C++ built-in std::complex class makes complex numbers easy to programme. C++ is a powerful, high-level language that lets programmers write efficient, scalable code for many applications. Scientific computing and engineering often add two complex numbers in C++ using the + operator or a function. C++ is ideal for complex number calculations due to the std::complex class. We’ll write a C++ program to add two complex numbers and discuss the key concepts in this article.
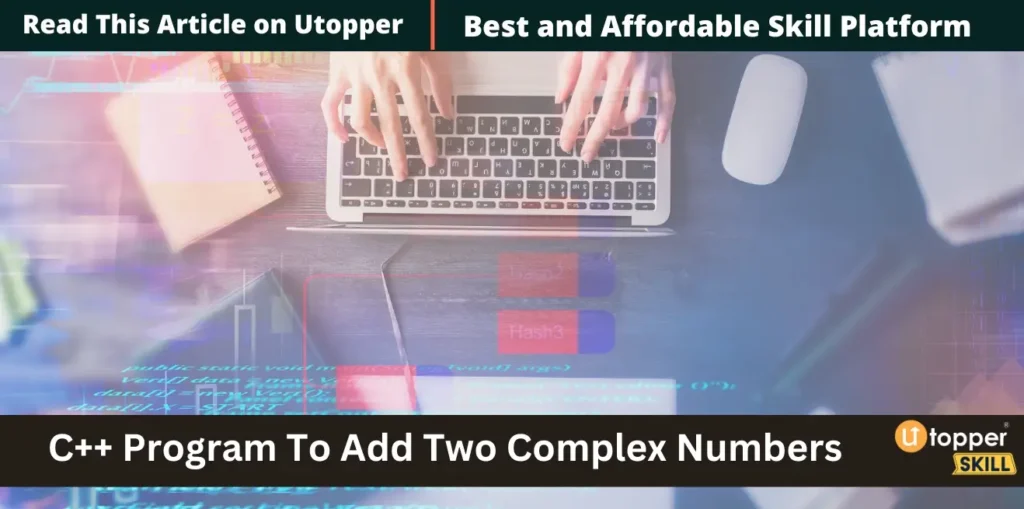
What is a Complex Number?
Complex numbers can be written as a + bi, where a and b are real numbers and I is the imaginary unit, which is the square root of -1. Complex numbers have real and imaginary parts.
On a complex plane, the real axis is horizontal and the imaginary axis is vertical. Real and imaginary parts of a complex number determine its complex plane position.
Math, science, engineering, and other fields use complex numbers. They represent alternating current in electrical engineering and waves in physics. Signal processing, control systems, and other applied mathematics fields use them.
Approach to follow for C++ Program To Add Two Complex Numbers
To write a C++ program to add two complex numbers, we can follow the following approach:
- Define a class for Complex numbers with private data members for the real and imaginary parts.
- Implement a constructor to initialize the real and imaginary parts of the Complex number.
- Implement an operator function to add two Complex numbers using the
+
operator. - Implement a member function to print the Complex number.
- In the
main()
function, create two Complex objects with initial values, add them using the+
operator, and print the sum.
You can Also the C++ Program to Print ASCII Value of Character
Pseudo Code To Add Two Complex Numbers in C++
Here’s a pseudo code to add two complex numbers in C++:
// Define a class for Complex numbers
class Complex {
private:
float real; // Real part of the complex number
float imag; // Imaginary part of the complex number
public:
// Constructor to initialize the real and imaginary parts
Complex(float r = 0, float i = 0) {
real = r;
imag = i;
}
// Operator function to add two Complex numbers
Complex operator +(Complex const &obj) {
Complex res; // Create a new Complex object to store the result
res.real = real + obj.real; // Add the real parts
res.imag = imag + obj.imag; // Add the imaginary parts
return res; // Return the result
}
// Function to print the Complex number
void print() {
cout << real << " + i" << imag << endl; // Print the real and imaginary parts
}
};
// In the main function
int main() {
Complex num1(2.5, 3.5); // Create a Complex object with initial values
Complex num2(1.5, 2.5); // Create another Complex object with initial values
Complex sum = num1 + num2; // Add the two Complex numbers using the + operator
cout << "Sum of two complex numbers is ";
sum.print(); // Print the sum of the two Complex numbers
return 0;
}
C++ Program To Add Two Complex Numbers
To add two complex numbers in C++, you can create a Complex
class to represent a complex number with real and imaginary parts. The Complex
class should have an operator+
function that adds two Complex
objects and returns the result.
#include <iostream>
using namespace std;
// Define a class for Complex numbers
class Complex {
private:
float real; // Real part of the complex number
float imag; // Imaginary part of the complex number
public:
// Constructor to initialize the real and imaginary parts
Complex(float r = 0, float i = 0) {
real = r;
imag = i;
}
// Operator function to add two Complex numbers
Complex operator +(Complex const &obj) {
Complex res; // Create a new Complex object to store the result
res.real = real + obj.real; // Add the real parts
res.imag = imag + obj.imag; // Add the imaginary parts
return res; // Return the result
}
// Function to print the Complex number
void print() {
cout << real << " + i" << imag << endl; // Print the real and imaginary parts
}
};
// In the main function
int main() {
Complex num1(2.5, 3.5); // Create a Complex object with initial values
Complex num2(1.5, 2.5); // Create another Complex object with initial values
Complex sum = num1 + num2; // Add the two Complex numbers using the + operator
cout << "Sum of two complex numbers is ";
sum.print(); // Print the sum of the two Complex numbers
return 0;
}
In this program, we define a class Complex
with two private members real
and imag
to represent a complex number. We also define a constructor to initialize the real and imaginary parts of the complex number, and an operator+
function to add two complex numbers.
In the operator+
function, we create a new Complex
object to store the result of the addition of the two complex numbers. We add the real parts and imaginary parts of the two complex numbers and store the result in the new Complex
object.
In the print
function, we simply print the real and imaginary parts of the complex number.
In the main
function, we create two Complex
objects num1
and num2
with initial values, and add them using the operator+
function. Finally, we print the sum of the two complex numbers.
Output
The output of the above C++ program to add two complex numbers will be:
Sum of two complex numbers is 4 + i6
This is because the program creates two Complex
objects with initial values 2.5 + 3.5i
and 1.5 + 2.5i
, adds them using the operator+
function, and stores the result in the sum
variable. The sum of the two complex numbers is 4 + 6i
. The print
function is then called to print the result, which outputs 4 + i6
.