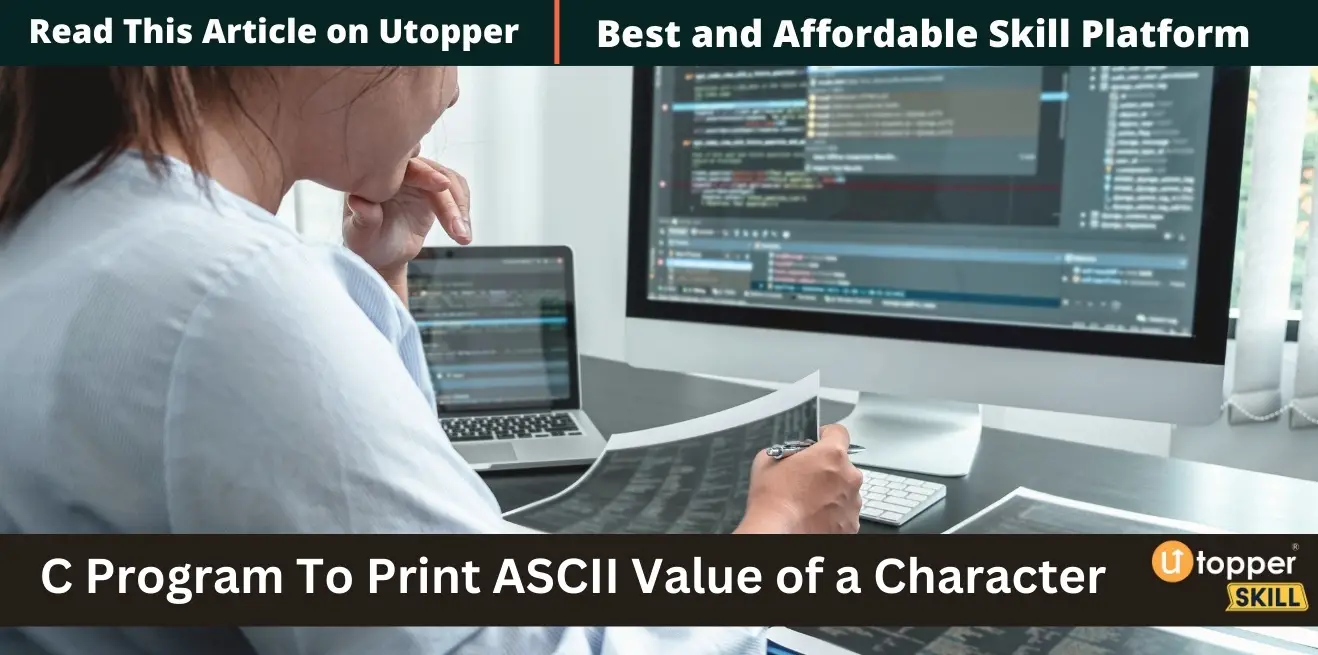
The C++ program to print ASCII value of a character is a simple and fundamental program that is used to obtain the ASCII value of a character input by the user. ASCII stands for American Standard Code for Information Interchange, and it is a character encoding standard used in computing. In this program, the user inputs a character, and the program uses the int()
function to convert the character to its corresponding ASCII value, which is then displayed to the console. This program is useful for beginners who want to learn about character encoding and working with characters in C++.
What is ASCII Value?
ASCII (American Standard Code for Information Interchange) character encoding assigns numeric codes to computer characters.
Each character has a 7-bit numeric code between 0 and 127 (total 128 characters). ASCII codes represent letters, numbers, punctuation marks, and control characters.
The ASCII code for uppercase “A” is 65, lowercase “a” is 97, and digit “0” is 48. ASCII codes allow text representation and manipulation across computer systems and programming languages.
Pseudo code to Print the ASCII Value of a Character
1. Input a character from the user.
2. Convert the character to its ASCII value.
3. Print the ASCII value.
And here’s the pseudocode in more detail:
1. Start
2. Declare a variable character of type character
3. Display "Enter a character: "
4. Read character from the user
5. Declare a variable asciiValue of type integer and initialize it to 0
6. Assign asciiValue the ASCII value of the character (cast character to integer)
7. Display "The ASCII value of <character> is <asciiValue>"
8. End
In this pseudocode, we first prompt the user to enter a character. Then, we declare a variable asciiValue
to store the ASCII value of the character. By casting the character to an integer, we assign its ASCII value to asciiValue
. Finally, we display the ASCII value to the user.
Approach to Print the ASCII Value of a Character
To print the ASCII value of a character in C programming, you can follow this approach:
- Declare a variable of type
char
to store the character. - Read the character from the user.
- Use the
%d
format specifier withprintf
to print the ASCII value of the character.
Here’s the step-by-step approach in more detail:
- Start the program.
- Declare a variable
character
of typechar
. - Display a message asking the user to enter a character.
- Read the character entered by the user and store it in the
character
variable. - Use
printf
to display the ASCII value of the character. Format the output using%d
to print the decimal representation of the ASCII value. - End the program.
By following this approach, you can obtain the ASCII value of a character and display it to the user.
C Program to Print the ASCII Value of a Character
#include <stdio.h>
int main() {
char character;
printf("Enter a character: ");
scanf("%c", &character);
printf("The ASCII value of '%c' is %d.\n", character, character);
return 0;
}
Output:
Enter a character: A
The ASCII value of 'A' is 65.
In this program, we declare a variable character
of type char
to store the input character. Then we prompt the user to enter a character using the printf
function. The %c
format specifier is used to read a character input from the user with the scanf
function, and the &
operator is used to get the address of the character
variable.
After reading the character, we use printf
to display the ASCII value of the character. The %d
format specifier is used to print the decimal representation of the ASCII value.
In the given output example, the user enters the character ‘A’, and the program prints its ASCII value, which is 65.
Output:
Enter a character: x
The ASCII value of 'x' is 120.
In this program, we follow the same approach as before. The user is prompted to enter a character, and the program reads it using scanf
. Then, using printf
, we display the ASCII value of the character by using the %d
format specifier.
In the given output example, the user enters the character ‘x’, and the program prints its ASCII value, which is 120.