Variables in C Programming
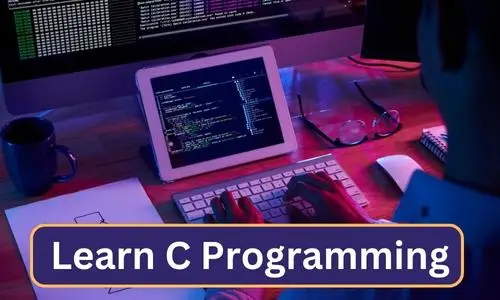
Page Index
In C programming, a variable is a named location in the computer’s memory that stores a value of a particular type, such as integer, float, or character. A variable allows a programmer to store and manipulate data within a program.
Here is an example of how to declare a variable in C programming:
int x; // declares an integer variable named x
float y; // declares a floating-point variable named y
char c; // declares a character variable named c
In the example above, int
declares an integer variable, float
declares a floating-point variable, and char
declares a character variable. The variable names (x
, y
, and c
) are user-defined, and can be any valid identifier.
To assign a value to a variable, use the assignment operator =
:
x = 10; // assigns the value 10 to x
y = 3.14; // assigns the value 3.14 to y
c = 'a'; // assigns the character 'a' to c
In the example above, x
is assigned the value 10
, y
is assigned the value 3.14
, and c
is assigned the character 'a'
.
Variables can also be initialized at the time of declaration, like this:
int x = 10; // declares and initializes x with the value 10
float y = 3.14; // declares and initializes y with the value 3.14
char c = 'a'; // declares and initializes c with the character 'a'
In this example, x is declared and initialized with the value 10, y is declared and initialized with the value 3.14, and c is declared and initialized with the character ‘a’.
Rules of Defining a Variable in c
- Variable names must start with a letter (a-z or A-Z) or an underscore (_), and can be followed by any combination of letters, digits (0-9), or underscores.
int x;
float my_variable;
char _ch1;
2. Variable names cannot be a keyword or reserved word in C programming. Examples of keywords include int, float, char, if, else, for, while, do, switch, and case.
// Invalid variable names
int if;
float for;
char switch;
3. Variable names are case sensitive. This means that x, X, and x1 are three different variables.
int x = 10;
int X = 20;
int x1 = 30;
4. Variable names should be descriptive and meaningful. This makes it easier for other programmers to understand the purpose of the variable.
float temperature_celsius;
int number_of_students;
char first_initial;
5. Variable names should not exceed 31 characters in length. This is because some C compilers may have a limit on the length of variable names.
// Valid variable names
int number_of_students_in_class;
char last_name_initial;
// Invalid variable names (exceeds 31 characters)
float this_is_a_very_long_variable_name_that_should_not_be_used;
Types of Variables in C Programming
In C programming, there are several types of variables that can be used to store data of different types and sizes. The following are the main types of variables in C:
- Integer Variables: These variables are used to store whole numbers (integers) without decimal places. Examples include
int
,short
, andlong
data types.
int x = 10;
short y = 5;
long z = 1000000L;
2. Floating-Point Variables: These variables are used to store numbers with decimal places. Examples include float and double data types.
float a = 3.14;
double b = 6.022e23;
3. Character Variables: These variables are used to store single characters. They are declared using the char data type.
char c = 'A';
4. Boolean Variables: These variables are used to store true or false values. They are declared using the bool data type.
bool is_raining = true;
5. Array Variables: These variables are used to store a collection of values of the same data type. They are declared by specifying the data type and the number of elements in the array.
int numbers[5] = {1, 2, 3, 4, 5};
6. Pointer Variables: These variables are used to store the memory addresses of other variables. They are declared using the symbol.
int x = 10;
int *ptr = &x;
7. Enum Variables: These variables are used to create user-defined data types that represent a set of named constants.
enum colors {RED, BLUE, GREEN};
enum colors favorite_color = BLUE;
Types of Variables Based on Scope
In C programming, there are several types of variables based on their scope and lifetime. The main types of variables are:
- Local Variables: These variables are declared inside a function or a block of code and can only be accessed within that function or block. They are created when the function or block is called and destroyed when it completes. Local variables are not visible outside the function or block in which they are declared.
void my_function() {
int x = 10; // local variable
// code block
}
2. Global Variables: These variables are declared outside any function or block and can be accessed from anywhere in the program. They are created when the program starts and destroyed when the program ends. Global variables are visible to all functions in the program.
int x = 10; // global variable
void my_function() {
// code block
}
3. Static Variables: These variables are declared inside a function or block and retain their value between function calls. They are created when the function or block is first called and destroyed when the program ends. Static variables are not visible outside the function or block in which they are declared.
void my_function() {
static int x = 0; // static variable
x++;
// code block
}
4. Automatic Variables: These variables are similar to local variables but are declared with the auto keyword. They are created when the function or block is called and destroyed when it completes. Automatic variables are not visible outside the function or block in which they are declared.
void my_function() {
auto int x = 10; // automatic variable
// code block
}
5. Register Variables: These variables are declared with the register keyword and are stored in the computer’s register instead of memory. They are used to speed up program execution by reducing the time it takes to access memory. Register variables are only used for variables that are frequently accessed in the program.
void my_function() {
register int x = 10; // register variable
// code block
}