C Program Structure
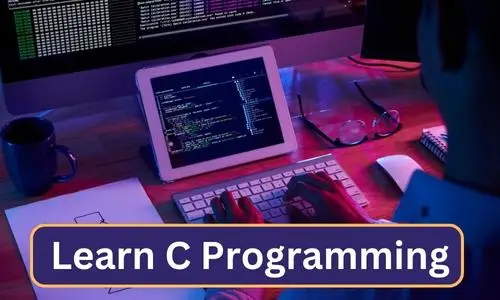
Page Index
A basic C program consists of the following parts:
1. Preprocessor Directives / Header Files : The preprocessor directives are instructions for the C preprocessor, which is a program that processes the source code before it is compiled. The most common preprocessor directive is #include
, which is used to include header files that provide function and variable declarations. For example, the following line of code includes the standard input/output header file. They are included at the beginning of the program using the #include
directive.
#include <stdio.h>
2. Main Function: The main function is the entry point of the program, and it is where the program execution begins. The main function has a return type of int
and takes no arguments by default. The main function is defined as follows:
int main() {
// statements
return 0;
}
The return 0 statement at the end of the main function indicates that the program has executed successfully.
3. Declarations: The declarations section contains variable and function declarations. It is located before the main function and is used to tell the compiler what types of variables and functions the program uses. For example, the following code declares a variable named x
int x;
4. Statements: The statements section contains the actual code that performs the tasks of the program. The statements are executed in sequence, one after the other. For example, the following code uses the printf
function to display a message to the user
printf("Hello, World!");
The printf function takes a string argument, which is enclosed in double quotes.
Putting it all together, a basic C program that displays “Hello, World!” on the screen would look like this:
#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
This program includes the stdio.h
header file, which provides the printf
function. The main function uses the printf
function to display the message “Hello, World!” to the user. The return 0
statement at the end of the main function indicates that the program has executed successfully.
Compile and Execute the Above C Program Step by Step :
To compile and execute the basic “Hello, World!” program in C, follow these steps:
- Open a text editor: Open a text editor of your choice, such as Notepad or Sublime Text.
- Write the program: Write the following code in the text editor:
#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
- Save the program: Save the program with a .c extension. For example, you can save it as
hello.c
. - Open a terminal: Open a terminal or command prompt on your computer.
- Navigate to the directory: Navigate to the directory where the program is saved using the
cd
command. - Compile the program: Compile the program using the following command:
gcc -o hello hello.c
This will compile the program and generate an executable file named hello
.
7. Execute the program: Execute the program by running the following command:
./hello
This will run the program and output “Hello, World!” to the terminal.