What is LCM?
LCM means “Least Common Multiple”. This mathematical idea finds the smallest positive integer that is a multiple of two or more integers. In other terms, the LCM of two numbers is the least number divisible by all of them.
12 is the lowest positive integer that is a multiple of both 3 and 4, hence it is the LCM of 3 and 4. 30, the smallest positive integer divisible by all three numbers, is the LCM of 2, 3, and 5.
LCM is significant in number theory, algebra, and calculus. Practical uses include calculating the time it takes two or more objects to accomplish a task or determining the frequency of events at varied intervals.
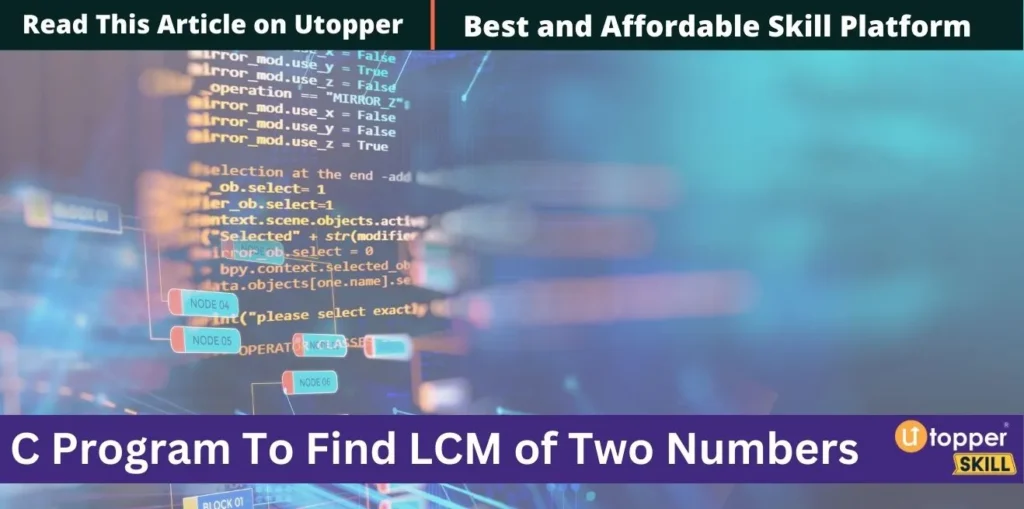
How to find LCM in Maths?
To find the LCM (Least Common Multiple) of two or more numbers in mathematics, you can follow these steps:
- Write down the prime factorization of each number.
- Identify all the unique prime factors from the prime factorizations.
- For each unique prime factor, find the highest power of that factor that appears in any of the prime factorizations.
- Multiply all the prime factors together, each raised to the highest power found in step 3. The result is the LCM.
For example, let’s find the LCM of 12 and 18:
- Prime factorization of 12: 2 x 2 x 3 Prime factorization of 18: 2 x 3 x 3
- Unique prime factors: 2, 3
- Highest power of 2: 2 x 2 = 4 Highest power of 3: 3 x 3 = 9
- LCM = 2^2 x 3^2 = 36
Therefore, the LCM of 12 and 18 is 36.
Pseudo Code to Find LCM of Two Numbers using C Program
// Declare variables to store the two input numbers and the LCM
int num1, num2, lcm;
// Ask the user to input the two numbers
print("Enter the first number: ")
read num1
print("Enter the second number: ")
read num2
// Find the maximum number between num1 and num2
if num1 > num2 then
lcm = num1
else
lcm = num2
// Loop until a common multiple is found
while true do
// Check if lcm is a multiple of both num1 and num2
if lcm % num1 == 0 and lcm % num2 == 0 then
// lcm is the LCM, print it and break out of the loop
print("The LCM of", num1, "and", num2, "is", lcm)
break
end if
// If lcm is not a common multiple, increment it by the maximum input value
lcm = lcm + max(num1, num2)
end while
Approach to Find LCM of Two Numbers using C Program
To find the LCM (Least Common Multiple) of two numbers in C language, you can use the following steps:
- Ask the user to input the two numbers.
- Find the maximum number among the two numbers and assign it to a variable called “max”.
- Create a while loop that will continue until a common multiple is found.
- Inside the while loop, increment the value of “max” by the maximum value of the two numbers.
- Check if both numbers are divisible by the value of “max”. If they are, then “max” is the LCM and you can exit the loop.
- Outside the loop, print the value of “max” as the LCM.
C Program To Find LCM of Two Numbers
#include <stdio.h>
int main() {
int num1, num2, max;
printf("Enter the first number: ");
scanf("%d", &num1);
printf("Enter the second number: ");
scanf("%d", &num2);
// Find the maximum number between num1 and num2
max = (num1 > num2) ? num1 : num2;
// Loop until a common multiple is found
while (1) {
if (max % num1 == 0 && max % num2 == 0) {
printf("The LCM of %d and %d is %d", num1, num2, max);
break;
}
max++;
}
return 0;
}
Output
This program takes two integer inputs from the user and calculates their LCM using a loop. The output is displayed on the console with a message like “The LCM of 6 and 8 is 24”, depending on the user inputs.
Here’s an example output for input values of 6 and 8:
Enter the first number: 6
Enter the second number: 8
The LCM of 6 and 8 is 24