Literals in C Programming
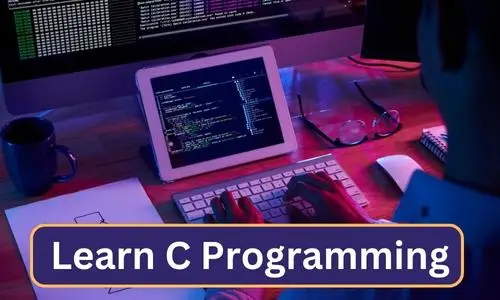
Page Index
Literals in C Programming language refers to a fixed value that is directly written in the source code. These values can be of various types such as integer, floating-point, character, string, or boolean.
For example, the integer literal “42” represents the value 42, the floating-point literal “3.14” represents the value 3.14, the character literal ‘A’ represents the ASCII code of the letter A, and the string literal “Hello World!” represents a string of characters.
Literals are commonly used in C programs to initialize variables or to pass values to functions. For instance, we can assign an integer literal to an integer variable as follows:
int x = 42; // assigning integer literal 42 to integer variable x
Similarly, we can pass a string literal as an argument to a function that expects a string:
printf("Hello, World!"); // printing string literal "Hello, World!" using printf() function
Note that literals are fixed values and cannot be modified during the execution of the program. Therefore, they are sometimes referred to as constants.
Type of Literals in C Programming
In C programming language, there are different types of literals based on the data they represent. The most commonly used types of literals are:
- Integer literals: These are used to represent whole numbers. They can be written in decimal, octal (prefixed with 0), or hexadecimal (prefixed with 0x) format. Examples include 42, 077, and 0x2A.
- Floating-point literals: These are used to represent decimal numbers with fractional parts. They can be written in standard or scientific notation. Examples include 3.14, 6.02e23, and -0.5.
- Character literals: These are used to represent single characters enclosed in single quotes. Examples include ‘A’, ‘7’, and ‘\n’ (newline character).
- String literals: These are used to represent a sequence of characters enclosed in double quotes. Examples include “Hello, World!”, “42”, and “C Programming”.
- Boolean literals: These are used to represent true or false values. In C, true is represented by the value 1, and false is represented by the value 0.
- Null literals: These are used to represent a null pointer. In C, the null literal is represented by the keyword “NULL”.
Understanding the different types of literals is important in programming as they are the basic building blocks for creating variables and performing calculations in C programs.
Integer literal with code and output
An integer literal is a type of literal used in C programming language to represent a whole number. An integer literal can be written in decimal, octal, or hexadecimal format.
In decimal format, an integer literal is simply a sequence of digits. In octal format, it is represented by a sequence of digits preceded by a 0. In hexadecimal format, it is represented by a sequence of digits and/or letters preceded by 0x or 0X.
Here’s an example code that demonstrates the use of integer literals in C:
#include <stdio.h>
int main() {
int a = 42; // decimal format
int b = 077; // octal format
int c = 0x2A; // hexadecimal format
printf("Decimal: %d\\nOctal: %o\\nHexadecimal: %X\\n", a, b, c);
return 0;
}
In this example, we have declared three integer variables a
, b
, and c
, and assigned them integer literals in decimal, octal, and hexadecimal format respectively. The printf()
function is then used to print the values of these variables in decimal, octal, and hexadecimal format using the %d
, %o
, and %X
format specifiers respectively.
When we run this program, the output will be:
Decimal: 42
Octal: 77
Hexadecimal: 2A
This shows that the integer literals in different formats are correctly assigned to the variables, and their values are printed in the correct format using the printf()
function.
Floating-point literals with code and output
A floating-point literal is a type of literal used in C programming language to represent decimal numbers with fractional parts. Floating-point literals can be written in standard or scientific notation.
In standard notation, a floating-point literal is represented by a sequence of digits, followed by a decimal point and another sequence of digits. In scientific notation, it is represented by a sequence of digits, followed by the letter ‘e’ or ‘E’, followed by another sequence of digits representing the exponent.
Here’s an example code that demonstrates the use of floating-point literals in C:
#include <stdio.h>
int main() {
float a = 3.14f; // standard notation
double b = 6.02e23; // scientific notation
long double c = -0.5L; // standard notation with long double precision
printf("Float: %f\\nDouble: %lf\\nLong Double: %Lf\\n", a, b, c);
return 0;
}
In this example, we have declared three floating-point variables a
, b
, and c
, and assigned them floating-point literals in standard and scientific notation with different precision. The printf()
function is then used to print the values of these variables using the %f
, %lf
, and %Lf
format specifiers for float, double, and long double precision respectively.
When we run this program, the output will be:
Float: 3.140000
Double: 602000000000000000000000.000000
Long Double: -0.500000
This shows that the floating-point literals in different formats are correctly assigned to the variables, and their values are printed in the correct format using the printf()
function. Note that the precision of the long double value is higher than that of float and double.
Character literals with code and output
A character literal is a type of literal used in C programming language to represent a single character enclosed in single quotes.
Here’s an example code that demonstrates the use of character literals in C:
#include <stdio.h>
int main() {
char a = 'A'; // character literal
char b = 48; // ASCII code for '0'
char c = '\\n'; // newline character
printf("Character a: %c\\n", a);
printf("Character b: %c\\n", b);
printf("Character c: %c", c);
return 0;
}
In this example, we have declared three character variables a
, b
, and c
, and assigned them character literals representing the letters ‘A’, the digit ‘0’, and a newline character respectively.
The printf()
function is then used to print the values of these variables using the %c
format specifier for character values.
When we run this program, the output will be:
Character a: A
Character b: 0
Character c:
This shows that the character literals are correctly assigned to the variables, and their values are printed using the printf()
function. Note that the ASCII code for the character ‘0’ is 48, and the newline character is represented by the escape sequence ‘\n’.
Represent the character literal
In C programming language, a character literal is represented by enclosing a single character within single quotes (‘ ‘). The character can be any printable ASCII character or a special character represented by an escape sequence.
For example, the character ‘A’ is represented by the character literal ‘A’. Similarly, the character ‘0’ is represented by the character literal ‘0’. Special characters such as newline, tab, backslash, and others are represented by escape sequences. For example, the newline character is represented by the escape sequence ‘\n’, the tab character is represented by ‘\t’, and the backslash character itself is represented by ‘\’.
Here are some examples of character literals:
char a = 'A'; // character literal representing the letter 'A'
char b = '0'; // character literal representing the digit '0'
char c = '\\n'; // character literal representing the newline character
char d = '\\t'; // character literal representing the tab character
char e = '\\\\'; // character literal representing the backslash character
Note that a character literal can only represent a single character. If you want to represent a sequence of characters, you should use a string literal enclosed within double quotes (” “).
String Literal :
A string literal is a type of literal used in C programming language to represent a sequence of characters enclosed in double quotes.
Here’s an example code that demonstrates the use of string literals in C:
#include <stdio.h>
int main() {
char greeting[] = "Hello, World!"; // string literal
printf("%s\\n", greeting);
return 0;
}
In this example, we have declared a character array greeting
and assigned it a string literal “Hello, World!”. The printf()
function is then used to print the value of the greeting
array using the %s
format specifier for string values.
When we run this program, the output will be:
Hello, World!
This shows that the string literal is correctly assigned to the character array and its value is printed using the printf()
function.
In C programming language, a string literal is a constant and cannot be modified. If you want to manipulate a string, you should create a character array and copy the string literal into it.
Here’s an example code that demonstrates copying a string literal into a character array:
#include <stdio.h>
#include <string.h>
int main() {
char greeting[] = "Hello, World!"; // string literal
char message[20];
strcpy(message, greeting); // copy string literal to character array
printf("%s\\n", message);
return 0;
}
In this example, we have declared two character arrays greeting
and message
. The strcpy()
function is used to copy the string literal “Hello, World!” from the greeting
array to the message
array. The printf()
function is then used to print the value of the message
array.
When we run this program, the output will be:
Hello, World!
This shows that the string literal is copied into the character array and its value is printed using the printf()
function.
Boolean Literals:
A boolean literal is a type of literal used in C programming language to represent a true or false value. In C, true is represented by the value 1, and false is represented by the value 0.
Here’s an example code that demonstrates the use of boolean literals in C:
#include <stdio.h>
#include <stdbool.h>
int main() {
bool a = true; // boolean literal representing true
bool b = false; // boolean literal representing false
printf("Boolean a: %d\\n", a);
printf("Boolean b: %d\\n", b);
return 0;
}
In this example, we have declared two boolean variables a
and b
, and assigned them boolean literals representing true and false respectively.
The printf()
function is then used to print the values of these variables using the %d
format specifier for integer values.
When we run this program, the output will be:
Boolean a: 1
Boolean b: 0
This shows that the boolean literals representing true and false are correctly assigned to the variables, and their values are printed using the printf()
function as integer values 1 and 0 respectively. Note that the boolean literals are automatically converted to integer values when used with the %d
format specifier.
The <stdbool.h>
header file provides a standard way to work with boolean values in C. It defines the bool
type and the true
and false
constants. The use of bool
and true/false
constants improves code readability and makes the intent of the code clearer.
Null Pointer
In C programming language, a null literal is used to represent a null pointer. A null pointer is a pointer that does not point to any memory location. In C, the null literal is represented by the keyword “NULL”.
Here’s an example code that demonstrates the use of null literals in C:
#include <stdio.h>
int main() {
int *ptr = NULL; // null literal representing a null pointer
printf("%p\n", ptr);
return 0;
}
In this example, we have declared a pointer variable ptr
and assigned it a null literal using the keyword “NULL”.
The printf()
function is then used to print the value of the ptr
variable using the %p
format specifier for pointer values.
When we run this program, the output will be:
0x0
This shows that the null literal representing a null pointer is correctly assigned to the pointer variable ptr
, and its value is printed using the printf()
function as the memory address 0x0.
Note that dereferencing a null pointer is undefined behavior and can lead to unexpected results or even program crashes. Therefore, it is important to always check whether a pointer is null before dereferencing it.
Here’s an example code that demonstrates checking for a null pointer:
#include <stdio.h>
int main() {
int *ptr = NULL;
if (ptr == NULL) {
printf("Pointer is null.\\n");
} else {
printf("Pointer is not null.\\n");
}
return 0;
}
In this example, we use the equality operator ==
to check whether the pointer ptr
is null. If the pointer is null, we print the message “Pointer is null.” Otherwise, we print the message “Pointer is not null.”
When we run this program, the output will be:
Pointer is null.
This shows that the null pointer is correctly detected using the equality operator ==
.