Identifiers In C Programming
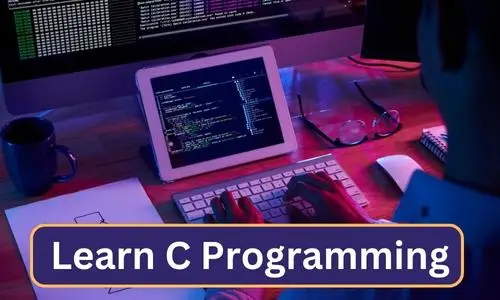
Page Index
Identifiers in C programming refer to the names given to various program entities such as variables, functions, arrays, structures, and so on. An identifier can be any sequence of letters, digits, and underscore character, but it must begin with a letter or underscore. Identifiers are used to give a unique name to a program element, so it can be referred to and used within the program.
For example, in the C program statement “int x = 10;”, “x” is an identifier that is used to give a name to the integer variable that stores the value 10. It can then be referenced and manipulated within the program using this name.
It is important to choose meaningful and descriptive names for identifiers to make the code more readable and easier to understand. Also, C programming language has some reserved keywords which cannot be used as identifiers.
Rules for constructing C identifiers
In C programming, identifiers are used to give a name to program entities such as variables, functions, arrays, structures, and so on. Identifiers are created based on a set of rules that must be followed. The rules for constructing C identifiers are as follows:
- The name must begin with a letter (uppercase or lowercase) or underscore (_).
- The rest of the name can consist of letters, digits, and underscores.
- The name must not be a C keyword or reserved word, such as “int”, “if”, “while”, “struct”, etc.
- Identifiers are case-sensitive, meaning “x” and “X” are two different identifiers.
- The maximum length of an identifier is implementation-defined, meaning it may vary between different C compilers.
It is important to follow these rules when creating identifiers in C programming to avoid syntax errors or conflicts with existing keywords or reserved words. Additionally, it is recommended to choose meaningful and descriptive names for identifiers to make the code more readable and easier to understand.
Examples of valid and invalid identifiers
Valid identifiers:
- myVariable
- my_variable
- MyVariable
- x
- _myVariable
- _x2
- MAX_VALUE
Invalid identifiers:
- 2x (must begin with a letter or underscore)
- my-variable (can only contain letters, digits, and underscores)
- void (a reserved keyword in C)
- while (a reserved keyword in C)
- My Variable (cannot contain spaces)
- very_long_identifier_name_that_exceeds_the_maximum_allowed_length (exceeds the maximum length limit)
It is important to remember that identifiers in C are case-sensitive, so “myVariable” and “MyVariable” are considered different identifiers. It is recommended to choose meaningful and descriptive names for identifiers to make the code more readable and easier to understand.
Types of Identifiers In C Programming
In C programming, different types of identifiers are used to name program entities. These include:
- Variables: Identifiers that are used to store values of different data types, such as integers, floating-point numbers, characters, and so on.
- Constants: Identifiers that are used to represent fixed values in a program, such as pi (3.14159), the speed of light (299792458), or the maximum value of an integer (2147483647).
- Functions: Identifiers that are used to define and call functions, which are blocks of code that perform specific tasks.
- Arrays: Identifiers that are used to store a collection of values of the same data type.
- Pointers: Identifiers that are used to store the memory address of a variable or function.
- Structures: Identifiers that are used to define custom data types that can hold different data types and values.
- Labels: Identifiers used to mark specific locations in a program, which can be used for branching and looping statements.
- Macros: Identifiers that are used to define symbolic constants or code snippets that can be used to simplify code or make it more readable.
It is important to choose meaningful and descriptive names for all identifiers to make the code more readable and easier to understand.
Differences between Keyword and Identifier in table form
Aspect | Keywords | Identifiers |
---|---|---|
Definition | Reserved words that have predefined meaning in C programming. | User-defined names that are given to program entities such as variables, functions, arrays, and so on. |
Examples | if, else, while, int, float, char, void, return, etc. | myVariable, calculateSum, MAX_VALUE, numberOfItems, etc. |
Usage | Cannot be used as identifiers for program entities. | Used to give a name to program entities. |
Number | Limited in number. There are only 32 keywords in C programming. | Unlimited in number. Identifiers can be created based on the rules of construction. |
Case-sensitivity | Keywords are always in lowercase. | Identifiers can be in any combination of uppercase, lowercase, and underscores. |
Conflict | Keywords cannot be used as identifiers for program entities. | Identifiers should not be the same as keywords to avoid conflicts and errors. |
Meaning | Keywords have a predefined meaning in C programming. | The meaning of an identifier is determined by its use and context within a program. |
Identifier Using C programming Language
#include <stdio.h>
int main() {
int age; // declaring an integer variable called "age"
printf("Enter your age: "); // asking for user input
scanf("%d", &age); // reading user input and storing it in "age" variable
printf("You are %d years old.", age); // displaying the value of "age" variable
return 0;
}
In this program, the identifier used is “age”, which is a variable of the “int” data type that is used to store the age of the user. The program prompts the user to enter their age, reads the input using the “scanf” function, and stores it in the “age” variable. Finally, the program displays the value of “age” using the “printf” function.
Identifiers like “age” can be used to name program entities such as variables, functions, arrays, and so on, and are essential in C programming for giving unique names to program elements so they can be easily referenced and used within the program.
example C program that demonstrates the case-sensitivity of identifiers, along with the output:
#include <stdio.h>
int main() {
int num1 = 10; // declaring an integer variable called "num1"
int Num1 = 20; // declaring another integer variable called "Num1"
printf("num1 = %d\\n", num1); // displaying the value of "num1"
printf("Num1 = %d\\n", Num1); // displaying the value of "Num1"
return 0;
}
Output:
num1 = 10
Num1 = 20
In this program, we have declared two integer variables with similar names, but different cases: “num1” and “Num1”. Since C programming is case-sensitive, these are treated as two separate and distinct variables, even though they have similar names.
When we run the program, the output displays the values of these variables separately. The first printf statement displays the value of “num1”, which is 10, while the second printf statement displays the value of “Num1”, which is 20. This shows that even though the variable names are similar, their case determines their identity and meaning in the program.