Keywords in C Programming
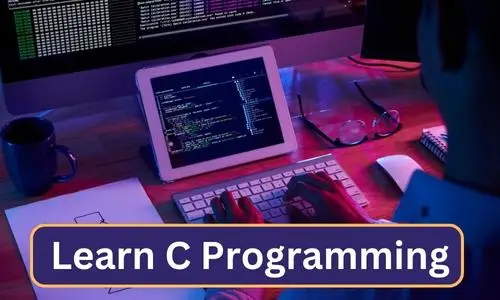
Page Index
Keywords in C Programming are the reserved words that have a predefined meaning and functionality in the C programming language. These keywords cannot be used as identifiers or variable names because they are specifically reserved for certain purposes within the language.
Some examples of keywords in C include “int”, “float”, “double”, “char”, “void”, “if”, “else”, “while”, “for”, “switch”, “case”, “default”, “return”, “break”, “continue”, “typedef”, “struct”, “union”, “enum”, “sizeof”, and “const”.
These keywords serve as building blocks for programming in C, and their proper usage is essential for writing correct and effective C code.
Keyword | Description |
auto | Specifies automatic storage class |
break | Terminates the nearest enclosing loop or switch statement |
case | Marks a branch in a switch statement |
char | Declares a character data type |
const | Specifies that a variable’s value cannot be modified |
continue | Causes the loop to skip the current iteration |
default | Specifies the default branch in a switch statement |
do | Initiates a do-while loop |
double | Declares a double-precision floating-point data type |
else | Specifies an alternative branch in an if statement |
enum | Defines an enumerated data type |
extern | Specifies external linkage for a variable or function |
float | Declares a floating-point data type |
for | Initiates a for loop |
goto | Transfers control to a labeled statement |
if | Specifies a conditional statement |
int | Declares an integer data type |
long | Declares a long integer data type |
register | Specifies register storage class |
return | Returns a value from a function |
short | Declares a short integer data type |
signed | Declares a signed data type |
sizeof | Returns the size of a data type or variable |
static | Specifies static storage class |
struct | Defines a structure data type |
switch | Initiates a switch statement |
typedef | Creates a new data type |
union | Defines a union data type |
unsigned | Declares an unsigned data type |
void | Specifies a void data type or function return type |
volatile | Specifies that a variable’s value can be modified by hardware or another thread |
while | Initiates a while loop |
Types of Keywords in C Programming
Auto Keyword
The auto keyword in C programming is used to declare automatic variables, which are local to the function in which they are defined. By default, all local variables in C are auto variables, so the explicit use of the auto keyword is quite rare and often redundant.
Here’s a simple example to illustrate the use of auto:
#include <stdio.h>
void exampleFunction() {
auto int a = 10; // Declaring an auto variable (local to this function)
printf("Value of a: %d\n", a);
}
int main() {
exampleFunction();
// 'a' is not accessible here, as it's local to exampleFunction.
return 0;
}
Output:
Value of a: 10
In this program:
auto int a = 10;
declares an automatic variablea
and initializes it to 10. This variable is local toexampleFunction
.- The
printf
statement insideexampleFunction
prints the value ofa
. - The variable
a
cannot be accessed outsideexampleFunction
because it is an automatic (local) variable.
This example demonstrates how auto
variables are confined to the scope of the function in which they are declared. Remember, using auto
is optional since local variables are auto
by default.
Break and Continue Keyword
In C programming, break
and continue
are control flow statements that are used within loops (for
, while
, do-while
) and switch
cases.
- break: This keyword is used to immediately exit the loop or
switch
case in which it’s placed. Afterbreak
is executed, the control flow jumps to the statement following the loop orswitch
. - continue: This keyword skips the remaining code in the current iteration of the loop and jumps to the next iteration. In
for
loops, it first executes the update statement before checking the condition again.
Here’s an example program to demonstrate break
and continue
:
#include <stdio.h>
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
continue; // Skip the rest of the loop when i is 5
}
if (i == 8) {
break; // Exit the loop when i is 8
}
printf("%d ", i);
}
return 0;
}
Output:
0 1 2 3 4 6 7
Explanation:
- The loop iterates from 0 to 9.
- When
i
is equal to 5,continue
is executed, skipping theprintf
statement fori = 5
. - When
i
is equal to 8,break
is executed, causing an immediate exit from the loop. Therefore,printf
is not executed fori = 8
andi = 9
. - For all other values of
i
, the number is printed.
Switch, Case, and Default Keyword
switch
, case
, and default
are keywords used together to create a switch
statement, which is a type of control structure that allows you to execute different code segments based on the value of a variable or an expression. Here’s a brief explanation of each:
switch
: This keyword is used to start aswitch
statement. It is followed by a variable or an expression in parentheses. The value of this variable or expression is then compared with the values specified in thecase
labels.case
: Eachcase
keyword is followed by a value and a colon. It specifies a match for the value of the variable or expression used in theswitch
. If the value matches, the code following thatcase
label is executed until abreak
statement or the end of theswitch
block is reached.default
: This keyword is used as a fallback case in aswitch
statement. If none of thecase
values match the value of theswitch
expression, the code following thedefault
label is executed. Thedefault
case is optional and does not need abreak
statement, as it is always the last case.
Here’s a simple example to illustrate how these keywords are used together in a program:
#include <stdio.h>
int main() {
int number = 2;
switch (number) {
case 1:
printf("Number is one.\n");
break;
case 2:
printf("Number is two.\n");
break;
case 3:
printf("Number is three.\n");
break;
default:
printf("Number is not one, two, or three.\n");
}
return 0;
}
In this program, the switch statement evaluates the variable number. Since its value is 2, the program matches this with the case 2: label and executes the code in that block, printing “Number is two.” to the console.
If you run this program, the output will be:
Number is two.
The break statements are used to exit the switch block after a matching case is executed. Without a break, the program would continue executing the code in subsequent cases until a break is encountered or the end of the switch block is reached.
Char Keyword
char keyword is used to declare a character type variable. It is a data type that can store a single character, typically occupying one byte of memory. Characters in C are stored using their ASCII values, an encoding standard for representing characters as numbers.
Here’s a brief explanation of the char data type, followed by a simple program to demonstrate its usage:
1. char Data Type: A char variable can hold a single character, such as ‘A’, ‘z’, ‘3’, or even special characters like ‘@’. It can also store ASCII values directly, and you can perform arithmetic operations on these values.
2. Character Literals: In C, character literals are enclosed in single quotes, like ‘a’, ‘b’, etc. They represent the ASCII value of the character.
Here is a simple example program that uses the char data type:
#include <stdio.h>
int main() {
char letter = 'A';
printf("The character is: %c\n", letter);
printf("ASCII value of the character is: %d\n", letter);
// Incrementing the character
letter++;
printf("Next character is: %c\n", letter);
printf("ASCII value of next character is: %d\n", letter);
return 0;
}
In this program:
- We declare a
char
variableletter
and initialize it with the character ‘A’. - We use
%c
inprintf
to print the character stored inletter
. - We also use
%d
to print the ASCII value of the character. - Then, we increment the
letter
variable usingletter++
, which changes its value to the next character in the ASCII sequence.
If you run this program, the output will be:
The character is: A
ASCII value of the character is: 65
Next character is: B
ASCII value of next character is: 66
This output shows the initial character (‘A’) and its ASCII value (65), followed by the character after incrementing (‘B’) and its ASCII value (66).
Const Keyword
In C programming, the const
keyword is used to declare variables whose values cannot be modified after they are set. Essentially, const
creates read-only variables. This is useful for defining constants, ensuring that the value doesn’t accidentally get changed elsewhere in the program.
Here’s a closer look at how const
works:
const
Variables: When a variable is declared withconst
, it means that its value cannot be altered after initialization. If you try to change aconst
variable, the compiler will throw an error.- Usage in Functions: The
const
keyword can also be used in function parameters to indicate that the function should not modify the argument. - Pointers and
const
:const
can be used with pointers in different ways. For instance, you can declare a pointer to aconst
data, meaning you cannot modify the data through this pointer, or aconst
pointer, which means you cannot change what the pointer points to.
Here’s a simple example to illustrate the use of const
:
#include <stdio.h>int main() {
const int MY_CONST = 10;
printf("The constant value is: %d\n", MY_CONST);
// Uncommenting the following line would cause a compile-time error
// MY_CONST = 20;
return 0;
}
In this program:
- We declare a
const int
variableMY_CONST
and initialize it with the value10
. - We then print the value of
MY_CONST
. - If you try to uncomment the line
MY_CONST = 20;
, the compiler will generate an error because you are attempting to modify aconst
variable.
When you run this program, the output will be:
The constant value is: 10
This output shows the value of the constant. Remember, if you try to modify MY_CONST
by removing the comment, the program will not compile, demonstrating the enforcement of the const
qualifier.
Do Keyword
The do
keyword is used as part of the do-while
loop, a post-tested loop that ensures the code block inside the loop is executed at least once. The do-while
loop is similar to the while
loop, but the key difference is that the do-while
loop checks its condition at the end of the loop body.
Here’s how it works:
- Structure of
do-while
Loop:- The loop starts with the
do
keyword, followed by a block of code enclosed in curly braces{}
. - After the code block, the
while
keyword is used, followed by a condition in parentheses()
. - The loop ends with a semicolon
;
.
- The loop starts with the
- Execution Flow:
- The code block inside the
do
part is executed first. - After executing the block, the condition specified in the
while
part is evaluated. - If the condition is true, the loop runs again, repeating the code block. If the condition is false, the loop ends.
- The code block inside the
- Use Case: The
do-while
loop is particularly useful when you need to ensure that the loop body is executed at least once, regardless of whether the loop condition is initially true or false.
Here is an example program that uses a do-while
loop:
#include <stdio.h>int main() {
int count = 1;
do {
printf("Count is: %d\\n", count);
count++;
} while (count <= 5);
return 0;
}
In this program:
- We have a counter variable
count
initialized to1
. - Inside the
do-while
loop, we print the value ofcount
and then increment it. - The loop continues to run as long as
count
is less than or equal to5
.
If you run this program, the output will be:
Count is: 1
Count is: 2
Count is: 3
Count is: 4
Count is: 5
This output shows the loop running five times, incrementing and printing count
each time until the condition count <= 5
becomes false.
Double and Float Keyword
In C programming, float
and double
are two data types used for representing floating-point numbers, which are numbers that can have a fractional part. These two types differ in their precision and range:
float
:- A
float
variable is a single-precision floating-point data type. - It typically requires 4 bytes of memory.
float
provides approximately 6-7 decimal digits of precision.- Suitable for most applications where approximate real numbers are sufficient.
- A
double
:- A
double
variable is a double-precision floating-point data type. - It usually takes 8 bytes of memory.
double
provides about 15-16 decimal digits of precision.- Preferred when higher precision in calculations is required.
- A
Here’s an example program illustrating the use of float
and double
:
#include <stdio.h>int main() {
float floatValue = 3.14159f; // 'f' suffix denotes a float literal
double doubleValue = 3.141592653589793;
printf("Float value is: %f\\n", floatValue);
printf("Double value is: %lf\\n", doubleValue);
return 0;
}
In this program:
- We declare a
float
variablefloatValue
and initialize it with a floating-point number. Thef
at the end of the number signifies that it is afloat
literal. - We also declare a
double
variabledoubleValue
with a more precise value. - Both values are printed using
printf
. Note that%f
is used for bothfloat
anddouble
inprintf
, but%lf
is more specifically fordouble
to indicate long float (double).
When you run this program, the output will be something like:
Float value is: 3.141590
Double value is: 3.141593
The output shows the floating-point numbers printed with their respective precision. The float
value is rounded to fewer decimal places than the double
value, demonstrating the difference in precision between these two types.
if-else Keyword
In C programming, if
and else
are conditional statements used to perform different actions based on different conditions. These keywords allow you to execute certain parts of code only when specific conditions are met.
if
Statement:- The
if
statement is used to test a condition. It is written asif (condition) { // code }
. - If the condition is true, the code inside the
if
block is executed. If the condition is false, theif
block is skipped.
- The
else
Statement:- The
else
statement is used in conjunction with anif
statement. - It is written as
else { // code }
and is placed after anif
block. - The code inside the
else
block is executed only if theif
condition is false.
- The
else if
Statement:- This is an optional extension of the
if
statement which allows you to check multiple conditions. - Written as
else if (condition) { // code }
, it is placed after anif
block and before anelse
block. - It lets you specify a new condition to test if the previous
if
condition was false.
- This is an optional extension of the
Here’s an example program using if
, else if
, and else
:
#include <stdio.h>int main() {
int number = 10;
if (number > 0) {
printf("Number is positive.\\n");
} else if (number < 0) {
printf("Number is negative.\\n");
} else {
printf("Number is zero.\\n");
}
return 0;
}
In this program:
- We have an integer variable
number
set to10
. - The
if (number > 0)
checks ifnumber
is greater than0
. Since it is, it prints “Number is positive.” - If
number
were less than0
, theelse if (number < 0)
block would execute. - If
number
were exactly0
, theelse
block would execute.
When you run this program, the output will be:
Number is positive.
This output indicates that the number
was indeed greater than 0
, so the code inside the if
block was executed. If you change the value of number
and run the program again, different blocks of code will execute based on the new value.
enum keyword
The enum
keyword in C programming is used to define an enumeration, a user-defined type that consists of a set of named integral constants. An enumeration is a way to group related constants together under a single name, making a program easier to read, maintain, and understand.
Here’s a breakdown of how enum
works and its features:
Basic Syntax
The basic syntax for defining an enumeration using the enum
keyword is as follows:
enum enum_name { constant1, constant2, ..., constantN };
enum_name
is the name of the enumeration.constant1
,constant2
, …,constantN
are the names of the constants that belong to the enumeration. These are often referred to as “enumerators”.
#include <stdio.h>enum week {Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday};
int main() {
enum week today;
today = Wednesday;
printf("Day %d", today);
return 0;
}