Learn C Programming
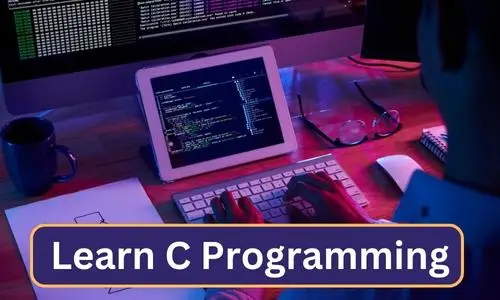
Page Index
What is C Programming?
C Programming language is a high-level programming language that was developed in the early 1970s by Dennis Ritchie at Bell Labs. It is a general-purpose programming language that is used for developing operating systems, compilers, embedded systems, and various other applications.
C programming language is known for its efficiency, portability, and low-level memory manipulation capabilities. It provides features such as structured programming, data types, and syntax that allow programmers to write efficient and concise code.
C has influenced many other programming languages and is still widely used today. It has a large community of developers and a wealth of resources available, making it an excellent language to learn for anyone interested in programming.
Why learn C Programming?
There are several reasons why one might want to learn C programming:
- Widely used: C is one of the oldest and most widely used programming languages in the world. It is used to develop operating systems, compilers, embedded systems, and many other applications.
- Efficiency: C is a low-level language that provides access to memory and hardware, which makes it highly efficient. It allows programmers to write code that executes quickly and uses minimal system resources.
- Portability: C code can be compiled and executed on different platforms, making it highly portable. This means that programs written in C can be run on different operating systems and hardware without modification.
- Foundation for other languages: Many popular programming languages, such as C++, Java, and Python, are based on C. Learning C provides a strong foundation for learning other languages.
- Career opportunities: C is a highly sought-after skill in the job market. Many industries, including software development, embedded systems, and game development, require C programming skills.
Facts about C Programming
- Origin: C programming language was developed by Dennis Ritchie at Bell Labs in the early 1970s as an evolution of the B programming language.
- Influential: C programming language has had a huge impact on the development of many other programming languages. Many popular programming languages, including C++, Java, and Python, are based on C.
- Efficiency: C programming language is known for its efficiency and low-level memory manipulation capabilities. It allows programmers to write code that executes quickly and uses minimal system resources.
- Portability: C code can be compiled and executed on different platforms, making it highly portable. This means that programs written in C can be run on different operating systems and hardware without modification.
- Syntax: C programming language has a concise and structured syntax that allows programmers to write efficient and readable code.
- Standardization: C programming language is standardized by the International Organization for Standardization (ISO) and the American National Standards Institute (ANSI).
- Embedded systems: C programming language is widely used for developing embedded systems, which are computer systems that are embedded in other devices such as cars, appliances, and medical equipment.
- Open-source: There are many open-source C compilers and development tools available, which makes it accessible to a wide range of users.
Applications of C Programming
C programming language is used in a variety of applications, including:
- Operating systems: C programming language is commonly used for developing operating systems, such as Unix and Linux.
- Compilers: C programming language is used for developing compilers, which are programs that translate source code into executable code.
- Embedded systems: C programming language is widely used for developing embedded systems, which are computer systems that are embedded in other devices such as cars, appliances, and medical equipment.
- Networking: C programming language is used for developing networking applications such as network drivers, firewalls, and routers.
- Game development: C programming language is commonly used for developing video games because of its speed and low-level memory manipulation capabilities.
- Database systems: C programming language is used for developing database systems, which are software systems that manage large amounts of data.
- Scientific computing: C programming language is used for scientific computing applications, such as simulations, modeling, and data analysis.
- Graphics programming: C programming language is used for developing graphics applications, such as computer-aided design (CAD) software and image processing applications.
Where to Write the C Program?
You can write a C program in any text editor or integrated development environment (IDE) that supports C programming language. Some commonly used text editors and IDEs for C programming include:
- Visual Studio Code
- Eclipse
- Code::Blocks
- Sublime Text
- Atom
- Vim
- Emacs
Once you have written your C program in a text editor or IDE, you can save the file with a .c extension, such as hello.c
, and then compile and execute the program using a C compiler, such as gcc, in a terminal or command prompt.
Basic C Program Example
#include <stdio.h>
int main() {
printf("Hello, world!\n");
return 0;
}
The output of the Program :
The output of the above program, which prints the message “Hello, world!” to the console, will be:
Hello, world!
This output will be displayed in the terminal window after the program is compiled and executed using a C compiler, such as gcc.
Explanation of the Code :
- This code uses the C programming language to print the message “Hello, world!” to the console.
- The first line
#include <stdio.h>
is a preprocessor directive that tells the compiler to include thestdio.h
header file, which provides input and output functions for C programs. - The second line
int main()
is the main function of the program, where the execution of the code starts. - Inside the main function, the
printf()
function is called with the string"Hello, world!\\n"
as an argument. This function is used to print formatted output to the console, and the\\n
character sequence at the end of the string is used to add a newline character to the output. - Finally, the
return 0;
statement is used to indicate the successful completion of the program, with a return value of zero.
How to Compile and Execute the C Program
To compile and execute a C program, follow these steps:
- Open a text editor and write your C program code. Save the file with a .c extension, such as
hello.c
. - Open a command prompt or terminal window on your computer.
- Navigate to the directory where your C program file is saved using the
cd
command. For example, if your program file is saved in the “Documents” folder, you would use the commandcd Documents
. - Compile your C program using a C compiler, such as gcc, by typing the command
gcc -o output_file_name input_file_name.c
in the terminal. For example, if your program file is namedhello.c
, you would use the commandgcc -o hello hello.c
. This will create an executable file calledhello
. - To execute the program, type
./output_file_name
in the terminal. For example, if your output file name ishello
, you would use the command./hello
. This will run your C program, and the output should be displayed in the terminal.
Career Opportunities after Learning C Programming
There are many career opportunities available for individuals who have learned C programming.
- Software Developer: C is used to design operating systems, network drivers, compilers, and embedded systems. Software developers can hire C programmers.
- System Programmer: C is used for system-level programming, such as writing drivers, connecting with hardware, and working on operating system kernels. System programmers can work for operating system, embedded system, and hardware device developers.
- Game Developer: C is used to design game engines, graphics, and AI in games. C programmers can work in game development studios.
- Firmware Engineer: C is used to write firmware for embedded systems like microcontrollers. Firmware engineers work for hardware and embedded system firms.
- Security Researcher: C is used to write encryption methods and secure communication protocols. As a security researcher, you can work for cybersecurity or security software firms.
- Compiler Developer: C is used to construct compilers, which transform code from one computer language to another. You can work on open source compiler projects or for programming language firms as a compiler developer.
- Technical Writer: Writing documentation for software applications, APIs, and other technical items requires C programming skills. Software firms, tech startups, and freelance technical writers can collaborate together.
- Data Analyst: C can handle massive data sets and construct data processing algorithms, making it useful for data analysis. Data analysts might work in banking, healthcare, and technology industries that handle enormous volumes of data.
- Robotics Engineer: C programming is used to programme robots to do certain jobs or interact with their environment. Robotics engineers work for robot manufacturers or research universities.
- IOT Developer: C programming is beneficial for designing software for the Internet of Things (IoT), including embedded systems and devices that communicate. As an IoT developer, you can work with firms that make IoT products or startups that focus on IoT technologies.
Programming languages Developed Before C Programming
Language | Year Developed | Primary Use |
---|---|---|
Fortran | 1957 | Scientific and engineering applications |
Lisp | 1958 | List processing and artificial intelligence |
Algol | 1958 | Scientific computing |
COBOL | 1959 | Business applications |
FORTRAN II | 1959 | Scientific and engineering applications |
BASIC | 1964 | Beginner-friendly language |
PL/I | 1964 | General-purpose programming for scientific and business applications |
Simula | 1967 | Object-oriented programming for simulating complex systems |
SNOBOL | 1967 | Text processing and pattern matching |
ALGOL 68 | 1968 | Algorithmic language for scientific computing |
Features of C Programming
C programming language has several features that make it a powerful and versatile language for systems programming, including:
- Low-level programming: C provides low-level control over hardware and memory, allowing programmers to write efficient and optimized code.
- Portability: C code can be compiled on different platforms and architectures, making it a portable language for software development.
- Structured programming: C supports structured programming techniques, such as functions and control structures, which make code more organized and easier to maintain.
- Modularity: C supports modular programming, which allows code to be broken down into smaller, more manageable modules that can be reused in different applications.
- Pointer manipulation: C provides powerful pointer manipulation capabilities, which allow programmers to work with memory addresses directly.
- Efficient memory management: C allows programmers to manage memory directly, which can lead to more efficient use of system resources.
- Large standard library: C comes with a large standard library of functions and data types, which can be used to develop a wide range of software applications.
- Speed: C is a high-performance language that can execute code quickly, making it ideal for applications that require fast processing speeds, such as game development and scientific computing.
- Flexibility: C is a flexible language that can be used for a wide range of applications, from low-level systems programming to high-level application development.
- Open source: C is an open-source language, which means that it is freely available for anyone to use, modify, and distribute.
- Interoperability: C can be used to interface with other programming languages and libraries, allowing developers to leverage existing code and technologies in their applications.
- Standardization: C is a standardized language, with a well-defined syntax and semantics, making it easy for developers to write portable and maintainable code.
- Preprocessor: C includes a preprocessor that allows developers to perform text substitution and other operations before compilation, making it easier to write and maintain code.