C Programming Block
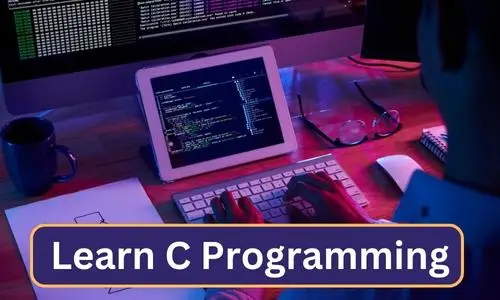
Page Index
The C programming language is a general-purpose, procedural programming language that was developed in the early 1970s. It is widely used for system programming, as well as developing applications and embedded systems. The building blocks of C programming are the fundamental concepts and components that make up the language. Here are some of the key building blocks:
- Variables: Variables are used to store data values in a program. They are named memory locations that can hold different types of data, such as integers, characters, or floating-point numbers. In C, you need to declare the type of a variable before using it, such as int, char, or float.
- Data types:Â Data types define the type and size of the data that a variable can hold. C supports several basic data types, including:
- int: integer numbers
- float: floating-point numbers
- double: double-precision floating-point numbers
- char: single characters
- Constants and Literals: Constants are fixed values that do not change during the execution of a program. Literals are the actual values assigned to variables or constants. There are several types of constants, such as integer, floating-point, and character constants.
- Operators:Â Operators are symbols that perform operations on operands (variables or values). C has several types of operators, such as:
- Arithmetic operators: +, -, *, /, %
- Relational operators: >, <, >=, <=, ==, !=
- Logical operators: &&, ||, !
- Bitwise operators: &, |, ^, ~, <<, >>
- Control Structures:Â Control structures determine the flow of execution in a program. They include:
- Conditional statements: if, if-else, switch
- Loop statements: for, while, do-while
- Branching statements: break, continue, goto
- Functions: Functions are blocks of code that perform a specific task. They can be called by other parts of the program, and can return a value or perform an action. Functions can be user-defined or built-in, such as printf() and scanf().
- Arrays: Arrays are a collection of elements of the same data type, stored in contiguous memory locations. They are used to store multiple values of the same type under a single name.
- Pointers: Pointers are variables that store the memory address of another variable. They are used for dynamic memory allocation, managing arrays, and working with functions.
- Structures: Structures are user-defined data types that group related variables of different data types under a single name. They are useful for organizing complex data.
- File I/O: File input/output (I/O) allows a program to read from and write to files. This is accomplished using built-in functions such as fopen(), fclose(), fscanf(), and fprintf().
- Preprocessor Directives: Preprocessor directives are commands that are processed before the compilation of a program. They include:
- #include: for including header files
- #define: for defining constants and macros
- #if, #else, #elif, #endif: for conditional compilation
- Standard Library: The C standard library is a collection of functions, macros, and header files that provide commonly used functionality for tasks like input/output, memory management, and string manipulation. Some commonly used header files include stdio.h, stdlib.h, string.h, and math.h
- Dynamic Memory Allocation: Dynamic memory allocation allows the programmer to allocate and deallocate memory during runtime. This is useful when the size of an array or the amount of memory needed cannot be determined at compile time. C provides functions for dynamic memory allocation, such as malloc(), calloc(), realloc(), and free().
- Command Line Arguments: Command line arguments are inputs passed to a program when it is executed from the command line. They allow a user to provide input data or options to control the program’s behavior. In C, command line arguments are accessed using the ‘argc’ (argument count) and ‘argv’ (argument vector) parameters in the main function.
- Typecasting: Typecasting is the process of converting one data type to another. This can be done implicitly by the compiler, or explicitly by the programmer using a typecast operator. For example, converting a float to an int or an int to a char.
- Enumerations: Enumerations are user-defined data types that consist of a set of named integer constants. They are used to make code more readable and maintainable by assigning meaningful names to integer values.
- Typedef: The typedef keyword is used to create aliases for existing data types. This can make code more readable by providing a more meaningful name for a data type or by simplifying complex type declarations.
- Unions: Unions are similar to structures but allow you to store different data types in the same memory location. They are used when you want to store different types of data in a single variable, but only one type at a time.
- Error handling: Error handling is an important aspect of programming to ensure that a program can handle unexpected situations and errors gracefully. In C, error handling can be achieved using return values, errno, and the perror() function.
- Recursion: Recursion is a programming technique where a function calls itself, either directly or indirectly, to solve a problem. It can be a powerful way to solve problems with a simple and elegant solution, but it can also lead to issues like stack overflow if not implemented carefully.
- Multi-file programs: Larger programs can be split into multiple source files to improve organization and maintainability. These files are combined during the compilation process. Functions and variables that need to be accessed across multiple source files can be declared with the ‘extern’ keyword.
- Modular programming: Modular programming is the practice of dividing a program into smaller, self-contained units or modules. Each module should have a well-defined purpose and interface, and the modules should be combined to create the complete program. This approach improves maintainability, reusability, and readability of the code.