Printf and Scanf in C Programming
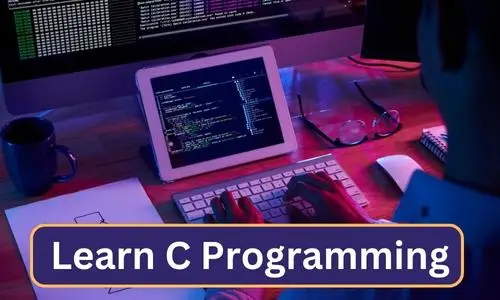
Page Index
Printf and Scanf in C Programming
Printf
and Scanf
are two functions in the C programming language that are used for input/output operations.
printf
is used to output data to the console or other output devices. It is used to display text and values on the screen. The function has a format string that describes the output, followed by a comma-separated list of values to be displayed. The format string contains format specifiers that specify the data type to be displayed, such as integers, floating-point numbers, characters, and strings.
For example, the following code will display the message “Hello, world!” on the screen:
#include <stdio.h>
int main() {
printf("Hello, world!\n");
return 0;
}
scanf
, on the other hand, is used to read input data from the user. It reads data from the standard input device, such as the keyboard, and stores the values in the specified variables. Like printf
, it has a format string that specifies the type of data to be read and the location to store it.
For example, the following code will read two integer values from the user and store them in the variables a
and b
:
#include <stdio.h>
int main() {
int a, b;
printf("Enter two integer values: ");
scanf("%d%d", &a, &b);
printf("You entered: %d and %d\n", a, b);
return 0;
}
In the code above, the format string %d%d
specifies that two integer values should be read from the user, and the &
operator is used to obtain the memory addresses of the variables a
and b
so that the input values can be stored in them.
Both printf
and scanf
are part of the standard C library and are included in the stdio.h
header file.
Syntax of Printf
The syntax of printf function in C programming language is:
printf("format string", argument1, argument2, ...);
where format string
is a string that specifies the output format and argument1
, argument2
, and so on are the values to be displayed.
The format string can contain plain text characters and format specifiers. The format specifiers start with the %
character and are followed by a character that specifies the data type of the corresponding argument. The most commonly used format specifiers are:
%d
: for integers%f
: for floating-point numbers%c
: for characters%s
: for strings%p
: for memory addresses%e
or%E
: for scientific notation (exponential format)%u
: for unsigned integers
For example, to display an integer value on the screen, you can use the following printf
statement:
int x = 10;
printf("The value of x is %d", x);
This statement will display the message “The value of x is 10” on the screen, where %d
is replaced by the value of x
.
You can also use multiple format specifiers in the same format string, separated by commas, to display multiple values on the screen:
int x = 10, y = 20;
printf("The value of x is %d and the value of y is %d", x, y);
This statement will display the message “The value of x is 10 and the value of y is 20” on the screen.
Syntax of Scanf
The syntax of the scanf
function in C programming language is:
scanf("format string", &variable1, &variable2, ...);
where format string
is a string that specifies the format of the input, and &variable1
, &variable2
, and so on are the memory addresses of the variables where the input values will be stored.
The format string can contain format specifiers that specify the type of input expected from the user. The most commonly used format specifiers are:
%d
: for integers%f
: for floating-point numbers%c
: for characters%s
: for strings%p
: for memory addresses%e
or%E
: for scientific notation (exponential format)%u
: for unsigned integers
For example, to read an integer value from the user and store it in the variable x
, you can use the following scanf
statement:
int x;
scanf("%d", &x);
This statement will wait for the user to input an integer value and store it in the variable x
.
You can also use multiple format specifiers in the same format string, separated by spaces or other non-digit characters, to read multiple values from the user:
int x, y;
scanf("%d %d", &x, &y);
This statement will wait for the user to input two integer values separated by whitespace and store them in the variables x
and y
.
Note that the &
operator is used to obtain the memory address of the variable, which is necessary for scanf
to write the input value to the correct location in memory.
Examples :
C program showing us of scanf and print
#include <stdio.h>
int main() {
int x, y, sum;
printf("Enter two integers: ");
scanf("%d %d", &x, &y);
sum = x + y;
printf("The sum of %d and %d is %d\n", x, y, sum);
return 0;
}
In this program, we declare three integer variables: x
, y
, and sum
. We then prompt the user to enter two integers by displaying the message “Enter two integers: ” using printf
. We read the two integers from the user using scanf
and store them in the variables x
and y
. We then compute their sum and store it in the variable sum
. Finally, we use printf
to display the message “The sum of x and y is sum”, where x
, y
, and sum
are replaced by their respective values.
For example, if the user enters the values 10 and 20, the program will output the following message:
Output :
Enter two integers: 10 20
The sum of 10 and 20 is 30
Note that we use the %d format specifier to read and display integers, and we use the & operator to pass the memory address of the variables to scanf for input. We also use the \n character in the printf statement to print a newline character after the message.
c program to find cube of any number showing use of scanf and printf
#include <stdio.h>
int main() {
int num, cube;
printf("Enter an integer: ");
scanf("%d", &num);
cube = num * num * num;
printf("The cube of %d is %d\n", num, cube);
return 0;
}
In this program, we declare two integer variables: num
and cube
. We then prompt the user to enter an integer by displaying the message “Enter an integer: ” using printf
. We read the integer from the user using scanf
and store it in the variable num
. We then compute its cube by multiplying it with itself three times and store the result in the variable cube
. Finally, we use printf
to display the message “The cube of num is cube”, where num
and cube
are replaced by their respective values.
For example, if the user enters the value 5, the program will output the following message:
Enter an integer: 5
The cube of 5 is 125
Note that we use the %d format specifier to read and display integers, and we use the & operator to pass the memory address of the variable to scanf for input. We also use the \n character in the printf statement to print a newline character after the message.