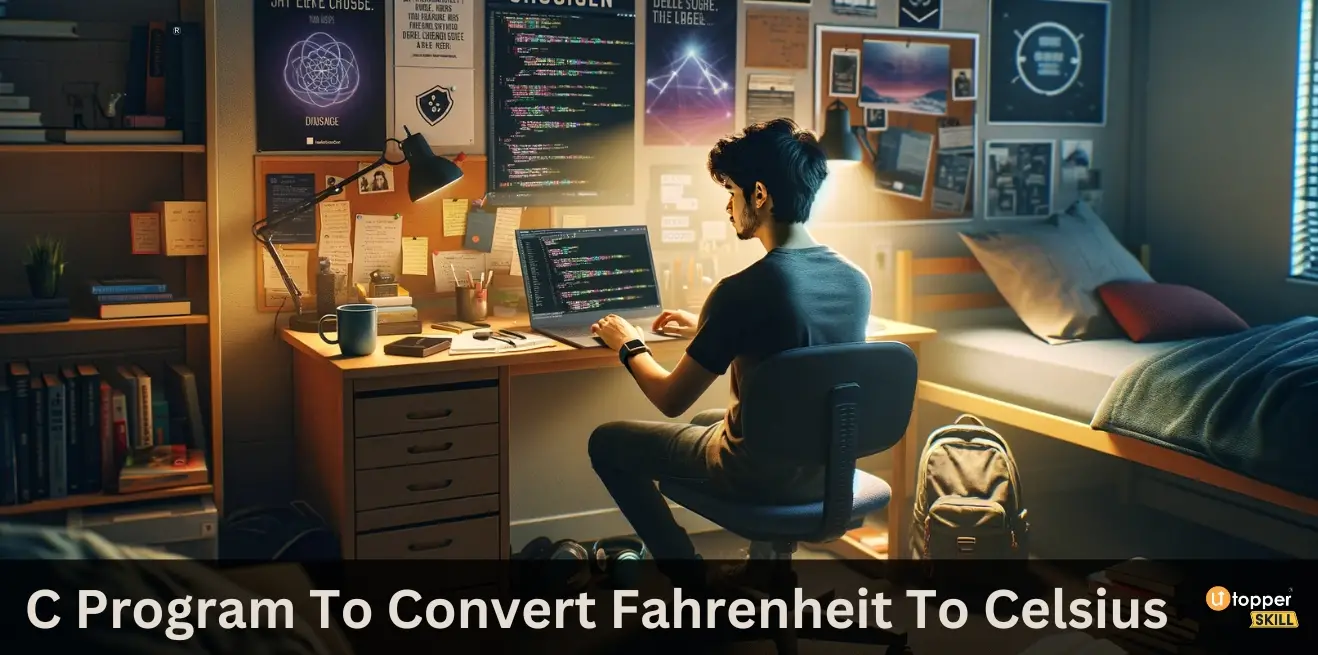
What are Celsius and Fahrenheit?
Common temperature scales are Celsius and Fahrenheit.
Centigrade is a metric temperature scale. In this scale, water’s freezing point is 0°C and its boiling point is 100°C.
The US and a few other countries use Fahrenheit. In this scale, the freezing point of water is 32 degrees Fahrenheit (°F) and the boiling point is 212 °F.
In the US, Fahrenheit is utilised in daily life, while Celsius is used in science and internationally.
In this article, we will share the detailed C Program To Convert Fahrenheit To Celsius, With Approach, Pseudo Code with Output.
What is the approach To Convert Fahrenheit To Celsius in C Programming
To convert a temperature value in Fahrenheit to Celsius in C, you can follow these steps:
- Declare two float variables to hold the Fahrenheit and Celsius temperature values:
float fahrenheit, celsius;
2. Prompt the user to enter a temperature value in Fahrenheit using the printf function:
printf("Enter temperature in Fahrenheit: ");
3. Read the temperature value in Fahrenheit entered by the user using the scanf function:
scanf("%f", &fahrenheit);
4. Convert the Fahrenheit temperature value to Celsius using the following formula:
celsius = (fahrenheit - 32) * 5 / 9;
In this formula, fahrenheit
is the temperature value in Fahrenheit and celsius
is the temperature value in Celsius.
5. Display the converted temperature value in Celsius using the printf function:
printf("%.2f Fahrenheit = %.2f Celsius", fahrenheit, celsius);
In this printf
statement, the %.2f
format specifier is used to display the Fahrenheit and Celsius temperature values with two decimal places.
Pseudo code to Convert Fahrenheit To Celsius in C Programming
// Declare variables
float fahrenheit, celsius
// Prompt user to enter temperature in Fahrenheit
Print "Enter temperature in Fahrenheit: "
Read fahrenheit
// Convert Fahrenheit to Celsius
celsius = (fahrenheit - 32) * 5 / 9
// Display the result in Celsius
Print "Temperature in Celsius: "
Print celsius
This program first declares two float variables fahrenheit
and celsius
. It then prompts the user to enter a temperature in Fahrenheit using the Print
and Read
statements.
Next, the program performs the conversion calculation from Fahrenheit to Celsius and stores the result in the celsius
variable.
C Program To Convert Fahrenheit To Celsius
#include <stdio.h>
int main() {
float fahrenheit, celsius;
printf("Enter temperature in Fahrenheit: ");
scanf("%f", &fahrenheit);
celsius = (fahrenheit - 32) * 5 / 9;
printf("%.2f Fahrenheit = %.2f Celsius", fahrenheit, celsius);
return 0;
}
- This program first declares two float variables:
fahrenheit
andcelsius
. Then, it prompts the user to enter a temperature in Fahrenheit using theprintf
andscanf
functions. - Next, the program performs the conversion calculation from Fahrenheit to Celsius and stores the result in the
celsius
variable. - Finally, the program prints out the original Fahrenheit temperature and the converted Celsius temperature using the
printf
function with formatting specifiers to display two decimal places.
Note : The conversion formula used in this program is (F - 32) * 5/9
, where F
is the temperature in Fahrenheit. This formula converts a Fahrenheit temperature to its Celsius equivalent.
Output
Enter temperature in Fahrenheit: 68
68.00 Fahrenheit = 20.00 Celsius
- In this program, the user is prompted to enter a temperature value in Fahrenheit. The program then reads this input using the
scanf
function and stores it in thefahrenheit
variable. - The program then converts the temperature value in Fahrenheit to Celsius using the formula
celsius = (fahrenheit - 32) * 5 / 9
, and stores the result in thecelsius
variable. - Finally, the program uses the
printf
function to display the converted temperature value in Celsius with two decimal places. The output shows the original temperature value in Fahrenheit and the corresponding temperature value in Celsius.