main repository to get the latest changes from the submodule’s remote repository.
- Fetch the latest changes from the remote submodule repository:
git submodule update --remote
- After fetching the changes, you can commit the updated submodule reference in the main repository to record the new commit hash of the submodule.
Cloning a Repository with Submodules:
When cloning a repository that contains submodules, you need to use the --recursive
option to ensure that the submodules are initialized and fetched along with the main repository.
- Clone a repository with submodules:
git clone --recursive <repository_url>
Pulling Changes in Submodule:
To update the submodules to the latest commit in their respective remote repositories, you can use the following command:
- Pull changes in submodules:
git submodule update --remote --merge
Removing a Submodule:
To remove a submodule from your main repository, you need to follow these steps:
- Deinitialize the submodule (removes it from the gitmodules file and the .git/config file):
git submodule deinit <path_to_submodule_directory>
2. Remove the submodule’s directory from the working tree and the index:
git rm <path_to_submodule_directory>
3. Remove any remaining submodule information from the .git/modules directory:
rm -rf .git/modules/<path_to_submodule_directory>
4. Commit the changes to finalize the removal.
Q.23 What is Git filter-branch? How can you use it to rewrite the history of a Git repository?
Git Filter-Branch: Rewriting Git Repository History
Git filter-branch is a powerful and advanced command in Git that allows you to rewrite the entire history of a Git repository. It provides a way to apply various filters to commits, such as modifying commit messages, filtering specific files or directories, or even completely removing certain commits. Git filter-branch is a specialized tool and should be used with caution, especially in shared repositories, as it alters the commit history and can cause conflicts for other developers.
Using Git Filter-Branch to Rewrite Repository History:
The git filter-branch
command is generally used to perform complex history rewriting operations. Here is an overview of how to use it:
- Backup Your Repository: Before running any history-rewriting commands, it’s crucial to back up your repository to prevent accidental data loss.
- Define a Filter: You can use various filters with
git filter-branch
to perform the desired history rewriting. Some common filters include:-msg-filter <command>
: Modify commit messages.-tree-filter <command>
: Apply changes to the repository’s file tree.-index-filter <command>
: Modify the index (staging area).-commit-filter <command>
: Create entirely new commits or skip certain commits.
- Applying Filters: After defining the desired filter, you run the
git filter-branch
command with the specified filter. For example, to change commit messages, use:
git filter-branch --msg-filter 'sed "s/old_text/new_text/"' -- --all
4. Completing the Filter-Branch Operation: After running git filter-branch
, Git creates new commits with the applied filters. You will end up with a completely rewritten history.
5. Handling Refs and Tags: Keep in mind that git filter-branch
rewrites the commit history and creates new commit IDs for each altered commit. If you have references (branches or tags) that point to the old commits, you may need to update them manually to point to the new commits after the filter-branch operation.
Considerations when using Git Filter-Branch:
- Impact on Collaborators: As history rewriting changes commit hashes, it can cause issues for collaborators who have already cloned the repository. Collaboration with others should be carefully managed to avoid conflicts and confusion.
- Backup and Test: Before applying
git filter-branch
, make sure to back up your repository, and test the filtering on a separate clone or branch to verify the desired outcomes. - Use with Caution: Git filter-branch is a powerful tool and should be used judiciously. It is generally recommended for situations where the history rewriting is essential and not for everyday use.
Alternatives to Git Filter-Branch:
For simpler history rewriting tasks, consider using the git commit --amend
, git rebase
, or git cherry-pick
commands, as they provide more straightforward and less intrusive ways to modify the commit history.
Q.24 What is Git reflog? How can you use it to recover lost commits or branches?
Git Reflog: Managing and Recovering Lost Commits or Branches
Git reflog (short for reference log) is a useful command in Git that records the history of references (e.g., branches, HEAD, stash, etc.) in your repository, including when they were updated or moved. It acts as a safety net, allowing you to recover lost commits or branches even if they are no longer visible in the regular commit history.
Viewing Git Reflog:
To view the reflog, use the following command:
git reflog
This will display a list of recent reference updates along with their corresponding commit hashes and actions.
Recovering Lost Commits or Branches:
Git reflog is helpful in recovering lost or accidentally deleted commits, branches, or other references. Here’s how you can use it to recover them:
- Find the Lost Commit or Branch: Use
git reflog
to find the commit or branch that you want to recover. Look for the commit or branch entry in the reflog and note down its commit hash. - Create a New Branch (Optional): If you want to recover a lost branch, first create a new branch at the commit you want to recover. This will provide a reference to the recovered commit.
git branch <branch_name> <commit_hash>
3. Recover Lost Commit: If you want to recover a lost commit, you can directly create a new branch or tag at the commit’s hash.
git branch <branch_name> <commit_hash>
or
git tag <tag_name> <commit_hash>
- Checkout the Recovered Commit or Branch: After creating the new branch or tag, you can checkout that branch or tag to access the recovered commit or branch.
git checkout <branch_name>
Preventing Data Loss:
While Git reflog is a useful tool for recovering lost data, it’s essential to take preventative measures to avoid accidental data loss in the first place:
- Regular Commits: Commit your changes frequently to ensure that important work is saved and can be easily recovered if needed.
- Branch Protection: If you are working with a shared repository, consider setting up branch protection to prevent accidental deletions of branches.
- Branch Renaming: Instead of deleting branches, consider renaming them with descriptive names to keep a record of historical branches.
Q.25 What is Git cherry-pick? How can you use it to apply a specific commit to another branch?
Git Cherry-Pick: Applying Specific Commits to Another Branch
Git cherry-pick is a command in the Git version control system that allows you to apply the changes introduced by specific commits to a different branch. It enables you to select individual commits from one branch and “cherry-pick” them onto another branch, incorporating only the desired changes without merging the entire branch history.
Using Git Cherry-Pick to Apply a Specific Commit to Another Branch:
- Identify the Target Commit: First, identify the commit that you want to apply to another branch. You can find the commit hash by using
git log
or other Git history inspection commands. - Switch to the Target Branch: Make sure you are on the branch where you want to apply the specific commit. If not, switch to that branch using
git checkout
.
git checkout <target_branch>
- Cherry-Pick the Commit: Use the
git cherry-pick
command followed by the commit hash of the specific commit you want to apply to the target branch. git cherry-pick <commit_hash>
Git will apply the changes introduced by the selected commit onto the current branch.- Resolve Conflicts (if any): If there are any conflicts between the changes introduced by the cherry-picked commit and the existing code on the target branch, Git will prompt you to resolve the conflicts manually. You need to edit the affected files, resolve the conflicts, and commit the changes.
- Commit the Cherry-Picked Changes: After resolving conflicts (if any), create a new commit to record the cherry-picked changes on the target branch.
git commit
Optionally, you can usegit cherry-pick -e
to edit the commit message during the cherry-pick process.
Benefits of Git Cherry-Pick:
- Git cherry-pick allows you to select and apply specific changes from one branch to another, making it useful for integrating bug fixes or specific features across branches.
- It keeps the commit history clean and focused, as it only introduces the changes from the cherry-picked commit without bringing in the entire commit history from the source branch.
Note:
- When cherry-picking commits, be cautious about potential conflicts or unintended changes that might arise from applying isolated changes to a different context.
- Cherry-picking should be used for specific cases where you need to transfer selected changes between branches. For regular feature integration, merging or
Q.26 What is Git blame? How can you use it to find out who made a specific change in a file?
git blame
is a Git command that allows you to determine who last modified each line of a file and when the modification was made. It’s particularly useful for tracking down the author and commit information for specific changes within a file. This can be helpful for understanding the context of changes, identifying who made certain modifications, and investigating the evolution of code.
Here’s how you can use git blame
to find out who made a specific change in a file:
git blame <file_path>
Replace <file_path>
with the path to the file you’re interested in. When you run this command, Git will display the content of the file along with the commit information for each line. Each line will be annotated with the commit hash, the author’s name, the date of the commit, and the line content.
For example:
^7a3e506 (John Doe 2021-06-15 10:30:42 +0300 1) This is the first line of the file.
^7a3e506 (John Doe 2021-06-15 10:30:42 +0300 2) This is the second line of the file.
^9b18723 (Alice Smith 2021-06-16 14:20:18 +0300 3) This line was added by Alice.
^7a3e506 (John Doe 2021-06-15 10:30:42 +0300 4) This is the fourth line of the file.
In this example, you can see that line 3 was added by Alice in a commit identified by the hash 9b18723
, and the commit was made on June 16th, 2021, by Alice Smith.
git blame
is a helpful tool for understanding the history of changes in a file and attributing specific modifications to their authors. It can be particularly useful in collaborative projects to see who introduced certain changes, which can aid in code reviews, bug tracking, and communication among team members.
Q.27 What is Git revert? How does it differ from Git reset?
git revert
and git reset
are both Git commands used to manage and manipulate your commit history, but they serve different purposes and have different effects. Let’s explore what each command does and how they differ:
Git Revert:git revert
is used to create a new commit that undoes the changes made in a specific commit or a range of commits. It essentially adds new commits that reverse the changes introduced by the specified commits, while preserving the commit history. This is a safe way to undo changes without rewriting history, which is important in shared or public repositories.
Syntax:
git revert <commit>
Here, <commit>
is the hash of the commit you want to revert.
Difference:
- Preservation of History: The key distinction between
git revert
andgit reset
is thatgit revert
maintains the commit history intact. It creates new commits to reverse changes while still keeping a record of the original commits. This makes it safe for collaborative environments where others might have based their work on the existing history.
Git Reset:git reset
is used to move the current branch pointer to a specified commit, effectively resetting the state of your repository. Depending on the mode used, it can also affect the staging area and working directory. This command can be powerful but should be used with caution, especially in shared repositories, as it can rewrite history and potentially cause problems for collaborators.
Syntax (soft reset):
git reset --soft <commit>
Here, <commit>
is the commit you want to reset to. This keeps the changes from the commits being reset in the staging area, allowing you to make new commits based on that reset state.
Syntax (hard reset):
git reset --hard <commit>
Here, <commit>
is the commit you want to reset to. This discards all changes from the commits being reset, including staged and unstaged changes.
Difference:
- History Rewrite: The main difference with
git reset
is that it can potentially rewrite history by moving the branch pointer and discarding commits. This can lead to problems if those commits were already pushed and used by other collaborators. It’s generally recommended to avoid hard resets on shared branches.
Q.28 What is Git stash? How can you use it to save changes temporarily without committing them?
git stash
is a command in Git that allows you to save changes you’ve made to your working directory and staging area temporarily, without committing them. This is useful when you’re in the middle of working on a task, but you need to switch to a different branch or handle an urgent fix without committing unfinished changes.
Here’s how you can use git stash
to save changes temporarily:
- Stash Changes: To stash your changes, use the following command:
git stash save "Description of changes"
Replace"Description of changes"
with a brief description of the changes you’re stashing. This will create a stash with the changes in your working directory and the staged changes. - Switch Branch or Handle Urgent Task: After stashing your changes, you can switch to a different branch or handle an urgent task without the incomplete changes interfering.
- Apply Stashed Changes: When you’re ready to continue working on the original task, you can apply the stashed changes back using one of the following methods:
- Apply the Latest Stash:
git stash apply
This applies the most recent stash to your working directory and staging area. The stash remains in the stash list. - Apply a Specific Stash: If you have multiple stashes and want to apply a specific one, you can provide the stash index:
git stash apply stash@{<index>}
Replace<index>
with the index of the stash you want to apply. - Apply and Remove Stash: If you want to remove the stash from the stash list after applying it, use:
git stash pop
- Apply the Latest Stash:
- Drop Stash: If you decide you no longer need the stashed changes, you can remove it from the stash list:
git stash drop stash@{<index>}
Replace<index>
with the index of the stash you want to drop.
Remember that git stash
is a useful tool for temporary storage, but it’s not a substitute for proper commits. It’s best used for short-term situations where committing the changes would be premature. If the changes you stashed turn out to be significant and complete, you should create a proper commit to capture them in your version history.
Q.29 What is Git branching? How can you create and switch between branches?
Git branching is a fundamental feature of Git that enables developers to work on different versions of a project simultaneously, allowing for isolated development, feature implementation, bug fixes, and experimentation without affecting the main codebase. Each branch represents a separate line of development that can have its own commits, changes, and history.
Here’s how you can create and switch between branches in Git:
Creating a New Branch: To create a new branch, you can use the following command:
git branch <branch_name>
Replace <branch_name>
with the desired name for your new branch. This command creates the new branch based on the current commit you’re on. However, you’ll still be on the original branch until you switch to the new branch.
Switching Between Branches: To switch to a different branch, use the git checkout
command:
git checkout <branch_name>
Replace <branch_name>
with the name of the branch you want to switch to. This command moves your HEAD (current position) to the specified branch, and your working directory and staging area will reflect the contents of that branch.
Creating and Switching to a New Branch in One Step: You can also create and switch to a new branch simultaneously using the -b
flag with the git checkout
command:
git checkout -b <new_branch_name>
This is equivalent to running both git branch <new_branch_name>
and git checkout <new_branch_name>
.
Viewing Branches: To see a list of all branches in your repository, including the one you’re currently on, use the git branch
command without any arguments:
git branch
The current branch will be highlighted with an asterisk (*) next to its name.
Remember that branches in Git allow for parallel development, enabling you to work on different features or fixes independently. Regularly merging or rebasing branches back into the main development line (usually the main
or master
branch) keeps your codebase up to date with the latest changes from all contributors.
Q.30 What is Git merging? How can you merge changes from one branch to another?
Git merging is the process of combining changes from one branch into another branch. This is typically done to integrate new features, bug fixes, or other changes from a separate development branch (often a feature branch) into the main branch (usually main
or master
), creating a unified codebase with all the changes.
Here’s how you can merge changes from one branch to another in Git:
- Ensure You’re on the Target Branch: First, make sure you’re on the branch where you want to merge the changes. For example, if you want to merge changes from a feature branch into the main branch, switch to the main branch:
git checkout main
- Merge the Changes: Once you’re on the target branch, use the
git merge
command to merge changes from the source branch (the branch you want to merge from) into the current branch:git merge <source_branch>
Replace<source_branch>
with the name of the branch you want to merge changes from. - Resolve Conflicts (if any): If there are conflicting changes between the branches you’re trying to merge, Git will notify you of these conflicts. You’ll need to manually resolve these conflicts by editing the affected files to include the desired changes. After resolving conflicts, you’ll need to stage the changes and commit them.
- Commit the Merge: After resolving conflicts and making sure everything is as expected, you can commit the merge:
git commit -m "Merge changes from <source_branch>"
This creates a new commit that captures the merged changes in the target branch. - Push the Merged Changes: If you’re working with a remote repository, don’t forget to push the merged changes to the remote to update the repository there as well:
git push origin main
Replacemain
with the name of your target branch.
Merging can sometimes result in what’s called a “fast-forward” or a “merge commit,” depending on the circumstances. A fast-forward occurs when the source branch’s commits can be added directly to the target branch without creating an additional commit. A merge commit is created when Git needs to create a new commit to capture the merged changes, especially if there are multiple commits being merged.
Merging is a fundamental aspect of collaborative development, allowing teams to combine their work into a shared codebase. However, it’s essential to follow best practices, communicate effectively with your team, and perform thorough testing after merging to ensure the stability and quality of your codebase.
Q.31 What is Git commit? How can you create a commit and add a commit message?
A Git commit is a snapshot of the changes you’ve made to your project’s files at a specific point in time. Each commit represents a unit of work and includes information about what changes were made, who made them, and when they were made. Commits are an essential part of Git’s version control system and help track the history of your project.
Here’s how you can create a commit and add a commit message:
- Stage Changes: Before you create a commit, you need to stage the changes you want to include in the commit. Staging means preparing the changes to be included in the upcoming commit. To stage changes, you can use the
git add
command followed by the file names or directory paths of the changes you want to stage:git add <file_name>
Replace<file_name>
with the name of the file you want to stage. You can also usegit add .
to stage all changes in the current directory. - Create a Commit: Once you’ve staged the changes, you can create a commit using the
git commit
command. This command captures the staged changes and saves them as a commit in your Git history.git commit -m "Your commit message here"
Replace"Your commit message here"
with a concise and meaningful description of the changes you’re committing. The commit message should provide enough context for others (and your future self) to understand the purpose of the commit. - View Commit History: You can use the
git log
command to view the commit history of your repository. This will show you a list of commits, including their commit messages, authors, dates, and unique commit hashes.git log
Creating informative commit messages is important for maintaining a clear and understandable history of your project. Good commit messages follow these guidelines:
- Keep it concise: Aim for around 50 characters for the summary part of the message.
- Start with a capital letter: Make the message grammatically correct.
- Use the imperative mood: Write the message as if you’re giving a command (e.g., “Add feature,” “Fix bug,” “Update documentation”).
- Provide details (if necessary): If the commit needs more explanation, add a separate paragraph after the summary.
Example of a well-structured commit message:
Add user authentication feature
This commit introduces a new user authentication system using OAuth2.
It includes the necessary routes, controllers, and database changes
Q.32 What is Git pull? How can you pull changes from a remote repository into your local repository?
git pull
is a Git command used to fetch and integrate changes from a remote repository into your local repository. It’s a combination of two actions: git fetch
, which retrieves the changes from the remote repository, and git merge
, which integrates those changes into your current branch.
Here’s how you can pull changes from a remote repository into your local repository:
- Check Your Current Branch: Make sure you’re on the branch where you want to pull the changes. You can use the
git branch
command to see which branch you’re currently on and a list of available branches:git branch
- Fetch and Merge Changes: To fetch and merge changes from the remote repository, use the
git pull
command:git pull origin <branch_name>
Replace<branch_name>
with the name of the remote branch you want to pull changes from. In most cases, the remote branch name will match the local branch name. For example, to pull changes from the remotemain
branch:git pull origin main
- Resolve Conflicts (if any): If there are conflicts between the changes you’re pulling and the changes you have in your local repository, Git will notify you. You’ll need to resolve these conflicts manually by editing the affected files, marking resolved sections, and then committing the resolved changes.
- Commit and Push Changes: After resolving any conflicts and ensuring the changes are as expected, you can commit the merged changes:
git commit -m "Merge remote changes"
Then, if you’re working in a shared repository, you should push the merged changes back to the remote repository:git push origin <branch_name>
Q.33 What is Git push? How can you push changes from your local repository to a remote repository?
git push
is a Git command that’s used to upload your locally committed changes to a remote repository. It allows you to share your code and collaborate with others by sending your commits to a central repository hosted on a remote server, such as GitHub, GitLab, or Bitbucket.
Here’s how you can push changes from your local repository to a remote repository:
- Check Remote Configuration: Make sure you have a remote repository configured as the destination for your push. You can use the
git remote
command to see the list of configured remote repositories:git remote -v
If you haven’t configured a remote yet, you’ll need to set it up using thegit remote add
command:git remote add origin <remote_repository_url>
Replace<remote_repository_url>
with the URL of the remote repository. - Commit Your Changes: Before pushing, ensure that you’ve committed your changes using the
git commit
command. A push will only send committed changes to the remote repository. - Push Changes: To push your committed changes to the remote repository, use the
git push
command:git push origin <branch_name>
Replace<branch_name>
with the name of the branch you want to push. For example, to push changes from your localmain
branch to the remote repository:git push origin main
If the branch you’re pushing to doesn’t exist on the remote repository, Git will create it. - Authentication and Authorization: Depending on the remote repository hosting service and your authentication setup, you may be prompted to enter your credentials (username and password or a personal access token) to authenticate and authorize the push.
- View Changes on Remote Repository: After a successful push, you can visit the remote repository on the hosting platform (such as GitHub) to see your changes, create pull requests, and collaborate with others.
Q.34 What is Git fetch? How can you fetch changes from a remote repository without merging them?
git fetch
is a Git command used to retrieve changes from a remote repository without automatically merging them into your local branch. It’s a way to bring the latest changes from the remote repository into your local repository, allowing you to see what’s new without immediately incorporating those changes into your working directory or branch.
Here’s how you can fetch changes from a remote repository without merging them:
- Check Remote Configuration: Make sure you have a remote repository configured as the source for your fetch. You can use the
git remote
command to see the list of configured remote repositories:git remote -v
If you haven’t configured a remote yet, you’ll need to set it up using thegit remote add
command:git remote add origin <remote_repository_url>
Replace<remote_repository_url>
with the URL of the remote repository. - Fetch Changes: To fetch changes from the remote repository, use the
git fetch
command:git fetch origin
Replaceorigin
with the name of the remote repository you want to fetch changes from. - View Changes: After fetching, your local repository will have the latest changes from the remote repository, but they won’t be automatically merged or applied to your working directory. You can view the changes using commands like
git log
to see the commit history from the remote branch. - Merge or Rebase (Optional): After fetching, you can choose whether to merge the fetched changes into your current branch using
git merge
, or rebase your current branch onto the updated remote branch usinggit rebase
. These actions allow you to incorporate the fetched changes into your working directory and history. For example, to merge the fetched changes into your current branch:git merge origin/<branch_name>
Replace<branch_name>
with the name of the remote branch you fetched changes from.
Q.35 What is Git clone? How can you clone a remote repository to your local machine?
git clone
is a Git command that allows you to create a copy of a remote repository on your local machine. Cloning a repository provides you with a full copy of all the repository’s files, commit history, and branches, enabling you to start working on the project, contribute, or simply explore the codebase.
Here’s how you can clone a remote repository to your local machine:
- Get the Repository URL: First, you need the URL of the remote repository you want to clone. You can find this URL on platforms like GitHub, GitLab, or Bitbucket. It’s usually provided as a HTTPS or SSH URL.
- Navigate to Your Desired Directory: Choose the directory on your local machine where you want to clone the repository. Open your terminal and navigate to that directory using the
cd
command:cd /path/to/your/desired/directory
- Clone the Repository: Use the
git clone
command followed by the repository URL to initiate the cloning process:git clone <repository_url>
Replace<repository_url>
with the URL of the remote repository. - Navigate into the Cloned Repository: After cloning, you’ll find a new directory with the same name as the repository. Use the
cd
command to navigate into the cloned directory:cd <repository_name>
- Start Working: Now you’re inside the cloned repository and can start working with the code. You can create new branches, make changes, and use Git commands to manage your changes.
By default, Git will clone the main
(or master
) branch of the remote repository. If you want to clone a specific branch, you can use the -b
flag followed by the branch name:
git clone -b <branch_name> <repository_url>
For example, to clone the develop
branch:
git clone -b develop <repository_url>
Q.36 What is Git log? How can you view the commit history of a Git repository?
git log
is a Git command used to view the commit history of a Git repository. It displays a list of commits in reverse chronological order (from the most recent to the oldest) along with relevant information such as the commit hash, author, date, and commit message. This command is invaluable for understanding the project’s development history and tracking the changes that have been made over time.
Here’s how you can use git log
to view the commit history of a Git repository:
- Open a Terminal: Start by opening a terminal on your computer.
- Navigate to the Repository Directory: Use the
cd
command to navigate to the directory of the Git repository you want to view the commit history for:cd /path/to/your/repository
- Run the Git Log Command: Once you’re in the repository directory, simply enter the following command to display the commit history:
git log
This will show you a list of commits in the default format, including the commit hash, author name, date, and commit message. You can scroll through the history using the arrow keys. To exit the log view, press theq
key. If you’re only interested in seeing a limited number of commits, you can use thegit log -n <number>
option, where<number>
is the desired number of commits to display. For example, to view the last 5 commits:git log -n 5
- Customizing the Log Output: Git log provides various options for customizing the output. For instance, you can use the
-oneline
flag to display each commit in a condensed format, showing only the commit hash and the first line of the commit message:git log --oneline
You can explore more formatting options and filters in the official Git documentation.
Q.37 What is Git ignore? How can you ignore certain files or directories in a Git repository?
In Git, the concept of “git ignore” refers to the ability to exclude certain files, directories, or patterns from being tracked by Git. This is particularly useful for preventing files that are generated during development, contain sensitive information, or are not relevant to version control from being included in the repository.
To ignore certain files or directories in a Git repository, you can create a special file named .gitignore
in the root directory of your repository. This file contains patterns that specify which files or directories should be ignored.
Here’s how you can create and use a .gitignore
file:
- Create a
.gitignore
File: Create a file named.gitignore
in the root directory of your repository. You can create this file using your preferred text editor or through the terminal. For example:touch .gitignore
- Edit the
.gitignore
File: Open the.gitignore
file in a text editor and add patterns for the files or directories you want to ignore. Each pattern should be on a separate line. Common examples of patterns in a.gitignore
file include:- File extensions:
.log
,.tmp
- Directories:
build/
,node_modules/
- Specific files:
secrets.txt
- File extensions:
- Save and Commit: Save the changes to the
.gitignore
file and commit it to your repository. Once the.gitignore
file is committed, Git will start ignoring the specified files and directories.
Keep in mind that once a file is ignored, it won’t be tracked by Git, and changes to ignored files won’t show up in the list of modified files when you run commands like git status
. Ignoring files is useful for maintaining a clean and focused version control history.
However, it’s essential to be cautious when using .gitignore
as it’s not suitable for sensitive information or security purposes. Ignored files are still present in the repository’s history and could be accessed by anyone who has access to the repository’s history.
Q.38 What is Git revert? How can you revert a commit and create a new commit to undo changes?
git revert
is a Git command used to create a new commit that effectively undoes the changes introduced by a specific commit or a range of commits. It’s a safe way to undo changes in a version-controlled codebase while preserving the commit history. Instead of removing commits from the history, git revert
adds new commits that reverse the changes made in the specified commit(s).
Here’s how you can use git revert
to undo changes introduced by a commit and create a new commit to record the reversal:
- Identify the Commit to Revert: First, identify the commit you want to revert. You’ll need the commit hash associated with that commit. You can use the
git log
command to view the commit history and find the hash of the commit you want to revert:git log
- Revert the Commit: To revert a commit, use the
git revert
command followed by the commit hash:git revert <commit_hash>
Replace<commit_hash>
with the hash of the commit you want to revert. - Edit the Revert Commit Message (Optional): After running the
git revert
command, a text editor will open to allow you to edit the default commit message. The default message usually indicates that you’re reverting a commit. You can modify this message to provide additional context if needed. - Save and Close the Commit Message: After editing the commit message, save and close the text editor. This will create a new commit that undoes the changes introduced by the specified commit.
- View the Commit History: If you use
git log
again, you’ll see the new revert commit in the history, along with the original commit(s) you reverted.
Keep in mind that git revert
is a valuable tool for undoing changes in a way that maintains a clear history and can be useful for shared repositories and collaboration. However, it doesn’t erase the original commit(s) from the history. Instead, it adds a new commit that undoes those changes.