Comments in C Programming
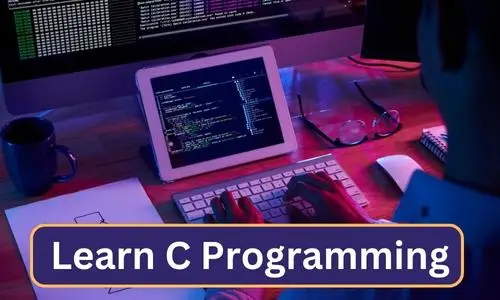
Page Index
Comments In C programming language are used to add notes or explanations to the code that are not interpreted by the compiler. Comments are meant to be read by other programmers, rather than by the computer.
There are two types of comments in C:
- Single line comments: These comments begin with two forward slashes (//) and continue until the end of the line. Single-line comments are typically used to provide brief explanations or to temporarily disable a line of code. For example:
// This is a single-line comment in C
- Multi line comments: These comments begin with /* and end with */. Multi-line comments are used for longer explanations or to comment out multiple lines of code. For example:
/* This is a multi-line comment in C
It can span multiple lines
and provide more detailed explanations */
Comments are useful for providing context and improving the readability of code. Good comments can help other programmers understand what the code does, how it works, and why certain decisions were made. It is important to use comments sparingly and to keep them up-to-date, as outdated or incorrect comments can be misleading.
Code to Explain the Comments in C Programming
/*
This is a multi-line comment in C.
It can span multiple lines and is useful for longer explanations or for temporarily disabling multiple lines of code.
In this example, the comment explains that the program prints "Hello, world!" to the console.
*/
#include <stdio.h>int main() {
// Print "Hello, world!" to the console
printf("Hello, world!\\n");
return 0;
}
In this example, the multi-line comment spans four lines and explains the purpose of the program: to print “Hello, world!” to the console. The comment is enclosed in /* and / characters, and each line of the comment starts with an asterisk (). The comment provides additional context and makes the code easier to understand for other programmers who might be reading the code.
It’s important to note that comments are not executed by the computer and do not affect the behaviour of the program. They are only meant to be read and understood by other programmers.