Data Type in C Programming
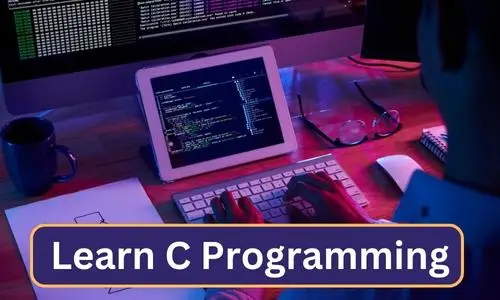
Page Index
Data type refers to the classification of data used in a program. It specifies the type of data that a variable can hold, the operations that can be performed on the variable, and the way the variable is stored in memory.
Classification of data type in c programming :
1. Primitive data types: These are the basic data types that are built into the language and are used to represent simple data values. They include:
Primitive data types are basic data types that are built into programming languages and cannot be broken down into simpler components. They are also often referred to as simple data types.
Here are some examples of primitive data types:
- Integer: This is a data type used to represent whole numbers, such as 1, 2, 3, -4, -5, etc. In most programming languages, integers are represented using the “int” keyword. For example:
int age = 25;
short num = 10;
long population = 1000000L;
long long bigNumber = 9223372036854775807LL;
2. Float: This is a data type used to represent decimal numbers with single precision, such as 3.14, 2.0, etc. In most programming languages, floats are represented using the “float” keyword. For example:
float price = 3.99f;
double pi = 3.14159265358979323846;
long double hugeNum = 1.23456789012345678901234567890L;
3. Double: This is a data type used to represent decimal numbers with double precision, such as 3.14159265359, 2.71828, etc. In most programming languages, doubles are represented using the “double” keyword. For example:
double gravity = 9.81;
4. Boolean: This is a data type used to represent true/false values. In most programming languages, booleans are represented using the “bool” keyword. For example:
bool isTall = true;
bool isSmart = false;
5. Character: This is a data type used to represent single characters, such as ‘a’, ‘b’, ‘c’, ‘A’, ‘B’, etc. In most programming languages, characters are represented using the “char” keyword. For example:
char letter = 'A';
char symbol = '$';
char newline = '\n';
These are just a few examples of primitive data types. Other primitive data types include byte, short, long, and void (in some programming languages).
2. Derived data types: These are data types that are created by combining primitive data types or by modifying them in some way. They include:
In C programming, derived data types are created by combining or modifying primitive data types or other derived data types. Here are some examples of derived data types:
1. Array data type:
An array is a derived data type that allows the storage of a collection of data items of the same type. For example:
int numbers[5] = {1, 2, 3, 4, 5};
In this example, numbers
is an array of integers that can hold 5 values. The values {1, 2, 3, 4, 5}
are assigned to the elements of the array.
2. Pointer data type:
A pointer is a derived data type that stores the memory address of another variable. For example:
int age = 25;
int *agePointer = &age;
In this example, agePointer
is a pointer to an integer that stores the memory address of the variable age
.
3. Structure data type:
A structure is a derived data type that allows the storage of a collection of data items of different types that are grouped together under a single name. For example:
struct person {
char name[50];
int age;
float height;
};
struct person john = {"John", 25, 1.82};
In this example, person
is a structure that contains three members: a character array name
, an integer age
, and a float height
. The variable john
is defined as a person
structure and initialized with the values {"John", 25, 1.82}
.
4. Union data type:
A union is a derived data type that allows the storage of different data types in the same memory location. For example:
union data {
int i;
float f;
char c;
};
union data value;
value.i = 10;
printf("value.i = %d\n", value.i);
value.f = 2.5;
printf("value.f = %f\n", value.f);
value.c = 'A';
printf("value.c = %c\n", value.c);
In this example, data
is a union that can hold an integer i
, a float f
, or a character c
. The variable value
is a data
union, and the values {10, 2.5, 'A'}
are assigned to the members of the union.
5. Enumeration data type:
An enumeration is a derived data type that consists of a set of named integer constants. For example:
enum weekday {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
};
enum weekday today = TUESDAY;
printf("Today is %d\n", today);
In this example, weekday is an enumeration that defines the names of the days of the week as integer constants. The variable today is defined as a weekday enumeration and assigned the value TUESDAY.
Data Type in Table View :
Data Type | Storage Size | Value Range |
char | 1 byte | -128 to 127 or 0 to 255 |
int | 2 or 4 bytes | -32,768 to 32,767 or -2,147,483,648 to 2,147,483,647 |
short | 2 bytes | -32,768 to 32,767 |
long | 4 bytes | -2,147,483,648 to 2,147,483,647 |
long long | 8 bytes | -(2^63) to (2^63)-1 |
float | 4 bytes | 1.2E-38 to 3.4E+38 |
double | 8 bytes | 2.3E-308 to 1.7E+308 |
long double | 10 or 16 bytes | 3.4E-4932 to 1.1E+4932 |
_Bool | 1 byte | 0 or 1 |
Example that demonstrates the usage of primitive data types in C:
#include <stdio.h>
int main() {
// Declare and initialize int, float, double, and char variables
int age = 30;
float height = 5.9;
double weight = 160.5;
char first_initial = 'A';
_Bool is_student = 0; // 0 for false, 1 for true
// Print the values of these variables
printf("Age: %d\n", age);
printf("Height: %.1f\n", height);
printf("Weight: %.1lf\n", weight);
printf("First initial: %c\n", first_initial);
printf("Is student? %s\n", is_student ? "Yes" : "No");
return 0;
}
This code initializes and prints the values of several primitive data types, including int, float, double, char, and _Bool. The printf function is used to display the values, with the appropriate format specifiers for each data type:
%d
: int%.1f
: float (with one decimal place)%.1lf
: double (with one decimal place)%c
: char%s
: string (used here to display “Yes” or “No” based on the boolean value)
Output :
Age: 30
Height: 5.9
Weight: 160.5
First initial: A
Is student? No