Constant in C Programming
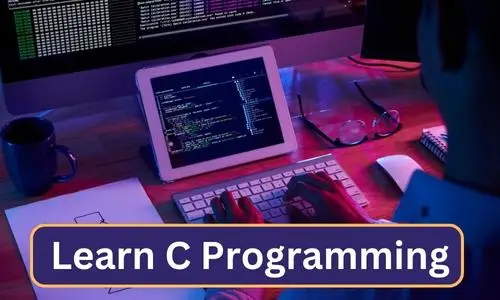
Page Index
Constant In C programming, is a value that cannot be changed during the execution of a program. Constants are used to represent fixed values that should not be modified or altered.
In C, there are two types of constants:
Numeric constants and Character constants.
Numeric Constants
Numeric constants are used to represent values such as integers, floating-point numbers or hexadecimal numbers. Numeric constants are declared using the following syntax:
const data_type constant_name = value;
Here is an example of how to define a constant for the value of pi:
const double PI = 3.14159265359;
In this example, the constant is declared as a double data type with a value of 3.14159265359.
Character Constants
Character constants are used to represent individual characters or strings of characters. Character constants are enclosed within single quotes (‘ ‘), such as:
const char LETTER = 'A';
Here is an example of how to define a constant for a string of characters:
const char* GREETING = "Hello, World!";
In this example, the constant is declared as a character pointer and contains a string of characters “Hello, World!”.
Enumeration Constants
Enumeration constants are used to represent a range of values that have a specific meaning. For example, you might use an enumeration constant to represent the days of the week:
enum DaysOfWeek { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday };
In this example, the enumeration constant “DaysOfWeek” defines seven constants, each representing a day of the week.
Macro Constants
Macro constants are a type of constant that is defined using the “#define” preprocessor directive. Macro constants are used to represent values that do not need to be assigned a data type. Here is an example of a macro constant:
#define MAX_SIZE 100
In this example, “MAX_SIZE” is a macro constant that represents the maximum size of an array. When the program is compiled, all instances of “MAX_SIZE” will be replaced with the value 100.
Types of constant in C
In C programming language, a constant is a value that cannot be altered during the execution of a program. There are different types of constants in C, each with its own syntax and rules. Here are some examples:
Integer Constants:
An integer constant is a number that has no decimal point. It can be either positive or negative, and can be represented in decimal, octal, or hexadecimal format.
Example:
int num = 100; // decimal integer constant
int num2 = 012; // octal integer constant (equals decimal 10)
int num3 = 0x64; // hexadecimal integer constant (equals decimal 100)
Floating Point Constants:
A floating-point constant is a number that has a decimal point. It can be represented in either the decimal or exponential form.
float num = 3.14; // decimal floating point constant
float num2 = 2E-3; // exponential floating point constant (equals 0.002)
Character Constants:
A character constant is a single character enclosed within single quotes.
Example:
char ch = 'a'; // character constant
String Constants:
A string constant is a sequence of characters enclosed within double quotes.
Example:
char str[] = "Hello, world!"; // string constant
Enumeration Constants:
An enumeration constant is a user-defined type that consists of a set of named constants.
Example:
enum days_of_week {Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday};
How to Define Constant in C Programming
In C programming language, you can define a constant using the #define directive or the const keyword. Here’s how to define constants using each method:
1. Using the #define directive:
The #define directive allows you to define a constant value that can be used throughout your program.
The syntax for #define is:
#define constant_name constant_value
Example:
#define PI 3.14159
In this example, we have defined a constant named PI with the value of 3.14159.
2. Using the const keyword:
The const keyword allows you to define a variable as constant, which means its value cannot be changed during the execution of the program. The syntax for const is:
const data_type constant_name = constant_value;
Example:
const int MAX_VALUE = 100;
In this example, we have defined a constant named MAX_VALUE
with the value of 100
as an integer.
Both #define
and const
can be used to define constants in C programming language. However, the const
keyword is preferred because it provides better type checking and is more flexible than the #define
directive.
C program that demonstrates the use of the #define preprocessor:
#include <stdio.h>
#define PI 3.14159
int main() {
float radius = 5.0;
float area = PI * radius * radius;
printf("The area of a circle with radius %.2f is %.2f\n", radius, area);
#define PI 3.14
area = PI * radius * radius;
printf("If we redefine PI as %.2f, the area becomes %.2f\n", PI, area);
return 0;
}
In this program, we define the constant PI using the #define preprocessor. We then use this constant to calculate the area of a circle with a given radius. Later in the program, we redefine the value of PI using #define, and show how this affects the calculation of the area.
Note: #define simply replaces any occurrence of the defined symbol with its value during preprocessing before the actual compilation of the program begins. This allows us to define constants or macros that can simplify code, improve readability, and reduce errors.