Operators in C Programming
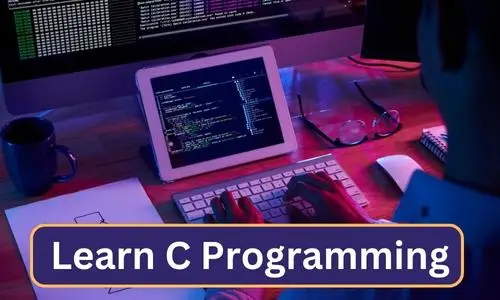
Page Index
Operators in C programming are symbols or keywords that are used to perform various operations on variables or values. These operations include arithmetic operations such as addition, subtraction, multiplication, and division, as well as logical operations such as AND, OR, and NOT.
C operators are classified into several categories based on their functionality.
- Arithmetic operators: These operators are used to perform mathematical operations like addition (+), subtraction (-), multiplication (*), division (/), and modulus (%).
- Logical operators: These operators are used to perform logical operations like AND (&&), OR (||), and NOT (!).
- Relational operators: These operators are used to compare two values or variables. Some of the relational operators in C include > (greater than), < (less than), >= (greater than or equal to), <= (less than or equal to), == (equal to), and != (not equal to).
- Bitwise operators: These operators are used to perform bitwise operations on binary numbers. Some of the bitwise operators in C include & (bitwise AND), | (bitwise OR), ^ (bitwise XOR), ~ (bitwise NOT), << (left shift), and >> (right shift).
- Assignment operators: These operators are used to assign values to variables. Some of the assignment operators in C include = (simple assignment), += (add and assign), -= (subtract and assign), *= (multiply and assign), /= (divide and assign), %= (modulus and assign), &= (bitwise AND and assign), |= (bitwise OR and assign), ^= (bitwise XOR and assign), <<= (left shift and assign), and >>= (right shift and assign).
- Increment and decrement operators: These operators are used to increase or decrease the value of a variable by one. The symbols used for increment and decrement operators are ++ (increment) and — (decrement).
Type Operators in C Programming
Arithmetic Operators
Arithmetic operators are used to perform mathematical operations on numerical values. There are five arithmetic operators in C:
- Addition operator (+): Adds two operands together
- Subtraction operator (-): Subtracts the second operand from the first operand
- Multiplication operator (*): Multiplies two operands together
- Division operator (/): Divides the first operand by the second operand
- Modulus operator (%): Computes the remainder after division of the first operand by the second operand
Here’s a table of examples showing how each of these arithmetic operators works:
Operator | Description | Example |
---|---|---|
+ | Addition | 5 + 3 = 8 |
– | Subtraction | 5 – 3 = 2 |
* | Multiplication | 5 * 3 = 15 |
/ | Division | 5 / 3 = 1 (integer division) |
% | Modulus (remainder) | 5 % 3 = 2 |
And here’s a sample program that demonstrates the use of arithmetic operators in C:
#include <stdio.h>
int main() {
int a = 5;
int b = 3;
int c = a + b;
int d = a - b;
int e = a * b;
int f = a / b;
int g = a % b;
printf("a + b = %d\n", c);
printf("a - b = %d\n", d);
printf("a * b = %d\n", e);
printf("a / b = %d\n", f);
printf("a %% b = %d\n", g);
return 0;
}
In this program, we declare five variables (a, b, c, d, e, f, and g) and initialize a and b to the values 5 and 3, respectively. We then use the arithmetic operators to perform various calculations and assign the results to the other variables. Finally, we print the results using printf statements.
Output of the above C program
a + b = 8
a - b = 2
a * b = 15
a / b = 1
a % b = 2
This is because the program performs the following calculations:
- a + b (5 + 3) = 8
- a – b (5 – 3) = 2
- a * b (5 * 3) = 15
- a / b (5 / 3) = 1 (since both operands are integers, this division results in an integer quotient)
- a % b (5 % 3) = 2 (the remainder when 5 is divided by 3)
Logical Operators
Logical operators are used to perform logical operations on boolean values (i.e., true/false). There are three logical operators in C:
- AND operator (&&): Returns true if both operands are true
- OR operator (||): Returns true if either operand is true
- NOT operator (!): Returns the opposite of the operand’s value (i.e., true if the operand is false, and false if the operand is true)
Here’s a table of examples showing how each of these logical operators works:
Operator | Description | Example |
&& | AND | true && false = false |
|| | OR | true || false = true |
! | NOT | !(true) = false |
And here’s a sample program that demonstrates the use of logical operators in C:
#include <stdio.h>
#include <stdbool.h>
int main() {
bool a = true;
bool b = false;
bool c = a && b;
bool d = a || b;
bool e = !a;
printf("a && b = %d\n", c);
printf("a || b = %d\n", d);
printf("!a = %d\n", e);
return 0;
}
In this program, we declare three boolean variables (a, b, and c) and initialize a to true and b to false. We then use the logical operators to perform various logical operations and assign the results to the other variables. Finally, we print the results using printf statements.
Note: We use the header file “stdbool.h” to include the boolean data type in this program. Without this header file, we would need to use integer values (0 for false and any nonzero value for true) to represent boolean values.
The output of this program would be:
a && b = 0
a || b = 1
!a = 0
This is because the program performs the following logical operations:
- a && b (true && false) = false
- a || b (true || false) = true
- !a (!true) = false
And then prints out each result using the printf function.
Relational Operators
Relational operators are used to compare two values or variables. They return a boolean value (i.e., true/false) indicating the result of the comparison. There are six relational operators in C:
- Greater than operator (>): Returns true if the first operand is greater than the second operand
- Less than operator (<): Returns true if the first operand is less than the second operand
- Greater than or equal to operator (>=): Returns true if the first operand is greater than or equal to the second operand
- Less than or equal to operator (<=): Returns true if the first operand is less than or equal to the second operand
- Equal to operator (==): Returns true if the two operands are equal
- Not equal to operator (!=): Returns true if the two operands are not equal
Here’s a table of examples showing how each of these relational operators works:
Operator | Description | Example |
> | Greater than | 5 > 3 = true |
< | Less than | 5 < 3 = false |
>= | Greater than or equal to | 5 >= 5 = true |
<= | Less than or equal to | 5 <= 3 = false |
== | Equal to | 5 == 3 = false |
!= | Not equal to | 5 != 3 = true |
Sample program that demonstrates the use of relational operators in C:
#include <stdio.h>
int main() {
int a = 5;
int b = 3;
if (a > b) {
printf("a is greater than b\n");
}
else {
printf("a is not greater than b\n");
}
if (a == b) {
printf("a is equal to b\n");
}
else {
printf("a is not equal to b\n");
}
return 0;
}
In this program, we declare two variables (a and b) and initialize a to 5 and b to 3. We then use the greater than and equal to operators to perform comparisons and print the results using if statements and printf statements.
The output of this program would be:
a is greater than b
a is not equal to b
This is because the program compares the values of a and b using the greater than and equal to operators and prints out the appropriate message for each comparison.
Bitwise Operators
Bitwise operators are used to perform bitwise operations on binary numbers. They manipulate individual bits of values or variables. There are six bitwise operators in C:
- Bitwise AND operator (&): Performs a bitwise AND operation on the two operands
- Bitwise OR operator (|): Performs a bitwise OR operation on the two operands
- Bitwise XOR operator (^): Performs a bitwise exclusive OR operation on the two operands
- Bitwise NOT operator (~): Performs a bitwise NOT operation on the operand (i.e., inverts all the bits)
- Left shift operator (<<): Shifts the bits of the first operand to the left by the number of positions specified in the second operand
- Right shift operator (>>): Shifts the bits of the first operand to the right by the number of positions specified in the second operand.
Here’s a table of examples showing how each of these bitwise operators works:
Operator | Description | Example |
& | Bitwise AND | 5 & 3 = 1 |
| | Bitwise OR | 5 | 3 = 7 |
^ | Bitwise XOR | 5 ^ 3 = 6 |
~ | Bitwise NOT | ~5 = -6 (assuming a 32-bit integer) |
<< | Left shift | 5 << 2 = 20 |
>> | Right shift | 5 >> 2 = 1 |
Note that the examples in the table are based on binary representations of the operands (5 and 3) and may not seem intuitive. It’s recommended to use binary representation to understand how these operators work.
And here’s a sample program that demonstrates the use of bitwise operators in C:
#include <stdio.h>
int main() {
int a = 5;
int b = 3;
int c = a & b;
int d = a | b;
int e = a ^ b;
int f = ~a;
int g = a << 2;
int h = a >> 2;
printf("a & b = %d\n", c);
printf("a | b = %d\n", d);
printf("a ^ b = %d\n", e);
printf("~a = %d\n", f);
printf("a << 2 = %d\n", g);
printf("a >> 2 = %d\n", h);
return 0;
}
In this program, we declare six variables (a, b, c, d, e, f, g, and h) and initialize a to 5 and b to 3. We then use the bitwise operators to perform various bitwise operations and assign the results to the other variables. Finally, we print the results using printf statements.
The output of this program would be:
a & b = 1
a | b = 7
a ^ b = 6
~a = -6
a << 2 = 20
a >> 2 = 1
This is because the program performs the following bitwise operations:
- a & b (101 & 011) = 001 (binary) = 1 (decimal)
- a | b (101 | 011) = 111 (binary) = 7 (decimal)
- a ^ b (101 ^ 011) = 110 (binary) = 6 (decimal)
- ~a (ones complement of 101) = 11111111 11111111111 11111111 11111111 11111010 (32-bit two’s complement representation) = -6 (decimal)
- a << 2 (101 shifted two bits to the left) = 10100 (binary) = 20 (decimal)
- a >> 2 (101 shifted two bits to the right) = 1 (binary and decimal)
Assignment operators
Assignment operators are used to assign values to variables. They combine an arithmetic or bitwise operation with an assignment operation. There are eight assignment operators in C:
- Simple assignment operator (=): Assigns the value on the right-hand side to the variable on the left-hand side
- Addition assignment operator (+=): Adds the value on the right-hand side to the variable on the left-hand side and assigns the result to the variable on the left-hand side
- Subtraction assignment operator (-=): Subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result to the variable on the left-hand side
- Multiplication assignment operator (*=): Multiplies the variable on the left-hand side by the value on the right-hand side and assigns the result to the variable on the left-hand side
- Division assignment operator (/=): Divides the variable on the left-hand side by the value on the right-hand side and assigns the result to the variable on the left-hand side
- Modulus assignment operator (%=): Computes the modulus of the variable on the left-hand side with the value on the right-hand side and assigns the result to the variable on the left-hand side
- Bitwise AND assignment operator (&=): Performs a bitwise AND operation between the variable on the left-hand side and the value on the right-hand side and assigns the result to the variable on the left-hand side
- Bitwise OR assignment operator (|=): Performs a bitwise OR operation between the variable on the left-hand side and the value on the right-hand side and assigns the result to the variable on the left-hand side
Here’s a table of examples showing how each of these assignment operators works:
Operator | Description | Example |
= | Simple assignment | int a = 5; |
+= | Addition assignment | a += 3; // equivalent to a = a + 3; |
-= | Subtraction assignment | a -= 3; // equivalent to a = a – 3; |
*= | Multiplication assignment | a *= 3; // equivalent to a = a * 3; |
/= | Division assignment | a /= 3; // equivalent to a = a / 3; |
%= | Modulus assignment | a %= 3; // equivalent to a = a % 3; |
&= | Bitwise AND assignment | a &= 3; // equivalent to a = a & 3; |
|= | Bitwise OR assignment | a |= 3; // equivalent to a = a | 3; |
And here’s a sample program that demonstrates the use of assignment operators in C:
#include <stdio.h>
int main() {
int a = 5;
a += 3; // equivalent to a = a + 3;
printf("a = %d\\n", a);
a -= 2; // equivalent to a = a - 2;
printf("a = %d\\n", a);
a *= 4; // equivalent to a = a * 4;
printf("a = %d\\n", a);
a /= 2; // equivalent to a = a / 2;
printf("a = %d\\n", a);
a %= 3; // equivalent to a = a % 3;
printf("a = %d\\n", a);
a &= 1; // equivalent to a = a & 1;
printf("a = %d\\n", a);
a |= 2; // equivalent to a = a | 2;
printf("a = %d\\n", a);
return 0;
}
In this program, we declare a variable “a” and initialize it to 5. We then use various assignment operators to perform arithmetic and bitwise operations on the variable and print the results using printf statements.
The output of this program would be:
a = 8
a = 6
a = 24
a = 12
a = 0
a = 2
This is because the program uses the various assignment operators to perform the following operations:
- a += 3: Adds 3 to a, so a becomes 8
- a -= 2: Subtracts 2 from a, so a becomes 6
- a *= 4: Multiplies a by 4, so a becomes 24
- a /= 2: Divides a by 2, so a becomes 12
- a %= 3: Computes the remainder of a divided by 3, which is 0, so a becomes 0
- a &= 1: Performs a bitwise AND operation between a and 1, so a becomes 0
- a |= 2: Performs a bitwise OR operation between a and 2, so a becomes 2
And then prints out each value of a using the printf function.
Increment and decrement operators
Increment and decrement operators are used to increase or decrease the value of a variable by 1. They can be used either as prefix operators (++variable) or postfix operators (variable++). Here’s a table of examples showing how they work:
Operator | Description | Example |
---|---|---|
++ | Increment | int a = 5; ++a; // a is now 6 |
— | Decrement | int a = 5; –a; // a is now 4 |
++ | Prefix increment | int a = 5; int b = ++a; // a is now 6, b is 6 |
— | Prefix decrement | int a = 5; int b = –a; // a is now 4, b is 4 |
++ | Postfix increment | int a = 5; int b = a++; // a is now 6, b is 5 |
— | Postfix decrement | int a = 5; int b = a–; // a is now 4, b is 5 |
Note that in the case of prefix operators, the variable is incremented or decremented before it is used in the expression, while in the case of postfix operators, the variable is used in the expression and then incremented or decremented.
Here’s a sample program that demonstrates the use of increment and decrement operators in C:
#include <stdio.h>
int main() {
int a = 5;
printf("a = %d\\n", a);
++a; // prefix increment, equivalent to a = a + 1;
printf("a = %d\\n", a);
--a; // prefix decrement, equivalent to a = a - 1;
printf("a = %d\\n", a);
int b = a++; // postfix increment, equivalent to b = a; a = a + 1;
printf("a = %d, b = %d\\n", a, b);
b = --a; // prefix decrement, equivalent to a = a - 1; b = a;
printf("a = %d, b = %d\\n", a, b);
return 0;
}
In this program, we declare a variable “a” and initialize it to 5. We then use various increment and decrement operators to modify the value of “a” and assign the result to other variables “b” and print the results using printf statements.
The output of this program would be:
a = 5
a = 6
a = 5
a = 6, b = 5
a = 5, b = 5
This is because the program uses the various increment and decrement operators to perform the following operations:
- ++a: Prefix increment, adds 1 to a, so a becomes 6
- -a: Prefix decrement, subtracts 1 from a, so a becomes 5 again
- a++: Postfix increment, assigns the value of a to b (5) and then adds 1 to a, so a becomes 6 and b remains 5
- -a: Prefix decrement subtracts 1 from a, so a becomes 5 again and assigns the new value to b, so b also becomes 5