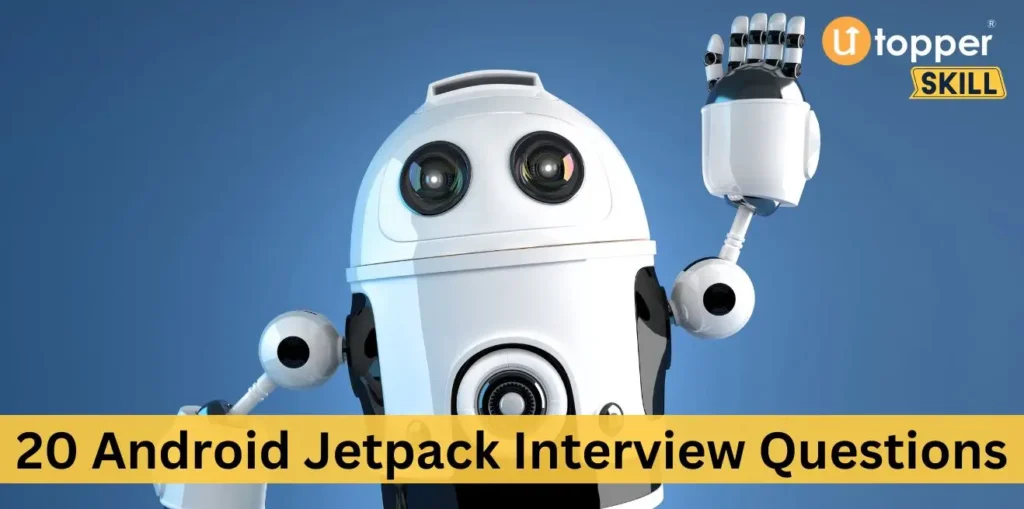
20 android jetpack interview questions
Here are 20 Android Jetpack interview questions that cover a range of topics related to this powerful toolkit.As Android development continues to evolve, Jetpack has emerged as a key component in modern Android app development. It is a set of libraries, tools, and guidance to help developers write high-quality apps more easily. With the growing importance of Jetpack, it’s important to understand the key concepts and features it offers. Whether you’re a seasoned Android developer or just starting out, the android Developer interview questions will help you understand the core components and benefits of Jetpack.
Q.1 What is Android Jetpack and what are its components?
Developers can write high-quality apps faster with Android Jetpack’s frameworks, tools, and coaching. It provides a standard architecture to support current Android development and make app writing and maintenance easier. Android Jetpack components:
- App compat and Android KTX are Android development’s foundation.
- Architecture: ViewModel, LiveData, and Room libraries for building strong, tested, and maintainable programmes.
- UI: libraries like Android KTS, Compose, and Data Binding help create beautiful and responsive user interfaces.
- Behaviour: background task libraries like WorkManager and Paging.
- Developer productivity: Hilt and Benchmark.
These components work together to provide a uniform, up-to-date set of tools and guidelines for modern Android development.
Q.2 What is the purpose of the Android KTX library?
The purpose of the Android KTX library is to make Android development with Kotlin more efficient and enjoyable. It does this by providing:
- Extensions for Android APIs: The Android KTX library provides extensions for common Android APIs, including the Android framework and support libraries, making it easier to work with these APIs in Kotlin.
- Concise and expressive code: By providing extensions for Android APIs, the Android KTX library helps developers write more concise and expressive code, reducing the amount of boilerplate code needed for common tasks.
- Improved code readability: The extensions provided by the Android KTX library help improve the readability of code by making it easier to understand what is happening in the code and how it works.
- Reduced amount of code: By providing extensions for common tasks, the Android KTX library helps developers reduce the amount of code they need to write, making it easier to maintain and update their code in the future.
Q.3 How does the Navigation component help simplify navigation in Android apps?
Android Jetpack’s Navigation component simplifies app navigation by providing a complete solution. Advantages:
Declarative navigation: The Navigation component lets you define an app’s navigation flow in one place without having to manage it in multiple places.
Safe argument passing: The Navigation component makes it easy to pass arguments between destination fragments without errors.
Dynamic navigation: The Navigation component lets developers change an app’s navigation flow at runtime without changing the navigation graph.
Easy deep linking: The Navigation component simplifies deep linking in apps by handling incoming deep links clearly and concisely.
Automated up-and-back navigation: The Navigation component handles up-and-back navigation automatically, making complex app navigation easier.
Q.4 What is the role of the ViewModel in Android Jetpack’s architecture components?
Android apps’ lifecycle-aware data management relies on Android Jetpack’s ViewModel. ViewModel’s main duties:
The ViewModel keeps data alive during configuration changes like screen rotations to prevent data loss.
Decoupling data from the View: The ViewModel separates data from the View’s lifecycle, making it easier to manage and maintain in an app.
Sharing data between fragments: The ViewModel can be shared between fragments, making it easier to share data and manage persistent data.
Unit testing: The ViewModel can be tested separately from the View, making app tests easier to write and maintain.
Q.5 How does LiveData improve data communication between a ViewModel and a View?
Android Jetpack’s LiveData data holder class improves ViewModel-View data communication. LiveData’s data communication advantages are:
Lifecycle-aware: LiveData can observe the lifecycle state of the View and automatically stop sending updates when the View is no longer active, preventing memory leaks and improving app performance.
Background thread-safe: LiveData automatically updates the View on the main thread if data changes on a background thread, ensuring safe and efficient data communication.
Simple and concise code: The LiveData API is simple and concise, making it easy to use and maintain in an app and reducing the boilerplate code needed to implement data communication between a ViewModel and a View.
LiveData makes it easy to update the View when data changes.
Q.6 What are the benefits of using Room as a local database solution?
Room, Android Jetpack’s local database, benefits developers:
Easy to use: Room’s API is simple and intuitive.
Room provides compile-time checks for SQLite queries, reducing runtime errors and making the code more robust.
Type-safe: Room allows type-safe queries and data access to avoid type mismatches.
Testability: Room simplifies local database test writing and maintenance.
Speed: Room optimizes database access for high-performance apps.
Migration support: Room makes it easy to upgrade the database schema as the app evolves.
Room offers Android apps a powerful and flexible local database solution with easy-to-use APIs, compile-time checks, type-safety, testability, speed, and migration support. Room makes apps more robust, maintainable, and testable.
Q.7 Can you explain how the Paging Library works and its use cases?
A feature of Android Jetpack called the Paging Library makes it simple to efficiently and fluidly load and display large amounts of data in an Android app. Large datasets can be handled by the Paging Library, which loads data in manageable “pages” that only contain the information required for screen display.
The Paging Library’s primary use cases include:
Displaying long lists of data, like a list of users, products, or articles, is a common use for the Paging Library. A smaller amount of data is loaded at a time thanks to the library’s chunk loading technique, which lowers the possibility of performance problems and improves the responsiveness of the application.
Large data sources: The Paging Library is made to work with large data sources, like databases, REST APIs, or cloud storage, making it simple to load and efficiently display large amounts of data.
The Paging Library can be used to implement infinite scrolling, which loads new data as the user scrolls down the list rather than delaying the loading of the entire dataset.
Network data: By loading the data in small chunks and displaying it as it becomes available, the Paging Library can be used to effectively load and display data from a network, such as a REST API.
All things considered, the Paging Library offers a strong and adaptable solution for quickly loading and showing large amounts of data in an Android app. Developers can create more responsive, testable, and maintainable apps by utilizing the Paging Library.
Q.8 What is the role of WorkManager in background processing in Android Jetpack?
Android Jetpack’s WorkManager makes background work scheduling and management easy. WorkManager simplifies background processing by providing a consistent and easy-to-use API for scheduling and executing tasks, regardless of whether the app is running.
WorkManager features include:
WorkManager supports Android versions starting with Android 5.0 (Lollipop) and provides a backwards-compatible API.
- Background processing: WorkManager can run tasks in the background even when the app is not running, making it ideal for background tasks.
- Scheduling: WorkManager’s API makes scheduling tasks, such as setting deadlines and conditions, simple.
- Chaining and parallel execution: WorkManager simplifies complex workflows by chaining and parallelizing tasks.
- Battery optimization: WorkManager schedules and executes work to minimize battery usage.
WorkManager is a powerful and flexible background processing solution for Android apps, providing a consistent and easy-to-use API for scheduling and executing tasks whether the app is running or not. WorkManager helps developers write reliable, maintainable apps that run background work efficiently.
Q.9 How does the Android KTX library simplify Android development?
The Android KTX (Kotlin Extensions) library simplifies Android development by making code concise and readable. The library extends Android API classes like Activity, Fragment, and View and Android Jetpack libraries like LiveData and ViewModel.
Android KTX simplifies Android development mainly by:
Concise code: Android KTX extensions make it easier to write concise and readable code, reducing boilerplate code and making it easier to understand.
Kotlin-friendly API: Android KTX provides a Kotlin-friendly API for the Android API and extensions for some Android Jetpack libraries, making them easier to use in Kotlin.
Improved readability: Android KTX’s extensions make code easier to understand and reduce bugs.
Enhanced functionality: Android KTX enhances some Android API classes and Jetpack libraries to make common tasks easier and code more efficient and maintainable.
The Android KTX library helps developers write concise, readable, and maintainable code. The Android KTX library helps Kotlin developers write more efficient and effective Android apps.
Q.10 What is the purpose of the Lifecycle Library in Android Jetpack?
A feature of Android Jetpack called the Lifecycle Library gives developers a way to control how long Android app fragments and activities last. The Lifecycle Library’s main goal is to make handling component lifecycles simpler so that code that is aware of the lifecycle state of the app components and can react to changes in that state can be written more quickly.
The Lifecycle Library’s main attributes and advantages are as follows:
Component lifecycle awareness is made possible by the Lifecycle Library, which gives app components a way to monitor their lifecycle state and react to changes in it. Writing code that can respond to occurrences like the creation, destruction, or interruption of app components is made simpler as a result.
Better component management: The Lifecycle Library offers a way to consistently and dependably manage the lifecycle of app components, making it simpler to write robust, maintainable code.
Better memory management: By ensuring that app components are destroyed when they are no longer required, the Lifecycle Library helps to improve memory management by lowering the possibility of memory leaks and enhancing app performance.
Easier to write code that can react to changes in the lifecycle state of app components thanks to the Lifecycle Library’s straightforward and simple API for handling lifecycle events.
Overall, the handling of component lifecycles in Android apps is made easier thanks to the Lifecycle Library, a crucial part of Android Jetpack. Developers can create more dependable, effective, and maintainable Android apps by utilizing the Lifecycle Library. These apps will be aware of the components’ current lifecycle states and will be able to react to changes in those states.c
Q.11 How does the Android Jetpack Compose library simplify UI development?
A contemporary UI toolkit for Android that makes it simpler to design stunning and responsive user interfaces is the Android Jetpack Compose library. For creating UIs, it offers a declarative and reactive programming model, making it simpler to write UI code that is condensed, readable, and maintainable.
Android Jetpack Compose streamlines UI development primarily in the following ways:
Declarative programming: When using Android Jetpack Compose, the developer specifies how the user interface (UI) should appear, and the library handles rendering. This makes writing clear, concise, and understandable UI code simpler.
Android Jetstate Compose employs reactive programming, which causes the UI to update automatically in response to data changes. In addition to making it simpler to write code that is testable and maintainable, this also makes it simpler to write UI code that is responsive and dynamic.
Simple layout management: Android Jetpack Compose offers a comprehensive collection of UI components that can be combined to easily create sophisticated and complex UIs. Additionally, it offers a simple layout system that makes it easy to align and arrange UI elements on the screen.
Better performance: Android Jetpack Compose is performance-optimized, and it uses cutting-edge methods like incremental updates and composition to speed up and improve efficiency. It is now simpler to design UIs that are quick, responsive, and fluid.
A better developer experience is provided by Android Jetpack Compose, which offers a cutting-edge and user-friendly API that makes writing UI code simple. It also offers tools and libraries that make it simple to debug and test UI code. This makes it simpler to create UIs that are dependable, strong, and of a high caliber.
Overall, by offering an up-to-date, declarative, and reactive programming model for creating UIs in Android, the Android Jetpack Compose library simplifies UI development. Developers can write UIs that are stunning, responsive, and of the highest caliber while writing less code and experiencing fewer headaches by using Android Jetpack Compose.
Q.12 What is the role of the Hilt library in Android Jetpack for dependency injection?
It’s simpler to design stunning and responsive user interfaces with the help of the Android Jetpack Compose library, a modern UI toolkit for Android. In order to make it simpler to write UI code that is condensed, readable, and maintainable, it offers a declarative and reactive programming model for creating UIs.
Android Jetpack Compose mainly makes UI development simpler in the following ways:
Declarative programming: The developer specifies how the user interface (UI) should appear, and the library takes care of rendering it, in the case of Android Jetpack Compose. The writing of clear, concise, and understandable UI code is made simpler as a result.
Reactive programming: The UI of Android Jetstate Compose automatically updates in response to data changes. This makes it simpler to write UI code that is dynamic and responsive, as well as easier to write code that is testable and maintainable.
Simple layout management: The rich collection of composable UI components offered by Android Jetpack Compose makes it simple to build intricate and sophisticated UIs. Assembling and aligning UI elements on the screen is also made simple by the layout system that is offered.
Better performance: Android Jetpack Compose uses cutting-edge methods like incremental updates and composition to make it quicker and more effective. It is performance-optimized. Fast, responsive, and smooth UI design is now made simpler by this.
Improved developer experience: Android Jetpack Compose offers a cutting-edge and user-friendly API that makes writing UI code simple. It also offers tools and libraries that make it simple to debug and test UI code. It is now simpler to create high-quality, dependable user interfaces as a result.
The Android Jetpack Compose library offers a contemporary, declarative, and reactive programming model for creating UIs in Android, which overall simplifies UI development. Developers can create high-quality, responsive, and beautiful user interfaces with Android Jetpack Compose while writing less code and experiencing fewer headaches.
Q.13 Can you give an example of using the Android KTX library to simplify code in an Android app?
Here’s an example of how the Android KTX library can simplify code in an Android app.
Suppose you have an activity that needs to show a Toast message to the user. Here’s what the code would look like without the Android KTX library:
val context = this
val text = "Hello, KTX!"
val duration = Toast.LENGTH_SHORT
val toast = Toast.makeText(context, text, duration)
toast.show()
Now, let’s see how the same code would look with the Android KTX library:
val text = "Hello, KTX!"
toast(text)
The Android KTX library simplifies and reads the code. KTX extension functions and properties simplify Android development tasks like showing a Toast message. The library simplifies writing maintainable and scalable code with a concise syntax.
The Android KTX library has many other extension functions and properties that simplify Android development tasks like working with SharedPreferences and making network requests. Developers can write cleaner, more readable, and easier-to-maintain Android apps with less code and fewer headaches using the Android KTX library.
Q.14 How can you handle configuration changes with the Android Jetpack architecture components?
Android Jetpack architecture components handle configuration changes reliably and efficiently. Configuration changes include device orientation, screen size, language, and other system settings that affect app UI.
ViewModel handles configuration changes. Lifecycle-aware ViewModels hold and manage UI data, surviving configuration changes. The system recreates the Activity after a configuration change, but the ViewModel retains UI data. The Activity then uses ViewModel data to restore the UI.
A simple ViewModel example for Android app configuration changes:
class MyViewModel : ViewModel() {
var name = MutableLiveData<String>()
}
class MainActivity : AppCompatActivity() {
private lateinit var viewModel: MyViewModel
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
viewModel = ViewModelProviders.of(this).get(MyViewModel::class.java)
// Use the data in the ViewModel to update the UI
}
}
In this example, the MainActivity uses ViewModelProviders to get a reference to MyViewModel. The name LiveData object in MyViewModel can update the UI. The system recreates the MainActivity after a configuration change, but the MyViewModel instance retains name data. The MainActivity can then use ViewModel data to restore the UI.
Using the ViewModel to handle configuration changes keeps your app responsive and prevents data loss or duplication. This simplifies app maintenance, scaling, and robustness.
Q.15 What is the role of the Android Test library in testing Android apps?
- JUnit4. Android Test library supports JUnit4, the most popular Java testing framework.
- Activity Testing: The Android Test library has APIs for launching an Activity and checking its UI state.
- Espresso: The Android Test library’s Espresso framework simplifies Android app UI testing. Espresso tests can click buttons, type text into fields, and verify data.
- UI Automator: The Android Test library provides UI Automator, a framework for writing multi-app UI tests. UI Automator tests can access system notifications and settings.
- Test runner: The Android Test library’s test runner runs your tests on an Android device or emulator, collects results, and provides detailed reports
Q.16 How can the Data Binding Library be used to simplify data binding in Android apps?
- Improved readability: The Data Binding Library makes data-binding code easier to read and understand.
- Reduced boilerplate code: The Data Binding Library makes data binding to the UI simpler and easier to maintain.
- Performance: The Data Binding Library optimises data binding using the Android data binding framework, improving performance compared to traditional methods.
- Two-way data binding: The Data Binding Library supports two-way data binding, which automatically updates the data and UI, reducing the amount of code needed to implement this feature.
The Data Binding Library simplifies data binding in Android apps, reduces boilerplate code, and improves performance and readability. Developers can focus on app development instead of data binding because the library simplifies it.
Q.17 What is the purpose of the Android Slices library and how can it be used to improve the user experience?
The Android Jetpack’s Android Slices library lets developers display parts of their app’s UI in other surfaces like Google Search. Slices respond to search queries with rich, interactive content. Search results can include text, images, and actions.
The Android Slices library improves user experience in these ways:
- Slices provide contextual information even outside the app. This helps users find and finish their tasks faster.
- Interactive content: Slices let users act on search results. This simplifies task completion without opening the app.
- Slices help users find app content and features. This can boost the app’s popularity.
- Slices provide a consistent experience across Google Search, Assistant, and Maps. This ensures a smooth app experience regardless of how users access content.
Developers can improve user experience by providing rich, interactive content outside the app using the Android Slices library. The library simplifies app discovery and provides a consistent experience across devices.
Q.18 How can the Android Animation & Transitions library be used to create animations and transitions in Android apps?
- JUnit4. Android Test library supports JUnit4, the most popular Java testing framework.
- Activity Testing: The Android Test library has APIs for launching an Activity and checking its UI state.
- Espresso: The Android Test library’s Espresso framework simplifies Android app UI testing. Espresso tests can click buttons, type text into fields, and verify data.
- UI Automator: The Android Test library provides UI Automator, a framework for writing multi-app UI tests. UI Automator tests can access system notifications and settings.
- Test runner: The Android Test library’s test runner runs your tests on an Android device or emulator, collects results, and provides detailed reports.
Q.19 What are the benefits of using the Android CameraX library for camera development in Android?
- CameraX is compatible with the Camera2 API and provides a higher-level interface for accessing the camera’s capabilities, making camera apps easier to build.
- Easy to use: CameraX’s API abstracts camera hardware and simplifies camera-based application development.
- Consistent behaviour across devices: CameraX ensures that the same code works on Android devices with different camera hardware.
- Improved image quality: CameraX’s automatic scene-based image adjustments reduce the effort needed to achieve the desired image quality.
- Use cases: CameraX includes pre-built use cases for common camera scenarios like taking photos and videos, previewing images, and applying filters.
- CameraX works well with other Jetpack libraries, making it easy to integrate camera functionality with other parts of your app, such as data or user interfaces.
Q.20 Can you explain the role of the Android Benchmark library in performance testing and optimization for Android apps?
Android Jetpack’s Android Benchmark library helps developers test and optimise Android app performance. The library includes tools and APIs for measuring Android app performance:
- CPU performance: The library has APIs for measuring CPU performance, such as code execution time.
- Memory usage: The library tracks object creation and app memory usage via APIs.
- I/O performance: The library has APIs for measuring disc and network I/O performance.
- User interface performance: The library provides APIs for measuring the time it takes to render a view or animation.