What is a Palindrome number?
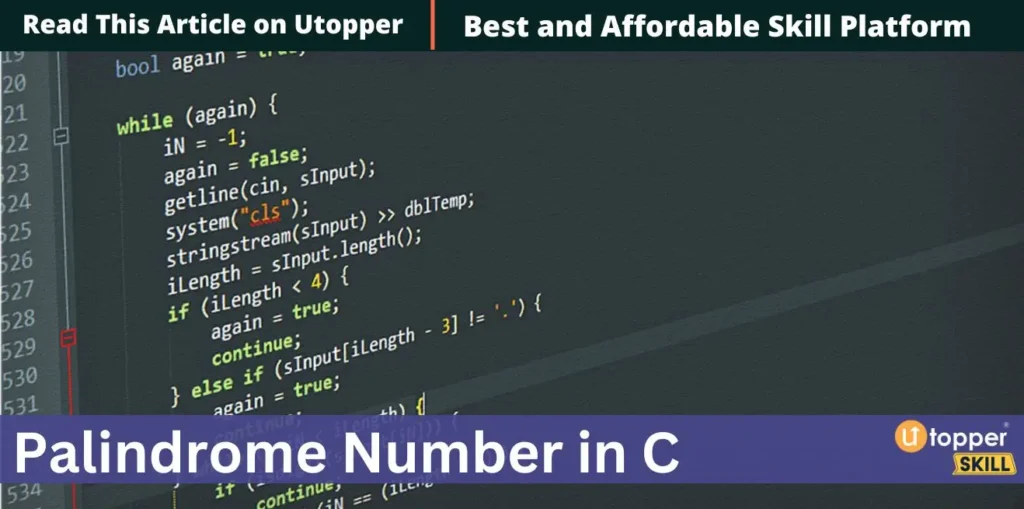
A palindrome number is a number that remains the same when its digits are reversed. For example, the number 121 is a palindrome because it is the same when read forwards or backward. Similarly, the number 1221 is also a palindrome.
Palindrome numbers can be integers or decimal numbers and can be of any length. They can also be negative numbers, but they are not common.
Palindrome numbers are used in many mathematical and computer science problems, such as in algorithms to check if a number is a palindrome or in coding challenges to find the largest palindrome made from the product of two three-digit numbers.
Approach to Check Palindrome Number in C
One approach to check if a number is a palindrome in C is to reverse the digits of the number and compare the reversed number with the original number. This can be done using a while loop and mathematical operations such as modulus and division.
Here is an example of this approach:
- Get the number from the user and store it in a variable (e.g. num).
- Create a variable (e.g. temp) and assign it the value of num.
- Create a variable (e.g. rev) and initialize it to 0.
- Use a while loop to iterate through the digits of temp.
- In each iteration, use the modulus operator (e.g. temp % 10) to get the last digit of temp.
- Multiply rev by 10 and add the last digit obtained from the modulus operation to rev.
- Divide temp by 10 to remove the last digit.
- Repeat steps 4-7 until temp becomes zero.
- After the while loop, check if rev is equal to num.
- If they are equal, the number is a palindrome. If not, it is not a palindrome.
This approach can also be optimized by using a string-based operation to compare the original value with the reverse of the string.
It is important to mention that this approach is not the only way to check if a number is a palindrome in C, and there may be other methods to accomplish this task.
Pseudo code to Check Palindrome Number in C
Here is some sample pseudocode that demonstrates how to check if a number is a palindrome in C:
int num, temp, digit, rev = 0;
printf("Enter a number: ");
scanf("%d", &num);
temp = num;
while (temp != 0) {
digit = temp % 10;
rev = rev * 10 + digit;
temp = temp / 10;
}
if (rev == num) {
printf("%d is a palindrome number", num);
} else {
printf("%d is not a palindrome number", num);
}
In this example, the user is prompted to enter a number, which is then stored in the variable “num.” The variable “temp” is then assigned the value of “num” and is used in the while loop to reverse the digits of the original number. The reversed number is stored in the variable “rev.” After the while loop has finished, the program checks if “rev” is equal to “num.” If they are equal, the original number is a palindrome and the program outputs “num is a palindrome number.” If they are not equal, the original number is not a palindrome and the program outputs “num is not a palindrome number.”
C Program to Check Palindrome Number
#include <stdio.h>
int main() {
int num, temp, digit, rev = 0;
printf("Enter a number: ");
scanf("%d", &num);
temp = num;
while (temp != 0) {
digit = temp % 10;
rev = rev * 10 + digit;
temp = temp / 10;
}
if (rev == num) {
printf("%d is a palindrome number", num);
} else {
printf("%d is not a palindrome number", num);
}
return 0;
}
In this program, the user is prompted to enter a number, which is then stored in the variable “num”. The variable “temp” is then assigned the value of “num” and is used in the while loop to reverse the digits of the original number. The reversed number is stored in the variable “rev”. After the while loop has finished, the program checks if “rev” is equal to “num”. If they are equal, the original number is a palindrome and the program outputs “num is a palindrome number.” If they are not equal, the original number is not a palindrome and the program outputs “num is not a palindrome number.”
In this program, the user input validation is not handled and it will only work with integers.
The output will look something like this:
Enter a number: 121
121 is a palindrome number
Enter a number: -121
-121 is a palindrome number