Approach to Print Alphabet Right triangle in C language
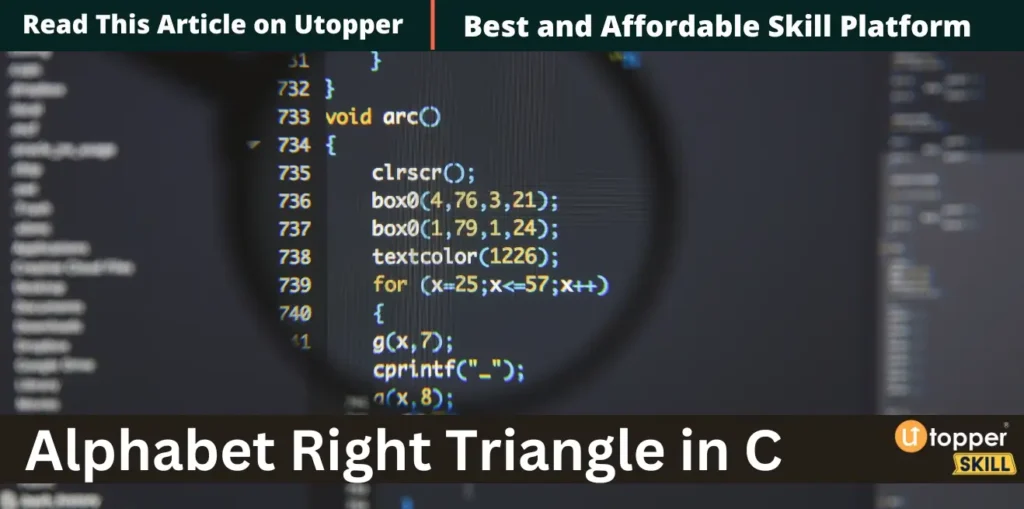
Basic steps for printing an alphabet triangle in C language:
- Define a variable to determine the number of rows in the triangle.
- Use nested for loops to iterate through the rows and columns of the triangle. The outer loop should iterate through the rows and the inner loop should iterate through the columns within each row.
- In each iteration of the inner loop, calculate the letter to be printed based on the current row and column indices. For example, you can initialize a variable to the starting letter of the triangle (such as ‘A’) and increment it with each iteration of the inner loop.
- Use the
printf
function to print the letter at the current position in the triangle. - Print a new line after each row using
printf("\n")
to create the triangle shape. - Another approach is to use recursion where you start with the base case and keep calling the recursion until you reach the uppermost layer of the triangle where you print the letter.
- You can also add some logic to print the triangle with different starting letter or different pattern.
- Finally, test the program and make sure it produces the desired output.
Pseudo code to print Alphabet Right Triangle in C.
Pseudocode for printing an alphabet triangle in C using For Loop .
// Define the number of rows for the triangle
int rows = 5;
// Use a nested loop to iterate through the rows and columns
for (int i = 0; i < rows; i++) {
for (int j = 0; j <= i; j++) {
// Calculate the letter to be printed
char letter = 'A' + j;
// Print the letter at the current position in the triangle
printf("%c ", letter);
}
// Print a new line to create the triangle shape
printf("\n");
}
This code uses nested for loops to first iterate through the rows (i) of the triangle and then the columns (j) within each row. The variable ‘letter’ is initialized to ‘A’ and is incremented with each iteration of the inner loop. The printf
function is then used to print each letter on the same line for each row. Finally, a new line is printed after each row using printf("\n")
to create the triangle shape.
Pseudo code to print alphabet triangle using recursion.
void print_triangle(int n, char c) {
if (n == 0) {
return;
}
print_triangle(n - 1, c);
for (int i = 0; i < n; i++) {
printf("%c ", c + i);
}
printf("\n");
}
C program to print alphabet triangle using for Loop
C program that uses a nested for loop to print an alphabet triangle:
#include <stdio.h>
int main() {
int rows = 5;
for (int i = 0; i < rows; i++) {
for (int j = 0; j <= i; j++) {
char letter = 'A' + j;
printf("%c ", letter);
}
printf("\n");
}
return 0;
}
This program defines a variable rows
to determine the number of rows in the triangle, and uses nested for loops to iterate through the rows and columns of the triangle. The variable letter
is initialized to ‘A’ and is incremented with each iteration of the inner loop. The printf
function is then used to print each letter on the same line for each row. Finally, a new line is printed after each row using printf("\n")
to create the triangle shape.
Output :
A
A B
A B C
A B C D
A B C D E
C program to print alphabet triangle using Recursion
#include <stdio.h>
void print_triangle(int n, char c) {
if (n == 0) {
return;
}
print_triangle(n - 1, c);
for (int i = 0; i < n; i++) {
printf("%c ", c + i);
}
printf("\n");
}
int main() {
int rows = 5;
char start = 'A';
print_triangle(rows, start);
return 0;
}
This program uses recursion to print the triangle, it accepts two parameters, number of rows and the starting letter, it calls the function print_triangle
with the given parameters and it prints the triangle.
You can also check the Other C Programs :